PHP Array Exercises : Delete a specific value from an array using array_filter() function
53. Delete Specific Value Using array_filter
Write a PHP script to delete a specific value from an array using array_filter() function.
Sample Solution:
PHP Code:
<?php
// Associative array representing colors
$colors = array('key1' => 'Red', 'key2' => 'Green', 'key3' => 'Black');
// Value to be excluded from the array
$given_value = 'Black';
// Print the original array
print_r($colors);
// Use array_filter with an anonymous function to create a new array excluding the given value
$filtered_array = array_filter($colors, function ($element) use ($given_value) {
return ($element != $given_value);
});
// Print the original array (Note: This line contains a typo, it should be $filtered_array instead of $new_filtered_array)
print_r($filtered_array);
// Print the new array excluding the given value
print_r($filtered_array);
?>
Output:
Array ( [key1] => Red [key2] => Green [key3] => Black ) PHP Notice: Undefined variable: filtered_array in /home/stu dents/2f6e9db0-f423-11e6-a8c0-b738b9ff32f9.php on line 7 Array ( [key1] => Red [key2] => Green )
Flowchart:
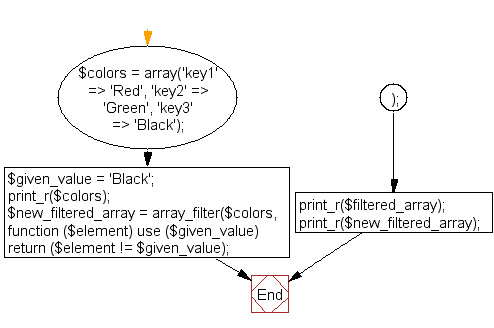
For more Practice: Solve these Related Problems:
- Write a PHP script to remove a given value from an array by filtering using array_filter and an anonymous function.
- Write a PHP function to delete all occurrences of a specific number from an array and then display the new array.
- Write a PHP program to filter an array for a specified value and output the array only with values not equal to that target.
- Write a PHP script to remove a string value from an array using array_filter while preserving the original keys.
Go to:
PREV : Filter a Multi-Dimensional Array by Value.
NEXT : Remove All White Spaces in Array.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.