Python: Find the Nth member of the sequence
Nth Member of Sequence
Write a Python program to create a sequence where the first four members of the sequence are equal to one. Each successive term of the sequence is equal to the sum of the four previous ones. Find the Nth member of the sequence.
Sample Solution:
Python Code:
# Define a recursive function 'new_seq' that generates a new sequence based on the sum
# of the four previous terms. The base cases are when 'n' is 1, 2, 3, or 4, where the
# function returns 1.
def new_seq(n):
if n == 1 or n == 2 or n == 3 or n == 4:
return 1
# Recursive step: The function returns the sum of the four previous terms.
return new_seq(n - 1) + new_seq(n - 2) + new_seq(n - 3) + new_seq(n - 4)
# Test the 'new_seq' function with different values of 'n' and print the results.
print(new_seq(5))
print(new_seq(6))
print(new_seq(7))
Sample Output:
4 7 13
Explanation:
This Python code defines a recursive function named "new_seq()" that generates a sequence based on the sum of the four previous terms. Here's a brief explanation:
- Function Definition:
- def new_seq(n):: Define a recursive function named "new_seq()" that takes an integer 'n' as input.
- Base Cases:
- if n 1 or n 2 or n 3 or n 4:: Check if 'n' is 1, 2, 3, or 4.
- return 1: If 'n' is one of these values, return 1 as a base case.
- Recursive Step:
- return new_seq(n - 1) + new_seq(n - 2) + new_seq(n - 3) + new_seq(n - 4): If 'n' is greater than 4, calculate the sum of the four previous terms using recursive calls to the "new_seq()" function.
- Function Testing:
- print(new_seq(5)): Test the function with 'n' equal to 5 and print the result.
- print(new_seq(6)): Test the function with 'n' equal to 6 and print the result.
- print(new_seq(7)): Test the function with 'n' equal to 7 and print the result.
Flowchart:
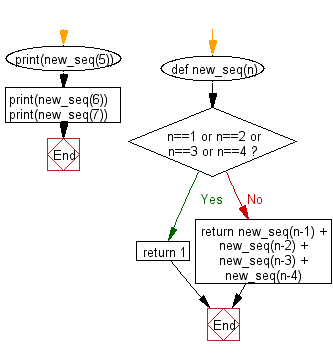
For more Practice: Solve these Related Problems:
- Write a Python program to compute the Nth member of a sequence where each term is the sum of the previous three terms (a modified Fibonacci sequence).
- Write a Python program to compute the Nth term of a sequence defined by a recurrence relation of variable order.
- Write a Python program to calculate the Nth term of a sequence where the sign alternates and each term is the sum of the previous two.
- Write a Python program to find the Nth term of a sequence where each term is computed as the product of the previous two terms plus a constant.
Go to:
Previous: Write a Python program to find the number of notes (Sample of notes: 10, 20, 50, 100, 200 and 500 ) against an given amount.
Next: Write a Python program that accept a positive number and subtract from this number the sum of its digits and so on. Continues this operation until the number is positive.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.