Python Object-Oriented Programming: Shopping cart class with item management and total calculation
8. Shopping Cart Class
Write a Python program to create a class representing a shopping cart. Include methods for adding and removing items, and calculating the total price.
Sample Solution:
Python Code:
# Define a class called ShoppingCart to represent a shopping cart
class ShoppingCart:
# Initialize the shopping cart with an empty list of items
def __init__(self):
self.items = []
# Add an item with a name and quantity to the shopping cart
def add_item(self, item_name, qty):
item = (item_name, qty)
self.items.append(item)
# Remove an item with a specific name from the shopping cart
def remove_item(self, item_name):
for item in self.items:
if item[0] == item_name:
self.items.remove(item)
break
# Calculate and return the total quantity of items in the shopping cart
def calculate_total(self):
total = 0
for item in self.items:
total += item[1]
return total
# Example usage
# Create an instance of the ShoppingCart class
cart = ShoppingCart()
# Add items to the shopping cart
cart.add_item("Papaya", 100)
cart.add_item("Guava", 200)
cart.add_item("Orange", 150)
# Display the current items in the cart and calculate the total quantity
print("Current Items in Cart:")
for item in cart.items:
print(item[0], "-", item[1])
total_qty = cart.calculate_total()
print("Total Quantity:", total_qty)
# Remove an item from the cart, display the updated items, and recalculate the total quantity
cart.remove_item("Orange")
print("\nUpdated Items in Cart after removing Orange:")
for item in cart.items:
print(item[0], "-", item[1])
total_qty = cart.calculate_total()
print("Total Quantity:", total_qty)
Sample Output:
Current Items in Cart: Papaya - 100 Guava - 200 Orange - 150 Total Quantity: 450 Updated Items in Cart after removing Orange: Papaya - 100 Guava - 200 Total Quantity: 300
Explanation:
In this above exercise -
- We define a ShoppingCart class representing a shopping cart. It has an attribute called items, which is initially an empty list.
- The "add_item()" method takes an item name and quantity as arguments and adds them as a tuple to the items list. This adds the item to the cart.
- The "remove_item()" method removes the first occurrence of an item with the specified name from the items list. It iterates over the list and checks if the current item's name matches the specified name. If a match is found, it removes the item from the list and breaks out of the loop.
- The "calculate_total()" method calculates and returns the total quantity of all items in the cart. It iterates over the items list and accumulates the quantity of each item.
- In the example usage section, we create an instance of the ShoppingCart class called cart. We add several items to the cart using the "add_item()" method and display the current items in the cart.
- We calculate and display the total quantity of items using the "calculate_total()" method.
- The "remove_item()" method is used to remove an item from the cart and then display the updated items.
Flowchart:
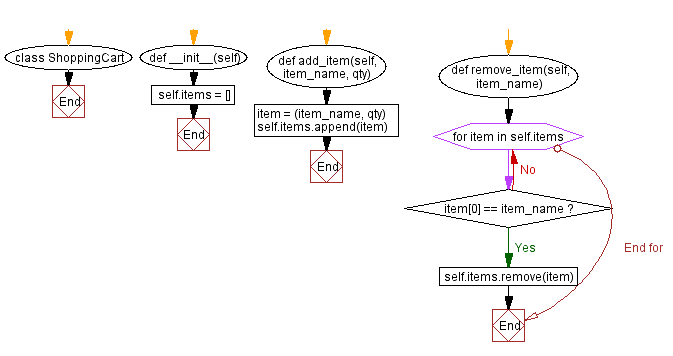
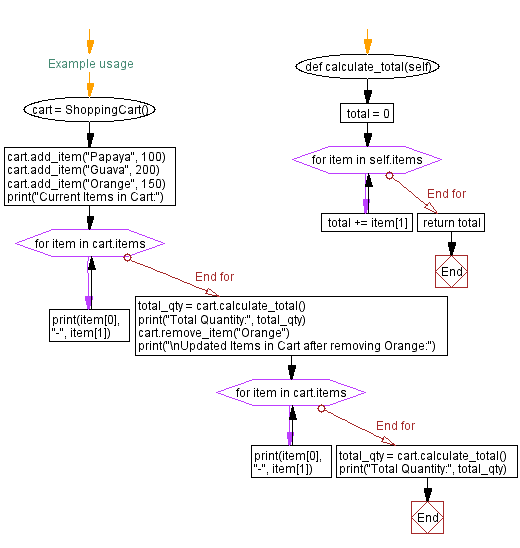
For more Practice: Solve these Related Problems:
- Write a Python class for a Shopping Cart that uses a dictionary to store items with their quantities and prices, and calculates the total cost.
- Write a Python class for a Shopping Cart that supports adding, removing, and updating item quantities and prints a detailed receipt.
- Write a Python class for a Shopping Cart that implements discount logic based on the total price and item count.
- Write a Python class for a Shopping Cart that logs each transaction and provides a method to review the transaction history.
Go to:
Previous: Linked list class with insertion, deletion, and display methods.
Next: Stack class with push, pop, and display methods.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.