Python HTTP GET Request to Public API example
Python urllib3 : Exercise-1 with Solution
Write a Python program that performs a simple HTTP GET request to a public API (e.g., JSONPlaceholder) and prints the response.
Sample Solution:
Python Code :
# Import the urllib3 library
import urllib3
# Create a PoolManager instance to manage HTTP connections
http = urllib3.PoolManager()
# Define the API endpoint URL
api_url = 'https://jsonplaceholder.typicode.com/posts/1'
# Make a GET request to the API endpoint
response = http.request('GET', api_url)
# Check if the request was successful (status code 200)
if response.status == 200:
# Print the response data (decoded as UTF-8)
print("Response Data:")
print(response.data.decode('utf-8'))
else:
# Print an error message if the request was not successful
print(f"Error: Unable to fetch data. Status Code: {response.status}")
Sample Output:
Response Data: { "userId": 1, "id": 1, "title": "sunt aut facere repellat provident occaecati excepturi optio reprehenderit", "body": "quia et suscipit\nsuscipit recusandae consequuntur expedita et cum\nreprehenderit molestiae ut ut quas totam\nnostrum rerum est autem sunt rem eveniet architecto" }
Explanation:
Here's a brief explanation of the above Python urllib3 library code:
- import urllib3: This line imports the 'urllib3' library, which is used for HTTP requests.
- http = urllib3.PoolManager(): It creates a PoolManager instance named 'http'. The 'PoolManager' manages connections to various hosts.
- api_url = 'https://jsonplaceholder.typicode.com/posts/1': It defines the URL of the public API endpoint. In this example, it's set to a specific resource (a post with ID 1) on JSONPlaceholder, a fake online REST API for testing and prototyping.
- response = http.request('GET', api_url): This line sends a GET request to the specified 'api_url' using the 'http' PoolManager. The response is stored in the response variable.
- if response.status == 200:: It checks if the HTTP status code of the response is 200, which indicates a successful request.
- print("Response Data:"): Prints a header indicating that the following lines will display the response data.
- print(response.data.decode('utf-8')): Prints the decoded content of the response. The decode('utf-8') is used to convert the binary response content to a UTF-8 string.
- else:: If the status code is not 200, this block is executed.
- print(f"Error: Unable to fetch data. Status Code: {response.status}"): Prints an error message along with the HTTP status code, indicating that the request was not successful.
Flowchart:
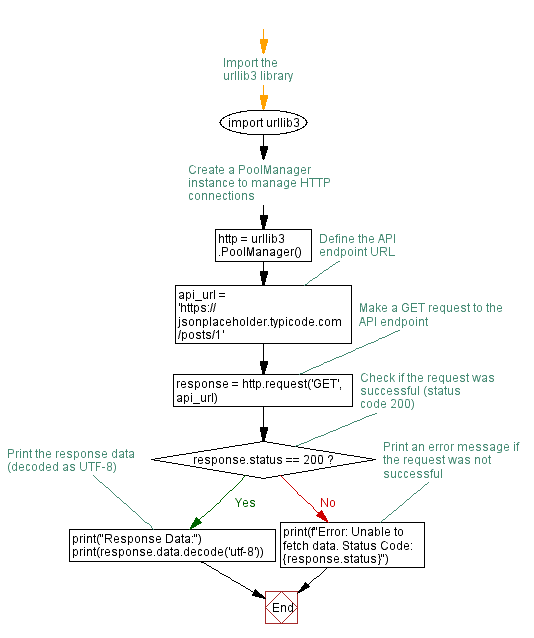
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: URLLIB3 Home.
Next: Python HTTP GET Request with custom Headers Example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics