PHP for loop Exercises: Generate and display the first n lines of a Floyd triangle
PHP for loop: Exercise-12 with Solution
Write a PHP program to generate and display the first n lines of a Floyd triangle. (use n=5 and n=11 rows).
According to Wikipedia Floyd's triangle is a right-angled triangular array of natural numbers, used in computer science education. It is named after Robert Floyd. It is defined by filling the rows of the triangle with consecutive numbers, starting with a 1 in the top left corner:
Visual Presentation:
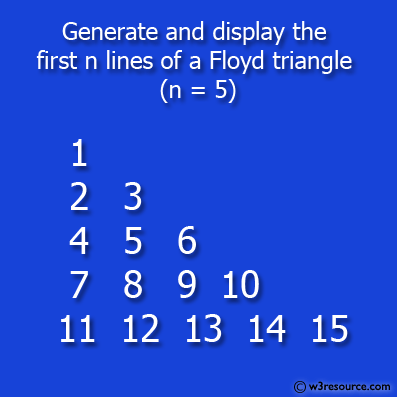
Sample Solution:
PHP Code:
<?php
// Initialize the value of n
$n = 5;
// Print the value of n
echo "n = " . $n . "\n";
// Initialize a counter variable
$count = 1;
// Outer loop for rows
for ($i = $n; $i > 0; $i--)
{
// Inner loop for columns
for ($j = $i; $j < $n + 1; $j++)
{
// Print the count with 4-character width
printf("%4s", $count);
// Increment the count
$count++;
}
// Move to the next line after each row
echo "\n";
}
?>
Output:
n = 5 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
Explanation:
In the exercise above,
- The code begins with a PHP opening tag <?php.
- It initializes the variable '$n' with the value 5, which represents the number of rows in the triangle pattern.
- It prints the value of 'n' using 'echo', along with a newline character (\n).
- The code initializes a counter variable '$count' to keep track of the numbers to be printed.
- It enters a nested loop structure:
- The outer loop (for ($i = $n; $i > 0; $i--)) controls the rows of the triangle, decrementing from 'n' to 1.
- Inside the outer loop, there's an inner loop (for ($j = $i; $j < $n + 1; $j++)) that controls the columns within each row. It starts from the current row number i and iterates up to 'n'.
- Within the inner loop, the code uses "printf()" to print the value of '$count', ensuring that each number is displayed with a width of 4 characters.
- After printing each number, the counter '$count' is incremented.
- After completing each row, the code moves to the next line by echoing a newline character (echo "\n";).
- Once all rows are printed, the PHP code ends with a closing PHP tag ?>.
Flowchart:
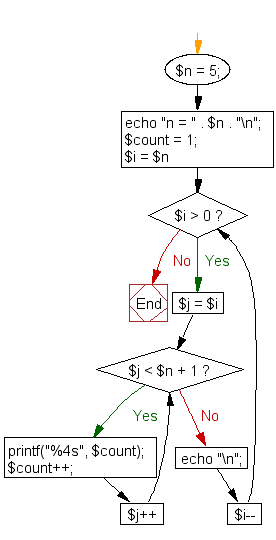
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program which iterates the integers from 1 to 100. For multiples of three print "Fizz" instead of the number and for the multiples of five print "Buzz". For numbers which are multiples of both three and five print "FizzBuzz".
Next: Write a PHP program to print alphabet pattern 'A'.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/php-exercises/php-for-loop-exercise-12.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics