PHP Class Exercises : Calculator class which will accept two values as arguments
PHP class: Exercise-6 with Solution
Write a PHP calculator class which will accept two values as arguments, then add them, subtract them, multiply them together, or divide them on request.
For example :
$mycalc = new MyCalculator( 12, 6);
echo $mycalc- > add(); // Displays 18
echo $mycalc- > multiply(); // Displays 72
Sample Solution:
PHP Code:
<?php
// Define a class named MyCalculator
class MyCalculator {
// Private properties to hold the first and second values
private $_fval, $_sval;
// Constructor to initialize the object with two values
public function __construct($fval, $sval) {
$this->_fval = $fval; // Assign the first value to the property _fval
$this->_sval = $sval; // Assign the second value to the property _sval
}
// Method to add the two values
public function add() {
return $this->_fval + $this->_sval; // Return the sum of _fval and _sval
}
// Method to subtract the second value from the first value
public function subtract() {
return $this->_fval - $this->_sval; // Return the difference of _fval and _sval
}
// Method to multiply the two values
public function multiply() {
return $this->_fval * $this->_sval; // Return the product of _fval and _sval
}
// Method to divide the first value by the second value
public function divide() {
return $this->_fval / $this->_sval; // Return the quotient of _fval and _sval
}
}
// Create an instance of the MyCalculator class with initial values 12 and 6
$mycalc = new MyCalculator(12, 6);
// Output the result of addition
echo $mycalc->add() . "\n"; // Displays 18
// Output the result of multiplication
echo $mycalc->multiply() . "\n"; // Displays 72
// Output the result of subtraction
echo $mycalc->subtract() . "\n"; // Displays 6
// Output the result of division
echo $mycalc->divide() . "\n"; // Displays 2
?>
Output:
18 72 6 2
Explanation:
In the exercise above,
- class MyCalculator { ... }: Defines a class named "MyCalculator" to encapsulate a set of calculator operations.
- private $_fval, $_sval;: Declares two private properties 'fval' and 'sval' to store the first and second values respectively.
- public function __construct($fval, $sval) { ... }: Constructor method that initializes the object with two values.
- public function add() { ... }: Method to add the two values.
- public function subtract() { ... }: Method to subtract the second value from the first value.
- public function multiply() { ... }: Method to multiply the two values.
- public function divide() { ... }: Method to divide the first value by the second value.
- $mycalc = new MyCalculator(12, 6);: Creates a new instance of the "MyCalculator" class with initial values 12 and 6.
- echo $mycalc->add() . "\n";: Invokes the "add()" method of the "$mycalc" object and displays the result.
- Similar invocations are made for the "multiply()", "subtract()", and "divide()" methods.
Flowchart :
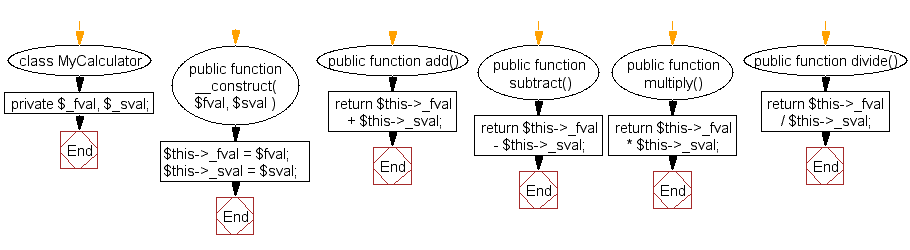
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Calculate the difference between two dates using PHP OOP approach.
Next: Write a PHP script to a convert string to Date and DateTime.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/php-exercises/php-class-exercise-6.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics