Python Object-Oriented Programming: Bank class for customer account management
11. Bank Class for Managing Customer Accounts and Transactions
Write a Python program to create a class representing a bank. Include methods for managing customer accounts and transactions.
Sample Solution:
Python Code:
# Define a class called Bank to implement a simple banking system
class Bank:
# Initialize the bank with an empty dictionary to store customer accounts and balances
def __init__(self):
self.customers = {}
# Create a new account with a given account number and an optional initial balance (default to 0)
def create_account(self, account_number, initial_balance=0):
if account_number in self.customers:
print("Account number already exists.")
else:
self.customers[account_number] = initial_balance
print("Account created successfully.")
# Make a deposit to the account with the given account number
def make_deposit(self, account_number, amount):
if account_number in self.customers:
self.customers[account_number] += amount
print("Deposit successful.")
else:
print("Account number does not exist.")
# Make a withdrawal from the account with the given account number
def make_withdrawal(self, account_number, amount):
if account_number in self.customers:
if self.customers[account_number] >= amount:
self.customers[account_number] -= amount
print("Withdrawal successful.")
else:
print("Insufficient funds.")
else:
print("Account number does not exist.")
# Check and print the balance of the account with the given account number
def check_balance(self, account_number):
if account_number in self.customers:
balance = self.customers[account_number]
print(f"Account balance: {balance}")
else:
print("Account number does not exist.")
# Example usage
# Create an instance of the Bank class
bank = Bank()
# Create customer accounts and perform account operations
acno1= "SB-123"
damt1 = 1000
print("New a/c No.: ",acno1,"Deposit Amount:",damt1)
bank.create_account(acno1, damt1)
acno2= "SB-124"
damt2 = 1500
print("New a/c No.: ",acno2,"Deposit Amount:",damt2)
bank.create_account(acno2, damt2)
wamt1 = 600
print("\nDeposit Rs.",wamt1,"to A/c No.",acno1)
bank.make_deposit(acno1, wamt1)
wamt2 = 350
print("Withdraw Rs.",wamt2,"From A/c No.",acno2)
bank.make_withdrawal(acno2, wamt2)
print("A/c. No.",acno1)
bank.check_balance(acno1)
print("A/c. No.",acno2)
bank.check_balance(acno2)
wamt3 = 1200
print("Withdraw Rs.",wamt3,"From A/c No.",acno2)
bank.make_withdrawal(acno2, wamt3)
acno3 = "SB-134"
print("A/c. No.",acno3)
bank.check_balance(acno3) # Non-existent account number
Sample Output:
New a/c No.: SB-123 Deposit Amount: 1000 Account created successfully. New a/c No.: SB-124 Deposit Amount: 1500 Account created successfully. Deposit Rs. 600 to A/c No. SB-123 Deposit successful. Withdraw Rs. 350 From A/c No. SB-124 Withdrawal successful. A/c. No. SB-123 Account balance: 1600 A/c. No. SB-124 Account balance: 1150 Withdraw Rs. 1200 From A/c No. SB-124 Insufficient funds. A/c. No. SB-134 Account number does not exist.
Explanation:
In this above exercise -
We define a Bank class representing a bank. It has an attribute called customers, which is initially an empty dictionary.
The "create_account()" method takes an account number and an optional initial balance as arguments. It checks if the account number already exists in the customers' dictionary. If it does, it prints a message indicating that the account number already exists. Otherwise, the account number is added as a key with the initial balance as the value.
The "make_deposit()" method takes an account number and an amount as arguments. It checks if the account number exists in the customers dictionary. If it does, it adds the amount to the current balance of the account. An error message is printed if the account number does not exist.
The "make_withdrawal()" method takes an account number and an amount as arguments. It checks if the account number exists in the customers dictionary. If it does, it checks if the account has sufficient funds to withdraw. If it does, it subtracts the amount from the current account balance. Otherwise, it prints an error message indicating insufficient funds. An error message is displayed if the account number does not exist.
The "check_balance()" method takes an account number as an argument. It checks if the account number exists in the customers dictionary. If it does, it retrieves and prints the current account balance. If the account number does not exist, it prints a message.
In the example usage section, we create an instance of the Bank class called bank. We use various methods to create customer accounts, make deposits, withdraw, and check account balances.
