NumPy: Print all the values of an array
Print All Array Values
Write a NumPy program to print all array values.
Pictorial Presentation:
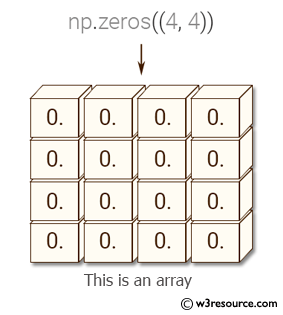
Sample Solution:
Python Code:
import numpy as np
import sys
# Setting the print options to display the entire array without truncation
# Use sys.maxsize or np.inf for untruncated representation
np.set_printoptions(threshold=sys.maxsize)
# Creating a 4x4 NumPy array 'x' filled with zeros
x = np.zeros((4, 4))
# Printing the array 'x'
print(x)
Sample Output:
[[ 0. 0. 0. 0.] [ 0. 0. 0. 0.] [ 0. 0. 0. 0.] [ 0. 0. 0. 0.]]
Explanation:
In the above code -
np.set_printoptions(threshold=np.nan): Set the print options in NumPy so that the entire array will be printed without truncation, regardless of its size. The 'threshold' parameter is set to 'np.nan' to ensure that all elements are displayed.
x = np.zeros((4, 4)): This line creates a 4x4 NumPy array 'x' filled with zeros.
print(x): Print the 4x4 array 'x', with all elements displayed due to the print options set earlier.
For more Practice: Solve these Related Problems:
- Write a NumPy program to print all elements of a multi-dimensional array by flattening it first.
- Create a function that iterates through every element of an array and prints each element on a new line.
- Test printing of large arrays to ensure that all elements are displayed without truncation.
- Compare the outputs of np.ndarray.flatten and np.ravel when printing every element of an array.
Go to:
PREV : Divide Each Row by Vector
NEXT : Raw Array to Binary String/Back
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.