Python POST Request to Sample API with Data example
Write a Python program that makes a POST request to a sample API with some data in the request body.
Sample Solution:
Python Code :
import urllib3
import json
def make_post_request():
# Create a PoolManager instance to manage HTTP connections
http = urllib3.PoolManager()
# Define the API endpoint URL
api_url = 'https://jsonplaceholder.typicode.com/posts'
# Define data to be sent in the request body
post_data = {
'title': 'Sample Title',
'body': 'This is the body of the post.',
'userId': 1
}
try:
# Encode the data as JSON
encoded_data = json.dumps(post_data).encode('utf-8')
# Make a POST request with the data
response = http.request('POST', api_url, body=encoded_data, headers={'Content-Type': 'application/json'})
# Check if the request was successful (status code 201 for created)
if response.status == 201:
# Print the response data
print("Post Request Successful:")
print(response.data.decode('utf-8'))
else:
# Print an error message if the request was not successful
print(f"Error: Unable to make POST request. Status Code: {response.status}")
except urllib3.exceptions.RequestError as e:
print(f"Error: {e}")
if __name__ == "__main__":
make_post_request()
Sample Output:
Post Request Successful: { "title": "Sample Title", "body": "This is the body of the post.", "userId": 1, "id": 101 }
Explanation:
Here's a brief explanation of the above Python urllib3 library code:
- Import Libraries:
- urllib3: Used for handling HTTP requests.
- json: Used for encoding and decoding JSON data.
- Define Function make_post_request:
- Encapsulates the logic for making a POST request.
- Create a PoolManager Instance:
- Initializes a urllib3.PoolManager() instance to manage HTTP connections.
- Define API Endpoint and Request Data:
- Specifies the API endpoint URL (api_url).
- Creates a dictionary (post_data) containing data to be sent in the POST request body.
- Make a POST Request:
- Uses http.request() to send a POST request to the API with the specified data.
- The request includes encoded JSON data in the request body.
- Check Response and Print Results:
- Checks the HTTP status code of the response.
- If the status code is 201 (created), print the response data.
- If not, prints an error message with the status code.
- Handle Exceptions:
- Uses a try-except block to catch potential "urllib3" request-related exceptions.
- Prints an error message if exceptions occur.
- Run the Function:
- Calls the make_post_request() function when the script is executed.
Flowchart:
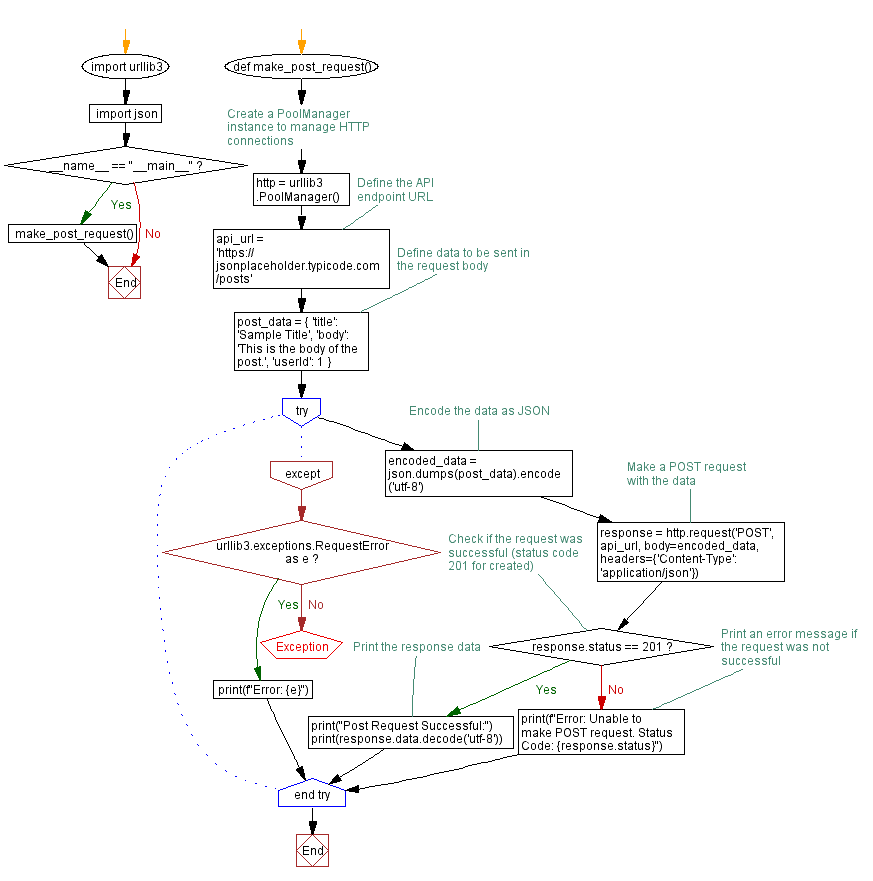
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Python HTTP GET Request with Query parameters example.
Next: Python GET Request with Timeout example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.