PHP Array Exercises : Filter array elements with certain key-names
PHP Array: Exercise-51 with Solution
Write a PHP program to filter out some array elements with certain key-names.
Sample Solution:
PHP Code:
<?php
// Original associative array
$first_array = array('c1' => 'Red', 'c2' => 'Green', 'c3' => 'White', 'c4' => 'Black');
// Array containing keys to be excluded from the original array
$second_array = array('c2', 'c4');
// Use array_flip to swap keys and values, then array_diff_key to get the difference in keys
$result = array_diff_key($first_array, array_flip($second_array));
// Print the resulting array
print_r($result);
?>
Output:
Array ( [c1] => Red [c3] => White )
Flowchart:
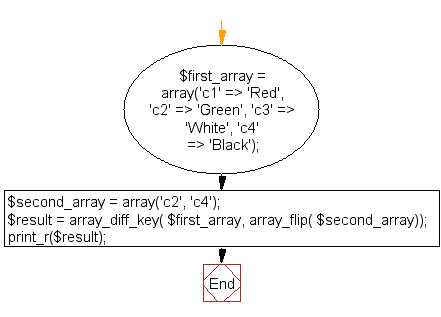
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP script to get the last value of an array without affecting the pointer.
Next: Write a PHP function to filter a multi-dimensional array. The function will return those items that will match with the specified value.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics