Python Object-Oriented Programming: Calculator class for basic arithmetic
3. Calculator Class for Basic Arithmetic Operations
Write a Python program to create a calculator class. Include methods for basic arithmetic operations.
Sample Solution:
Python Code:
# Define a class called Calculator to perform basic arithmetic operations
class Calculator:
# Define a method for addition that takes two arguments and returns their sum
def add(self, x, y):
return x + y
# Define a method for subtraction that takes two arguments and returns their difference
def subtract(self, x, y):
return x - y
# Define a method for multiplication that takes two arguments and returns their product
def multiply(self, x, y):
return x * y
# Define a method for division that takes two arguments and returns the result if the denominator is not zero,
# or an error message if the denominator is zero
def divide(self, x, y):
if y != 0:
return x / y
else:
return ("Cannot divide by zero.")
# Example usage
# Create an instance of the Calculator class
calculator = Calculator()
# Perform addition and print the result
result = calculator.add(7, 5)
print("7 + 5 =", result)
# Perform subtraction and print the result
result = calculator.subtract(34, 21)
print("34 - 21 =", result)
# Perform multiplication and print the result
result = calculator.multiply(54, 2)
print("54 * 2 =", result)
# Perform division and print the result
result = calculator.divide(144, 2)
print("144 / 2 =", result)
# Attempt to perform division by zero, which raises an error, and print the error message
result = calculator.divide(45, 0)
print("45 / 0 =", result)
Sample Output:
7 + 5 = 12 34 - 21 = 13 54 * 2 = 108 144 / 2 = 72.0 45 / 0 = Cannot divide by zero.
Explanation:
In the above exercise, we define a class called Calculator with methods add, subtract, multiply, and divide, representing basic arithmetic operations.
The add method performs addition of two numbers, subtract performs subtraction, multiply performs multiplication, and divide performs division. If the denominator is zero, the divide method returns an error message to avoid division by zero.
As an example, we create a Calculator instance, "calculator", and use it to perform various arithmetic operations.
Flowchart:
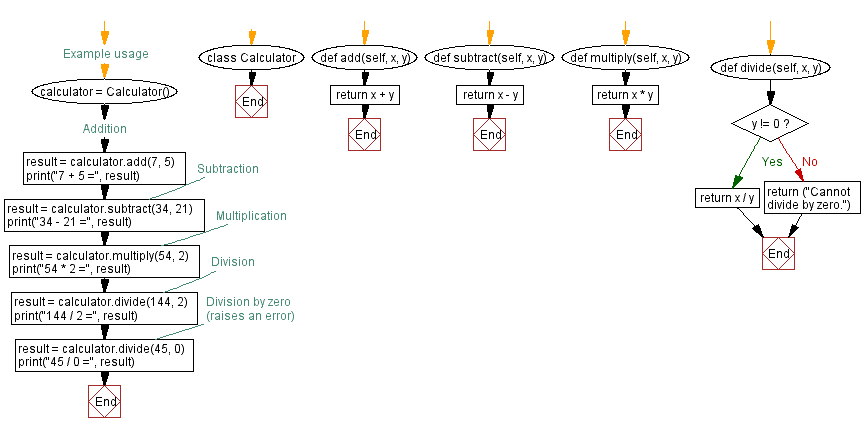
For more Practice: Solve these Related Problems:
- Write a Python class that implements basic arithmetic operations (add, subtract, multiply, divide) and handles division by zero gracefully.
- Write a Python class that supports operations on multiple operands using variable-length argument lists (*args) for addition and multiplication.
- Write a Python class that includes a memory feature for storing and recalling a value, in addition to standard arithmetic operations.
- Write a Python class that overloads arithmetic operators to allow direct use of +, -, *, and / between Calculator objects.
Go to:
Previous: Person class with age calculation.
Next: Write a Python program to create all possible strings by using 'a', 'e', 'i', 'o', 'u'. Use the characters exactly once.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.