NumPy: Divide each row by a vector element
Divide Each Row by Vector
Write a NumPy program to divide each row by a vector element.
Pictorial Presentation:
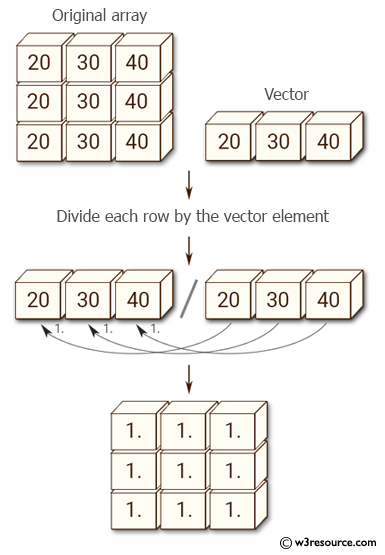
Sample Solution:
Python Code:
# Importing the NumPy library and aliasing it as 'np'
import numpy as np
# Creating a 2D NumPy array 'x' with repeated rows
x = np.array([[20, 20, 20], [30, 30, 30], [40, 40, 40]])
# Printing a message indicating the original array will be displayed
print("Original array:")
# Printing the original array 'x' with its elements
print(x)
# Creating a 1D NumPy array 'v' representing a vector
v = np.array([20, 30, 40])
# Printing a message indicating the vector will be displayed
print("Vector:")
# Printing the vector 'v' with its elements
print(v)
# Performing element-wise division of array 'x' by vector 'v' using broadcasting
# Dividing each row of 'x' by each element of 'v' using 'None' to introduce a new axis for vector 'v'
result = x / v[:, None]
# Printing the result of element-wise division
print(result)
Sample Output:
Original array: [[20 20 20] [30 30 30] [40 40 40]] Vector: [20 30 40] [[ 1. 1. 1.] [ 1. 1. 1.] [ 1. 1. 1.]]
Explanation:
In the above exercise –
x = np.array([[20,20,20],[30,30,30],[40,40,40]]): This line creates a 3x3 NumPy array 'x' with rows containing repeated values.
v = np.array([20,30,40]): This line creates a 1D NumPy array 'v' with three elements.
v[:, None]: Reshape the 1D array 'v' to a 2D array with shape (3, 1) by introducing a new axis using 'None' or 'np.newaxis'. The resulting array has dimensions that allow broadcasting with 'x'.
print(x / v[:, None]): Perform element-wise division of 'x' by the reshaped 'v' using broadcasting. Each row of 'x' is divided by the corresponding element of 'v'. The result is a 3x3 array with each row containing ones (since the elements of 'x' are multiples of the corresponding elements in 'v').
For more Practice: Solve these Related Problems:
- Write a NumPy program to divide each row of a 2D array by corresponding elements of a 1D vector using broadcasting.
- Create a function that normalizes each row of a matrix by a provided vector and verifies the output shape.
- Test row-wise division on an array and compare the results with manual element-wise division.
- Implement the division using np.divide and check for correct broadcasting behavior when dimensions differ.
Go to:
PREV : Check if Array is Empty
NEXT : Print All Array Values
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.