PHP Array Exercises : Filter a multi-dimensional array and get those items that will match with the specified value
52. Filter a Multi-Dimensional Array by Value
Write a PHP function to filter a multi-dimensional array. The function will return those items that will match with the specified value.
Sample Solution:
PHP Code:
<?php
// Function to filter a multi-dimensional array based on a specific value in a specified index
function array_filter_by_value($my_array, $index, $value)
{
// Check if the input is a non-empty array
if(is_array($my_array) && count($my_array) > 0)
{
// Loop through the keys of the input array
foreach(array_keys($my_array) as $key){
// Extract the value at the specified index for the current key
$temp[$key] = $my_array[$key][$index];
// Check if the extracted value matches the desired value
if ($temp[$key] == $value){
// If so, add the corresponding array to the new array
$new_array[$key] = $my_array[$key];
}
}
}
// Return the filtered array
return $new_array;
}
// Example usage
$colors = array(
0 => array('key1' => 'Red', 'key2' => 'Green', 'key3' => 'Black'),
1 => array('key1' => 'Yellow', 'key2' => 'White', 'key3' => 'Pink')
);
// Filter the array based on the value 'White' in the 'key2' index
$results = array_filter_by_value($colors, 'key2', 'White');
// Print the filtered results
print_r($results);
?>
Output:
Array ( [1] => Array ( [key1] => Yellow [key2] => White [key3] => Pink ) )
Flowchart:
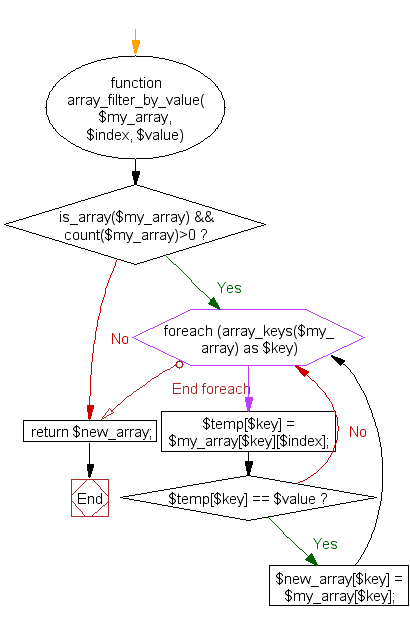
For more Practice: Solve these Related Problems:
- Write a PHP function to search through a multidimensional array and return all subarrays where a specified key equals a given value.
- Write a PHP script to filter a multidimensional array based on a specific value and then output the filtered result.
- Write a PHP program to use array_filter recursively on a multidimensional array to match a target value.
- Write a PHP script to implement a function that extracts subarrays from a multidimensional array when a specified key has a designated value.
Go to:
PREV : Filter Out Elements with Certain Key-Names.
NEXT : Delete Specific Value Using array_filter.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.