Flowchart:
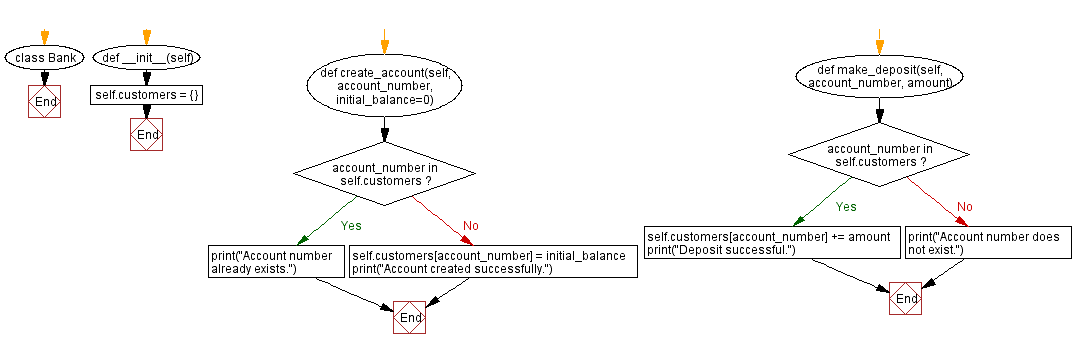
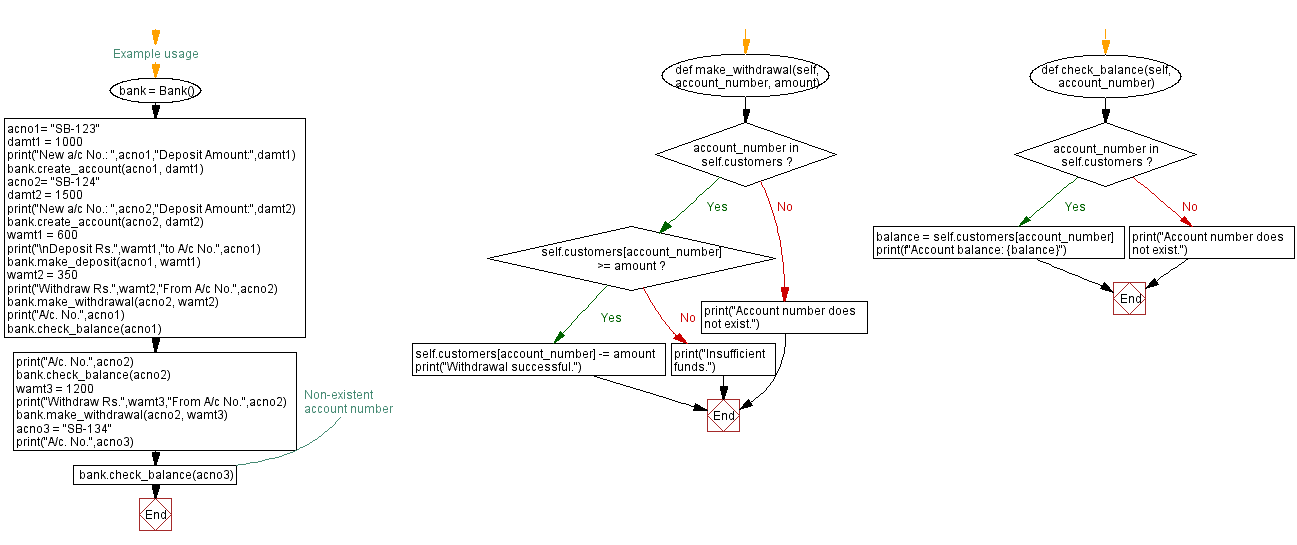
For more Practice: Solve these Related Problems:
- Write a Python class for a Bank that maintains customer accounts in a dictionary and supports deposit and withdrawal operations with error handling.
- Write a Python class for a Bank that logs transactions for each account and provides a method to print a detailed transaction history.
- Write a Python class for a Bank that implements a funds transfer method between accounts and verifies sufficient balance.
- Write a Python class for a Bank that includes a method to calculate and apply monthly interest to all customer accounts.
Go to:
Previous: Queue class with enqueue and dequeue methods.
Next: Python Decorator Exercises Home.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.