Python HTTP GET Request with custom Headers Example
Write a Python program that makes a GET request with custom headers, such as User-Agent and Authorization.
Sample Solution:
Python Code :
# Import the urllib3 library
import urllib3
# Create a PoolManager instance to manage HTTP connections
http = urllib3.PoolManager()
# Define the API endpoint URL
api_url = 'https://jsonplaceholder.typicode.com/posts/1'
# Define custom headers
custom_headers = {
'User-Agent': 'MyCustomUserAgent',
'Authorization': 'Bearer YourAccessTokenHere'
}
# Make a GET request with custom headers
response = http.request('GET', api_url, headers=custom_headers)
# Check if the request was successful (status code 200)
if response.status == 200:
# Print the response data (decoded as UTF-8)
print("Response Data:")
print(response.data.decode('utf-8'))
else:
# Print an error message if the request was not successful
print(f"Error: Unable to fetch data. Status Code: {response.status}")
Sample Output:
Response Data: { "userId": 1, "id": 1, "title": "sunt aut facere repellat provident occaecati excepturi optio reprehenderit", "body": "quia et suscipit\nsuscipit recusandae consequuntur expedita et cum\nreprehenderit molestiae ut ut quas totam\nnostrum rerum est autem sunt rem eveniet architecto" }
Explanation:
Here's a brief explanation of the above Python urllib3 library code:
- Import Library:
- Import the urllib3 library, which is used for HTTP requests.
- Create PoolManager:
- Create a PoolManager instance named 'http'. This manager is responsible for handling HTTP connections.
- Define API Endpoint URL:
- Set the URL of the public API endpoint. In this example, it's set to a specific resource (a post with ID 1) on JSONPlaceholder.
- Define Custom Headers:
- Create a dictionary (custom_headers) to store custom headers like 'User-Agent' and 'Authorization'. These headers may include information about the client and an authorization token.
- Make a GET Request:
- Use the http.request method to make a GET request to the specified API endpoint (api_url) with the custom headers.
- Check Response Status:
- Check if the response status code is 200, which indicates a successful request.
- Print Response Data or Error Message:
- If the request is successful (status code 200), print the decoded content of the response (UTF-8).
- If the request is not successful, print an error message along with the HTTP status code.
Flowchart:
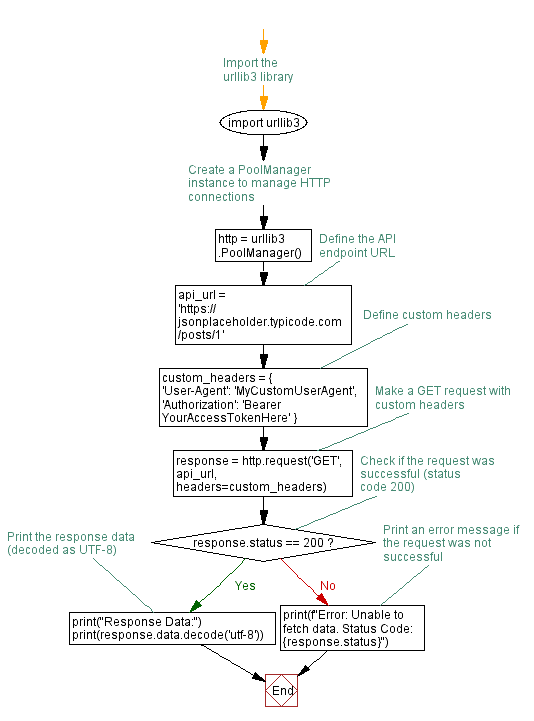
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Python HTTP GET Request to Public API example.
Next: Python HTTP GET Request with Query parameters example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.