Python Cyber Security - Check password strength and get suggestions
Python Cyber Security: Exercise-7 with Solution
Write a Python program that creates a password strength meter. The program should prompt the user to enter a password and check its strength based on criteria such as length, complexity, and randomness. Afterwards, the program should provide suggestions for improving the password's strength.
The highest strength password that meets the criteria specified in the program will receive a score of 5.
(e.g. at least 8 characters, contains both uppercase and lowercase letters, and at least one number and one special character).
Sample Solution:
Python Code:
import re
def check_password_strength(password):
score = 0
suggestions = []
# check length
if len(password) >= 8:
score += 1
else:
suggestions.append("Password should be at least 8 characters long")
# check for uppercase letter
if re.search(r"[A-Z]", password):
score += 1
else:
suggestions.append("Password should contain at least one uppercase letter")
# check for lowercase letter
if re.search(r"[a-z]", password):
score += 1
else:
suggestions.append("Password should contain at least one lowercase letter")
# check for numeric digit
if re.search(r"\d", password):
score += 1
else:
suggestions.append("Password should contain at least one numeric digit")
# check for special character
if re.search(r"[!@#$%^&*(),.?\":{}|<>]", password):
score += 1
else:
suggestions.append("Password should contain at least one special character (!@#$%^&*(),.?\":{}|<>)")
return score, suggestions
password = input("Input a password: ")
print(check_password_strength(password))
Sample Output:
Input a password: Akoiuw3^*(!@ (5, []) Input a password: ABH4@LLO (4, ['Password should contain at least one lowercase letter']) Input a password: Ohuyt1234 (4, ['Password should contain at least one special character (!@#$%^&*(),.?":{}|<>)']) Input a password: Aqwjuir (2, ['Password should be at least 8 characters long', 'Password should contain at least one numeric digit', 'Password should contain at least one special character (!@#$%^&*(),.?":{}|<>)'])
Flowchart:
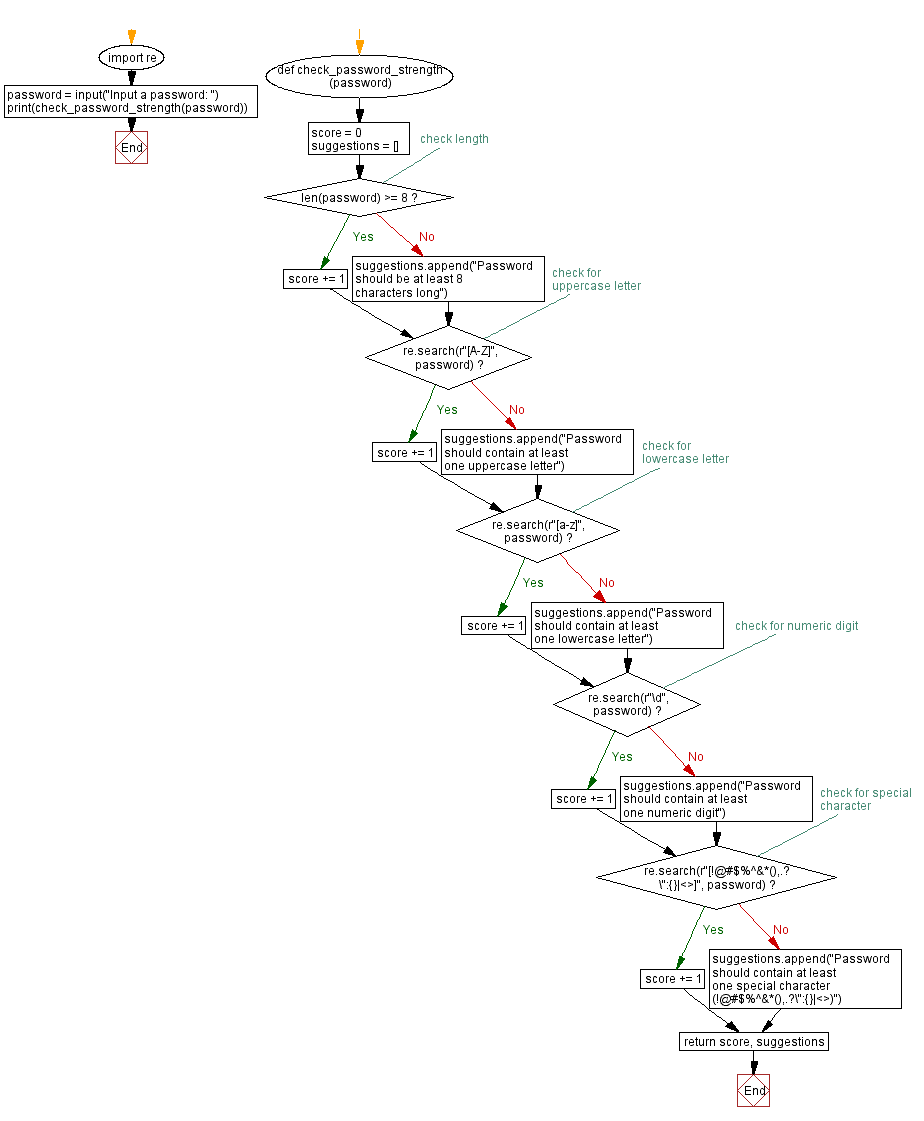
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Check if passwords in a list have been leaked in data breaches.
Next: Generate password from a dictionary file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics