PHP Class Exercises : Sort an ordered integer array with the help of sort() function
PHP class: Exercise-4 with Solution
Write a PHP class that sorts an ordered integer array with the help of sort() function.
Sample array : array(11, -2, 4, 35, 0, 8, -9)
Sample Solution:
PHP Code:
<?php
// Define a class named array_sort
class array_sort
{
// Declare a protected property named $_asort to store the array
protected $_asort;
// Define the constructor method which accepts an array $asort
public function __construct(array $asort)
{
// Assign the provided array to the protected property $_asort
$this->_asort = $asort;
}
// Define a method named alhsort to sort the array in ascending order
public function alhsort()
{
// Create a copy of the original array
$sorted = $this->_asort;
// Sort the copied array in ascending order
sort($sorted);
// Return the sorted array
return $sorted;
}
}
// Create an instance of the array_sort class with an array argument
$sortarray = new array_sort(array(11, -2, 4, 35, 0, 8, -9));
// Call the alhsort method of the $sortarray object and print the sorted array
print_r($sortarray->alhsort())."\n";
?>
Output:
Array ( [0] => -9 [1] => -2 [2] => 0 [3] => 4 [4] => 8 [5] => 11 [6] => 35 )
Explanation:
In the exercise above,
- class array_sort {: This line defines a class named "array_sort".
- protected $_asort;: This line declares a protected property named '$_asort' to store the array.
- public function __construct(array $asort) {: This line defines the constructor method of the class which accepts an array '$asort' as a parameter.
- $this->_asort = $asort;: This line assigns the provided array '$asort' to the protected property '$_asort'.
- public function alhsort() {: This line defines a public method named "alhsort()" which sorts the array stored in '$_asort' in ascending order.
- $sorted = $this->_asort;: This line creates a copy of the original array stored in '$_asort'.
- sort($sorted);: This line sorts the copied array '$sorted' in ascending order using the sort function.
- return $sorted;: This line returns the sorted array.
- $sortarray = new array_sort(array(11, -2, 4, 35, 0, 8, -9));: This line creates a new instance of the array_sort class with the provided array as an argument.
- print_r($sortarray->alhsort())."\n";: This line calls the "alhsort()" method of the "$sortarray" object to sort the array and prints the sorted array using "print_r".
Flowchart :
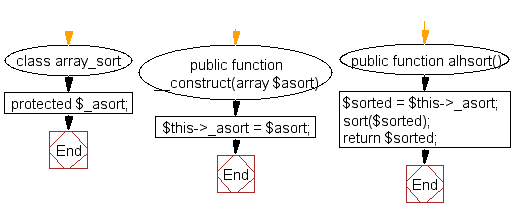
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP class that calculates the factorial of an integer.
Next: Calculate the difference between two dates using PHP OOP approach.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/php-exercises/php-class-exercise-4.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics