Python Object-Oriented Programming: Stack class with push, pop, and display methods
9. Stack Data Structure Class with Display Method
Write a Python program to create a class representing a stack data structure. Include methods for pushing, popping and displaying elements.
Sample Solution:
Python Code:
# Define a class called Stack to implement a stack data structure
class Stack:
# Initialize the stack with an empty list to store items
def __init__(self):
self.items = []
# Push an item onto the stack
def push(self, item):
self.items.append(item)
# Pop (remove and return) an item from the stack if the stack is not empty
def pop(self):
if not self.is_empty():
return self.items.pop()
else:
raise IndexError("Cannot pop from an empty stack.")
# Check if the stack is empty
def is_empty(self):
return len(self.items) == 0
# Display the items in the stack
def display(self):
print("Stack items:", self.items)
# Example usage
# Create an instance of the Stack class
stack = Stack()
# Push items onto the stack
stack.push(10)
stack.push(20)
stack.push(30)
stack.push(40)
stack.push(50)
# Display the items in the stack
stack.display()
# Pop items from the stack and print the popped items
popped_item = stack.pop()
print("Popped item:", popped_item)
popped_item = stack.pop()
print("Popped item:", popped_item)
# Display the updated items in the stack
stack.display()
Sample Output:
Stack items: [10, 20, 30, 40, 50] Popped item: 50 Popped item: 40 Stack items: [10, 20, 30]
Explanation:
In this above exercise,
- We define a Stack class representing a stack data structure. It has an attribute called items, which is initially an empty list.
- The "push()" method takes an item as an argument and appends it to the items list, effectively adding it to the top of the stack.
- The "pop()" method removes and returns the topmost item from the stack. It checks if the stack is empty before popping an item. If the stack is empty, it raises an IndexError with an appropriate error message.
- The "is_empty()" method checks if the stack is empty by examining the length of the items list.
- The "display()" method simply prints the stack elements.
- In the example usage section, we create an instance of the Stack class called stack. We push and pop several items onto the stack using the "push()" and "pop()" methods. We then display the stack elements using the "display()" method.
Flowchart:
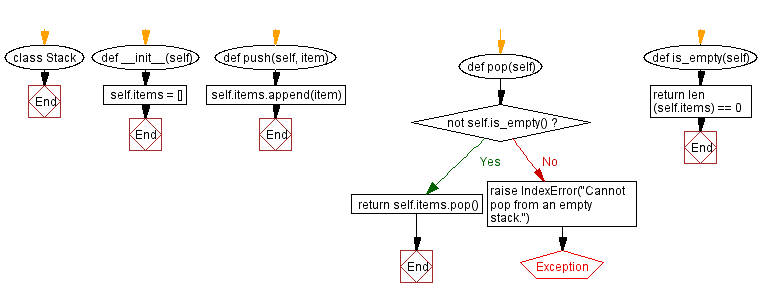
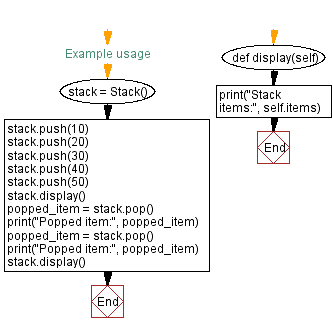
For more Practice: Solve these Related Problems:
- Write a Python class for a Stack that includes methods for push, pop, and a method to display all elements in the stack.
- Write a Python class for a Stack that provides a method to print the stack contents in reverse order.
- Write a Python class for a Stack that implements a peek() method and displays the top element without removing it.
- Write a Python class for a Stack that tracks the number of elements and provides a method to display both the elements and the count.
Go to:
Previous: Shopping cart class with item management and total calculation.
Next: Queue class with enqueue and dequeue methods.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.