Python Cyber Security - Check if passwords in a list have been leaked in data breaches
Python Cyber Security: Exercise-6 with Solution
Write a Python program that reads a file containing a list of usernames and passwords, one pair per line (separatized by a comma). It checks each password to see if it has been leaked in a data breach. You can use the "Have I Been Pwned" API (https://haveibeenpwned.com/API/v3) to check if a password has been leaked.
Using Python "request" module you can store the result using the following command: response = requests.get(f"https://api.pwnedpasswords.com/range/{password_hash[:5]}")
Note: You can check if your email or phone is in a data breach from here https://haveibeenpwned.com/.
Content of passwords.txt
user1,Pas1$Ku1 user2,password user3,password123 user4,password123$ user5,Password6#(% user6,Germany#12
Sample Solution:
Python Code:
import requests
import hashlib
# Read the file containing usernames and passwords
with open('passwords.txt', 'r') as f:
for line in f:
# Split the line into username and password
username, password = line.strip().split(',')
# Hash the password using SHA-1 algorithm
password_hash = hashlib.sha1(password.encode('utf-8')).hexdigest().upper()
# Make a request to "Have I Been Pwned" API to check if the password has been leaked
response = requests.get(f"https://api.pwnedpasswords.com/range/{password_hash[:5]}")
# If the response status code is 200, it means the password has been leaked
if response.status_code == 200:
# Get the list of hashes of leaked passwords that start with the same 5 characters as the input password
hashes = [line.split(':') for line in response.text.splitlines()]
# Check if the hash of the input password matches any of the leaked password hashes
for h, count in hashes:
if password_hash[5:] == h:
print(f"Password for user {username} has been leaked {count} times.")
break
else:
print(f"Could not check password for user {username}.")
Sample Output:
Password for user user2 has been leaked 9659365 times. Password for user user3 has been leaked 251682 times. Password for user user4 has been leaked 514 times. Password for user user6 has been leaked 1 times
Flowchart:
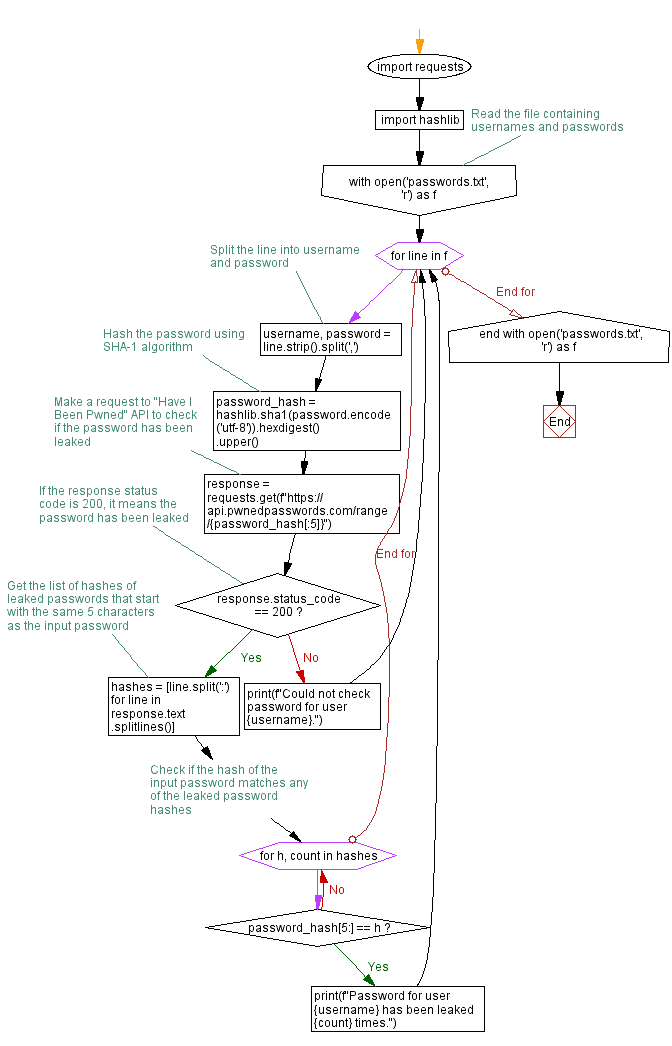
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Check and print valid passwords from a file.
Next: Check password strength and get suggestions.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics