Python Object-Oriented Programming: Circle class with area and perimeter calculation
1. Circle Class for Area and Perimeter
Write a Python program to create a class representing a Circle. Include methods to calculate its area and perimeter.
Sample Solution:
Python Code:
# Import the math module to access mathematical functions like pi
import math
# Define a class called Circle to represent a circle
class Circle:
# Initialize the Circle object with a given radius
def __init__(self, radius):
self.radius = radius
# Calculate and return the area of the circle using the formula: π * r^2
def calculate_circle_area(self):
return math.pi * self.radius**2
# Calculate and return the perimeter (circumference) of the circle using the formula: 2π * r
def calculate_circle_perimeter(self):
return 2 * math.pi * self.radius
# Example usage
# Prompt the user to input the radius of the circle and convert it to a floating-point number
radius = float(input("Input the radius of the circle: "))
# Create a Circle object with the provided radius
circle = Circle(radius)
# Calculate the area of the circle using the calculate_circle_area method
area = circle.calculate_circle_area()
# Calculate the perimeter of the circle using the calculate_circle_perimeter method
perimeter = circle.calculate_circle_perimeter()
# Display the calculated area and perimeter of the circle
print("Area of the circle:", area)
print("Perimeter of the circle:", perimeter)
Sample Output:
Input the radius of the circle: 4 Area of the circle: 50.26548245743669 Perimeter of the circle: 25.132741228718345
Input the radius of the circle: 5.7 Area of the circle: 102.07034531513239 Perimeter of the circle: 35.814156250923645
Explanation:
In the above exercise, we define a class called Circle with an init method that initializes the radius attribute. The class also includes two methods: calculate_circle_area and calculate_circle_perimeter.
The calculate_area method uses the formula pi*radius^2 to calculate the area of the circle. The calculate_circle_perimeter method uses the formula 2 * pi * radius to calculate the circle perimeter.
The program prompts the user to enter the circle radius in the example usage section. It then creates an instance of the Circle class using the given radius and calculates its area and perimeter. The calculated values are then printed.
Flowchart:
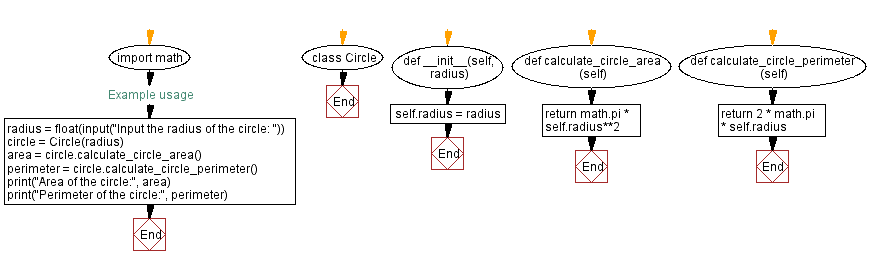
For more Practice: Solve these Related Problems:
- Write a Python class that calculates the area and circumference of a circle, and overloads the __add__ operator to combine circles by summing their areas.
- Write a Python class that validates the radius input and raises a custom exception for negative values while computing area and perimeter.
- Write a Python class for Circle that includes a class-level constant for π, and implement comparison operators to compare circles based on area.
- Write a Python class that computes the area and perimeter of a circle and provides a method to update the radius and recalculate the values.
Go to:
Previous: Python OOP Exercises Home.
Next: Person class with age calculation.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.