PHP for loop Exercises : Iterating on two integers to get Fizz, Buzz and FizzBuzz
PHP for loop: Exercise-11 with Solution
Write a PHP program which iterates the integers from 1 to 100. For multiples of three print "Fizz" instead of the number and for the multiples of five print "Buzz". For numbers which are multiples of both three and five print "FizzBuzz".
Sample Solution:
PHP Code:
<?php
// Loop through numbers from 1 to 100
for ($i = 1; $i <= 100; $i++)
{
// Check if the number is divisible by both 3 and 5
if ($i % 3 == 0 && $i % 5 == 0)
{
// Print FizzBuzz if divisible by both 3 and 5
echo $i . " FizzBuzz" . "\n";
}
// Check if the number is divisible by 3 only
else if ($i % 3 == 0)
{
// Print Fizz if divisible by 3 only
echo $i . " Fizz" . "\n";
}
// Check if the number is divisible by 5 only
else if ($i % 5 == 0)
{
// Print Buzz if divisible by 5 only
echo $i . " Buzz" . "\n";
}
// If the number is not divisible by 3 or 5
else
{
// Print the number itself
echo $i . "\n";
}
}
?>
Output:
1 2 3 Fizz 4 5 Buzz 6 Fizz 7 8 9 Fizz 10 Buzz ...... 90 FizzBuzz 91 92 93 Fizz 94 95 Buzz 96 Fizz 97 98 99 Fizz 100 Buzz
Explanation:
In the exercise above,
- The code starts with a PHP opening tag <?php.
- It enters a "for" loop that iterates through numbers from 1 to 100 (for ($i = 1; $i <= 100; $i++)).
- Inside the loop, there are several conditional statements.
- It first checks if the current number is divisible by both 3 and 5 (if ($i % 3 0 && $i % 5 0)).
- If it is divisible by both, it echoes "FizzBuzz".
- If not, it checks if the number is divisible by 3 only (else if ($i % 3 == 0)).
- If it is divisible by 3 only, it echoes "Fizz".
- Next, it checks if the number is divisible by 5 only (else if ($i % 5 == 0)).
- If it is divisible by 5 only, it echoes "Buzz".
- If the number is not divisible by either 3 or 5, it simply echoes the number itself.
- After evaluating the conditions for each number in the loop, the code proceeds to the next iteration.
- Once all numbers from 1 to 100 have been processed, the loop ends, and the PHP code closes with ?>.
Flowchart:
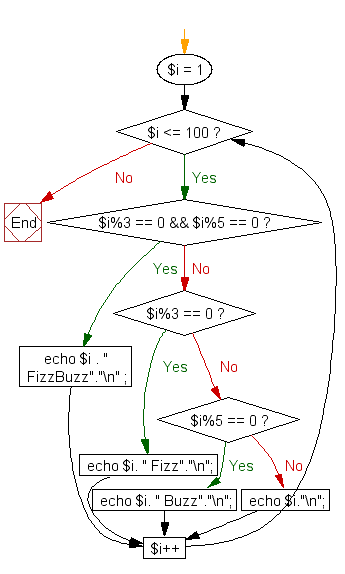
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP script that creates the specific table (use for loops).
Next: Write a PHP program to generate and display the first n lines of a Floyd triangle. (use n=5 and n=11 rows).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics