PHP Class Exercises : Calculate the difference between two dates
Calculate the difference between two dates using PHP OOP approach.
Sample Dates : 1981-11-03, 2013-09-04
Sample Salution:
PHP Code:
<?php
// Create a new DateTime object representing the start date "1981-11-03"
$sdate = new DateTime("1981-11-03");
// Create a new DateTime object representing the end date "2013-09-04"
$edate = new DateTime("2013-09-04");
// Calculate the interval between the two dates
$interval = $sdate->diff($edate);
// Output the difference between the dates in years, months, and days
echo "Difference : " . $interval->y . " years, " . $interval->m . " months, " . $interval->d . " days ";
?>
Output:
Difference : 31 years, 10 months, 1 days
Explanation:
In the exercise above,
- $sdate = new DateTime("1981-11-03");: This line creates a new DateTime object named '$sdate' representing the start date "1981-11-03".
- $edate = new DateTime("2013-09-04");: This line creates a new DateTime object named '$edate' representing the end date "2013-09-04".
- $interval = $sdate->diff($edate);: This line calculates the difference between the start date '$sdate' and the end date '$edate' using the "diff()" method, and stores the result in the variable '$interval'.
- echo "Difference : " . $interval->y . " years, " . $interval->m . " months, " . $interval->d . " days ";: This line outputs the difference between the two dates in years, months, and days by accessing the properties $interval->y (years), $interval->m (months), and $interval->d (days) of the "$interval" object.
Flowchart :
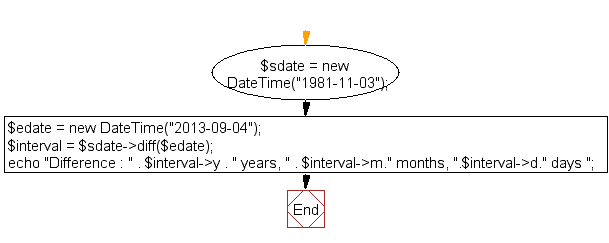
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP class that sorts an ordered integer array with the help of sort() function.
Next: Write a PHP calculator class which will accept two values as arguments, then add them, subtract them, multiply them together, or divide them on request.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.