Python Object-Oriented Programming: Person class with age calculation
Python OOP: Exercise-2 with Solution
Write a Python program to create a person class. Include attributes like name, country and date of birth. Implement a method to determine the person's age.
Sample Solution:
Python Code:
# Import the date class from the datetime module to work with dates
from datetime import date
# Define a class called Person to represent a person with a name, country, and date of birth
class Person:
# Initialize the Person object with a name, country, and date of birth
def __init__(self, name, country, date_of_birth):
self.name = name
self.country = country
self.date_of_birth = date_of_birth
# Calculate the age of the person based on their date of birth
def calculate_age(self):
today = date.today()
age = today.year - self.date_of_birth.year
if today < date(today.year, self.date_of_birth.month, self.date_of_birth.day):
age -= 1
return age
# Example usage
# Create three Person objects with different attributes
person1 = Person("Ferdi Odilia", "France", date(1962, 7, 12))
person2 = Person("Shweta Maddox", "Canada", date(1982, 10, 20))
person3 = Person("Elizaveta Tilman", "USA", date(2000, 1, 1))
# Access and print the attributes and calculated age for each person
print("Person 1:")
print("Name:", person1.name)
print("Country:", person1.country)
print("Date of Birth:", person1.date_of birth)
print("Age:", person1.calculate_age())
print("\nPerson 2:")
print("Name:", person2.name)
print("Country:", person2.country)
print("Date of Birth:", person2.date_of birth)
print("Age:", person2.calculate_age())
print("\nPerson 3:")
print("Name:", person3.name)
print("Country:", person3.country)
print("Date of Birth:", person3.date_of birth)
print("Age:", person3.calculate_age())
Sample Output:
Person 1: Name: Ferdi Odilia Country: France Date of Birth: 1962-07-12 Age: 60 Person 2: Name: Shweta Maddox Country: Canada Date of Birth: 1982-10-20 Age: 40 Person 3: Name: Elizaveta Tilman Country: USA Date of Birth: 2000-01-01 Age: 23
Explanation:
In the above exercise, we define a class called Person with an init method that initializes the attributes name, country, and date_of_birth.
The calculate_age method uses the date module from the datetime library to determine a person's age. This is based on their current date and their birth date. It calculates the difference between the current year and the birth year.
In the example usage section, we create three instances of the Person class, person1, person2 and person3, with different attribute values. Next, we access and print each person's attributes using dot notation (person.attribute_name), and determine their ages using calculate_age.
Flowchart:
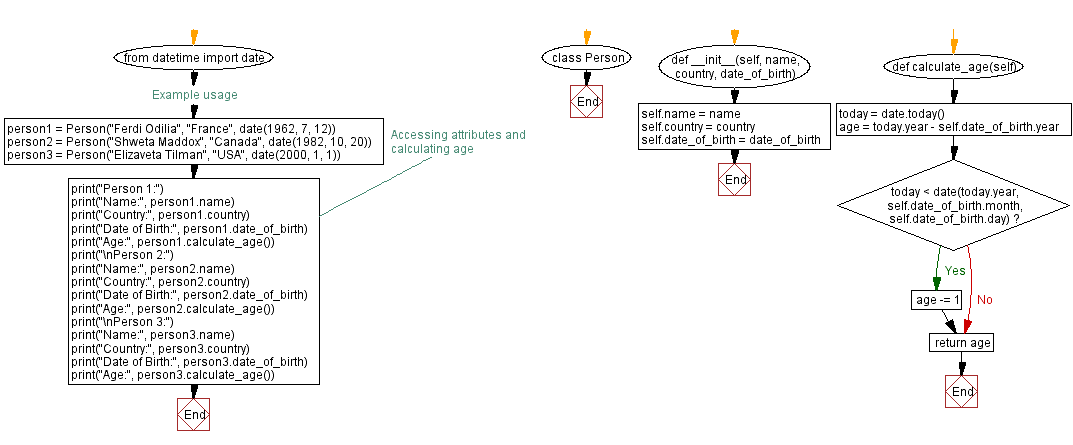
Python Code Editor:
Previous: Circle class with area and perimeter calculation.
Next: Calculator class for basic arithmetic.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics