PHP Class Exercises : Calculate the factorial of an integer
PHP class: Exercise-3 with Solution
Write a PHP class that calculates the factorial of an integer.
Sample Solution:
PHP Code:
<?php
// Define a class named factorial_of_a_number
class factorial_of_a_number
{
// Declare a protected property named $_n
protected $_n;
// Define the constructor method which accepts a parameter $n
public function __construct($n)
{
// Check if the parameter $n is not an integer
if (!is_int($n)) {
// Throw an exception if $n is not an integer
throw new InvalidArgumentException('Not a number or missing argument');
}
// Assign the value of $n to the protected property $_n
$this->_n = $n;
}
// Define a method named result to calculate the factorial of the number
public function result()
{
// Initialize the factorial variable to 1
$factorial = 1;
// Iterate from 1 to the value of $_n
for ($i = 1; $i <= $this->_n; $i++) {
// Multiply the factorial by the current value of $i
$factorial *= $i;
}
// Return the calculated factorial
return $factorial;
}
}
// Create an instance of the factorial_of_a_number class with the argument 5
$newfactorial = new factorial_of_a_number(5);
// Call the result method of the $newfactorial object and output the result
echo $newfactorial->result();
?>
Output:
120
Explanation:
In the exercise above,
- class factorial_of_a_number {: This line defines a class named "factorial_of_a_number".
- protected $_n;: This line declares a protected property named '$_n' to store the number for which the factorial will be calculated.
- public function __construct($n) {: This line defines the constructor method of the class which accepts a parameter '$n'.
- if (!is_int($n)) {: This line checks if the provided argument '$n' is not an integer using the is_int function.
- throw new InvalidArgumentException('Not a number or missing argument');: If the argument '$n' is not an integer, an "InvalidArgumentException" is thrown with the specified message.
- $this->_n = $n;: This line assigns the value of the parameter '$n' to the protected property '$_n'.
- public function result() {: This line defines a public method named "result()" which calculates the factorial of the number stored in '$_n'.
- for ($i = 1; $i <= $this->_n; $i++) {: This line initializes a for loop to iterate from 1 to the value of '$_n' and calculates the factorial.
- return $factorial;: This line returns the calculated factorial value.
- $newfactorial = new factorial_of_a_number(5);: This line creates a new instance of the "factorial_of_a_number" class with the argument 5.
- echo $newfactorial->result();: This line calls the "result()" method of the "$newfactorial" object and outputs the calculated factorial value.
Flowchart :
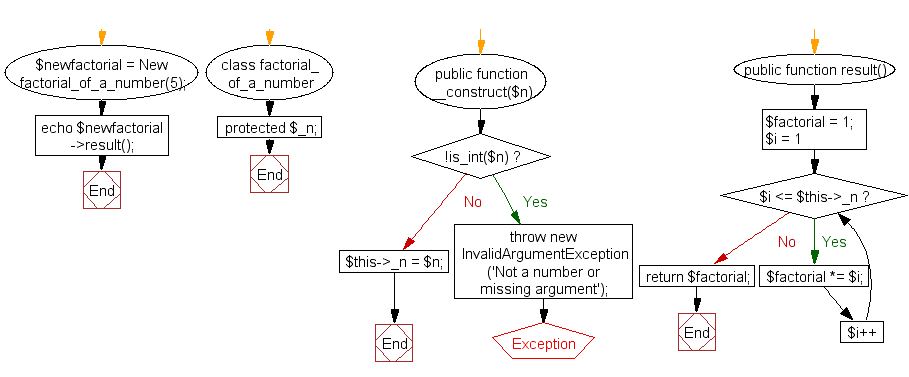
Go to:
PREV : Write a simple PHP class which displays an introductory message like "Hello All, I am Scott", where "Scott" is an argument value of the method within the class.
NEXT : Write a PHP class that sorts an ordered integer array with the help of sort() function.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.