Python HTTP GET Request with Query parameters example
Python urllib3 : Exercise-3 with Solution
Write a Python program that uses query parameters in a GET request to filter or limit the results from an API.
Sample Solution:
Python Code :
# Import the urllib3 library
import urllib3
# Create a PoolManager instance to manage HTTP connections
http = urllib3.PoolManager()
# Define the API endpoint URL
api_url = 'https://jsonplaceholder.typicode.com/posts'
# Define query parameters
query_params = {
'userId': 1 # Replace with the desired user ID
}
# Make a GET request with query parameters
response = http.request('GET', api_url, fields=query_params)
# Check if the request was successful (status code 200)
if response.status == 200:
# Print the response data (decoded as UTF-8)
print("Response Data:")
print(response.data.decode('utf-8'))
else:
# Print an error message if the request was not successful
print(f"Error: Unable to fetch data. Status Code: {response.status}")
Sample Output:
Response Data: [ { "userId": 1, "id": 1, "title": "sunt aut facere repellat provident occaecati excepturi optio reprehenderit", "body": "quia et suscipit\nsuscipit recusandae consequuntur expedita et cum\nreprehenderit molestiae ut ut quas totam\nnostrum rerum est autem sunt rem eveniet architecto" }, { "userId": 1, "id": 2, "title": "qui est esse", "body": "est rerum tempore vitae\nsequi sint nihil reprehenderit dolor beatae ea dolores neque\nfugiat blanditiis voluptate porro vel nihil molestiae ut reiciendis\nqui aperiam non debitis possimus qui neque nisi nulla" }, { "userId": 1, "id": 3, "title": "ea molestias quasi exercitationem repellat qui ipsa sit aut", "body": "et iusto sed quo iure\nvoluptatem occaecati omnis eligendi aut ad\nvoluptatem doloribus vel accusantium quis pariatur\nmolestiae porro eius odio et labore et velit aut" }, { "userId": 1, "id": 4, "title": "eum et est occaecati", "body": "ullam et saepe reiciendis voluptatem adipisci\nsit amet autem assumenda provident rerum culpa\nquis hic commodi nesciunt rem tenetur doloremque ipsam iure\nquis sunt voluptatem rerum illo velit" }, { "userId": 1, "id": 5, "title": "nesciunt quas odio", "body": "repudiandae veniam quaerat sunt sed\nalias aut fugiat sit autem sed est\nvoluptatem omnis possimus esse voluptatibus quis\nest aut tenetur dolor neque" }, { "userId": 1, "id": 6, "title": "dolorem eum magni eos aperiam quia", "body": "ut aspernatur corporis harum nihil quis provident sequi\nmollitia nobis aliquid molestiae\nperspiciatis et ea nemo ab reprehenderit accusantium quas\nvoluptate dolores velit et doloremque molestiae" }, { "userId": 1, "id": 7, "title": "magnam facilis autem", "body": "dolore placeat quibusdam ea quo vitae\nmagni quis enim qui quis quo nemo aut saepe\nquidem repellat excepturi ut quia\nsunt ut sequi eos ea sed quas" }, { "userId": 1, "id": 8, "title": "dolorem dolore est ipsam", "body": "dignissimos aperiam dolorem qui eum\nfacilis quibusdam animi sint suscipit qui sint possimus cum\nquaerat magni maiores excepturi\nipsam ut commodi dolor voluptatum modi aut vitae" }, { "userId": 1, "id": 9, "title": "nesciunt iure omnis dolorem tempora et accusantium", "body": "consectetur animi nesciunt iure dolore\nenim quia ad\nveniam autem ut quam aut nobis\net est aut quod aut provident voluptas autem voluptas" }, { "userId": 1, "id": 10, "title": "optio molestias id quia eum", "body": "quo et expedita modi cum officia vel magni\ndoloribus qui repudiandae\nvero nisi sit\nquos veniam quod sed accusamus veritatis error" } ]
Explanation:
Here's a brief explanation of the above Python urllib3 library code:
- Import urllib3:
- Imports the urllib3 library for handling HTTP requests.
- Create a PoolManager instance:
- Initializes a PoolManager instance (http) to manage HTTP connections.
- Define the API endpoint URL:
- Specifies the URL of the API endpoint (api_url) that the script will interact with.
- Define the query parameters:
- Creates a dictionary (query_params) containing parameters for the GET request, such as 'userId' with a value of 1.
- Make a GET request with the query parameters:
- Sends a GET request to the specified API endpoint (api_url) with the defined query parameters using the http.request method. The response is stored in the response variable.
- Check if the request was successful:
- Checks if the HTTP status code of the response is 200, indicating a successful request.
- Print the response data or an error message:
- If the request was successful, print the decoded response data as UTF-8. Otherwise, it prints an error message with the HTTP status code.
Flowchart:
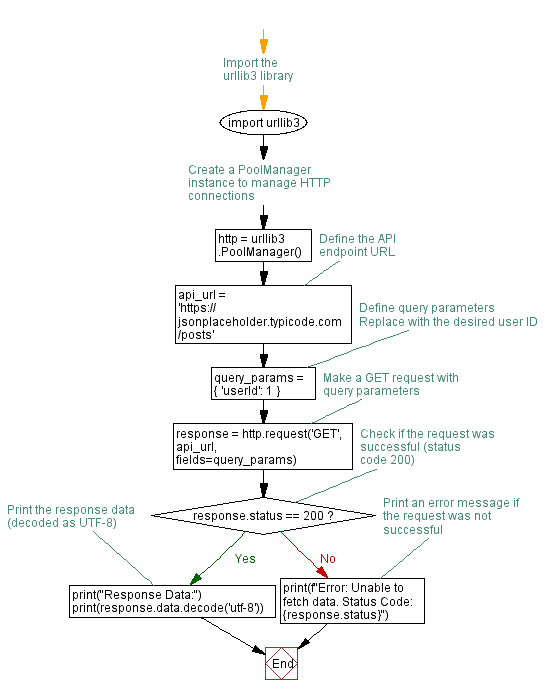
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Python HTTP GET Request with custom Headers Example.
Next: Python POST Request to Sample API with Data example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/urllib3/python-urllib3-exercise-3.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics