Python Object-Oriented Programming: Queue class with enqueue and dequeue methods
Python OOP: Exercise-10 with Solution
Write a Python program to create a class representing a queue data structure. Include methods for enqueueing and dequeueing elements.
Sample Solution:
Python Code:
# Define a class called Queue to implement a queue data structure
class Queue:
# Initialize the queue with an empty list to store items
def __init__(self):
self.items = []
# Add (enqueue) an item to the end of the queue
def enqueue(self, item):
self.items.append(item)
# Remove and return (dequeue) an item from the front of the queue if the queue is not empty
def dequeue(self):
if not self.is_empty():
return self.items.pop(0)
else:
raise IndexError("Cannot dequeue from an empty queue.")
# Check if the queue is empty
def is_empty(self):
return len(self.items) == 0
# Example usage
# Create an instance of the Queue class
queue = Queue()
# Enqueue (add) items to the queue
queue.enqueue(10)
queue.enqueue(20)
queue.enqueue(30)
queue.enqueue(40)
queue.enqueue(50)
# Print the current items in the queue
print("Current Queue:", queue.items)
# Dequeue (remove) items from the front of the queue and print the dequeued items
dequeued_item = queue.dequeue()
print("Dequeued item:", dequeued_item)
dequeued_item = queue.dequeue()
print("Dequeued item:", dequeued_item)
# Print the updated items in the queue
print("Updated Queue:", queue.items)
Sample Output:
Current Queue: [10, 20, 30, 40, 50] Dequeued item: 10 Dequeued item: 20 Updated Queue: [30, 40, 50]
Explanation:
In this above exercise -
- We define a Queue class representing a queue data structure. It has an attribute called items, which is initially an empty list.
- The "enqueue()" method takes an item as an argument and appends it to the end of the items list. This adds it to the back of the queue.
- The "dequeue()" method removes and returns the item at the front of the queue. It checks if the queue is empty before dequeuing an item. If the queue is empty, it raises an IndexError with an appropriate error message.
- The "is_empty()" method checks if the queue is empty by examining the length of the items list.
- In the example usage section, we create an instance of the Queue class called queue. We enqueue several items into the queue using the "enqueue()" method.
- In addition, we demonstrate how to dequeue an item from the queue using the dequeue method.
Flowchart:
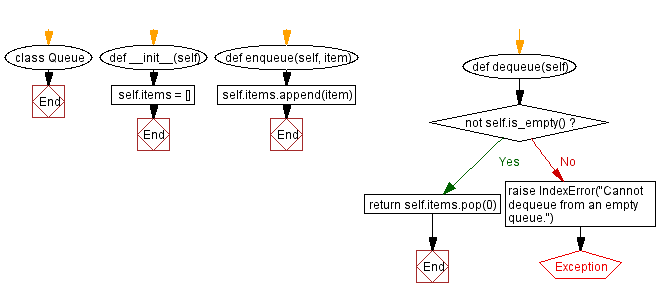
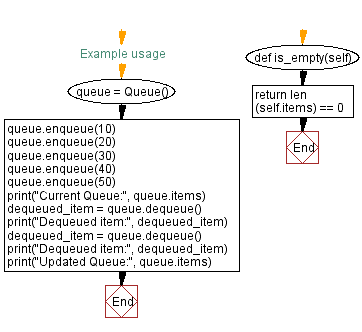
Python Code Editor:
Previous: Stack class with push, pop, and display methods.
Next: Bank class for customer account management.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics