Python Cyber Security - Generate password from a dictionary file
8. Random Word Password Generator
Write a Python program that generates a password using a random combination of words from a dictionary file.
Content of the dictionary file (dictionary.txt) :
Pas1$Ku1 password password123 password123$ Password6#(% Germany#12 USA12^$# England0#
Sample Solution:
Python Code:
import random
def generate_password():
# Open dictionary file
with open('dictionary.txt') as f:
words = f.read().splitlines()
# Randomly select four words
password = random.sample(words, 4)
# Combine words with hyphens
password = '-'.join(password)
return password
# Example usage
password = generate_password()
print(password)
Sample Output:
USA12^$#-password123-England0#-password123$ password-England0#-Password6#(%-Pas1$Ku1 USA12^$#-password123-password123$-password
Explanation:
In the above exercise, we first open a dictionary file and read all the words. Then, we use the random.sample() function to randomly select four words from the list of words. Finally, we combine the words with hyphens to form the final password. You can adjust the number of words included in the password by changing the argument to random.sample().
Flowchart:
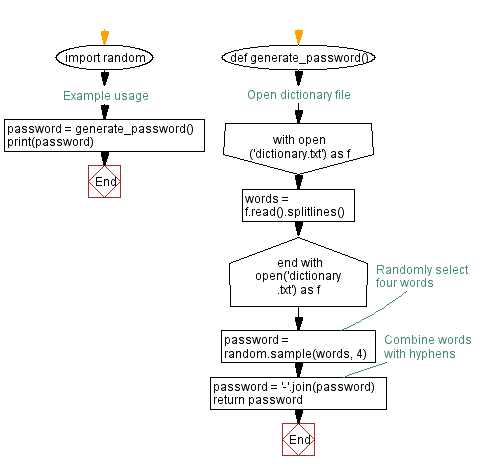
For more Practice: Solve these Related Problems:
- Write a Python program that reads words from a dictionary file, randomly selects three words, and concatenates them to form a password.
- Write a Python function that generates a passphrase by randomly choosing words from a given list and joins them with hyphens.
- Write a Python script to generate a password from random words, then apply common substitutions to enhance its complexity before printing.
- Write a Python program that allows the user to specify the number of words for a passphrase and then outputs a random passphrase from a dictionary file.
Go to:
Previous: Check password strength and get suggestions.
Next: Program for simulating dictionary attack on password.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.