Python GET Request with Timeout example
Write a Python program that sets a timeout for a GET request to handle cases where the server takes too long to respond.
Sample Solution:
Python Code :
# Import the urllib3 library
import urllib3
def make_get_request_with_timeout():
# Create a PoolManager instance with a timeout of 5 seconds
http = urllib3.PoolManager(timeout=5)
# Define the API endpoint URL
api_url = 'https://jsonplaceholder.typicode.com/posts/1'
try:
# Make a GET request with the specified timeout
response = http.request('GET', api_url)
# Check if the request was successful (status code 200)
if response.status == 200:
# Print the response data
print("GET Request Successful:")
print(response.data.decode('utf-8'))
else:
# Print an error message if the request was not successful
print(f"Error: Unable to make GET request. Status Code: {response.status}")
except urllib3.exceptions.RequestError as e:
print(f"Error: {e}")
if __name__ == "__main__":
make_get_request_with_timeout()
Sample Output:
GET Request Successful: { "userId": 1, "id": 1, "title": "sunt aut facere repellat provident occaecati excepturi optio reprehenderit", "body": "quia et suscipit\nsuscipit recusandae consequuntur expedita et cum\nreprehenderit molestiae ut ut quas totam\nnostrum rerum est autem sunt rem eveniet architecto" }
Explanation:
Here's a brief explanation of the above Python urllib3 library code:
- Import Library:
- urllib3: Used for handling HTTP requests.
- Define Function make_get_request_with_timeout:
- Encapsulates the logic for making a GET request with a specified timeout.
- Create a PoolManager Instance with Timeout:
- Initializes a urllib3.PoolManager() instance (http) with a 5 second timeout.
- Define API Endpoint:
- Specifies the API endpoint URL (api_url) for the GET request.
- Make a GET Request with Timeout:
- Uses http.request() to send a GET request to the API with the specified timeout.
- Check Response and Print Results:
- Check the HTTP status code of the response.
- If the status code is 200, print the response data.
- If not, print an error message with the status code.
- Handle Exceptions:
- Uses a try-except block to catch potential "urllib3" request-related exceptions, such as timeouts.
- Prints an error message if exceptions occur.
- Run the Function:
- Calls the make_get_request_with_timeout() function when the script is executed.
Flowchart:
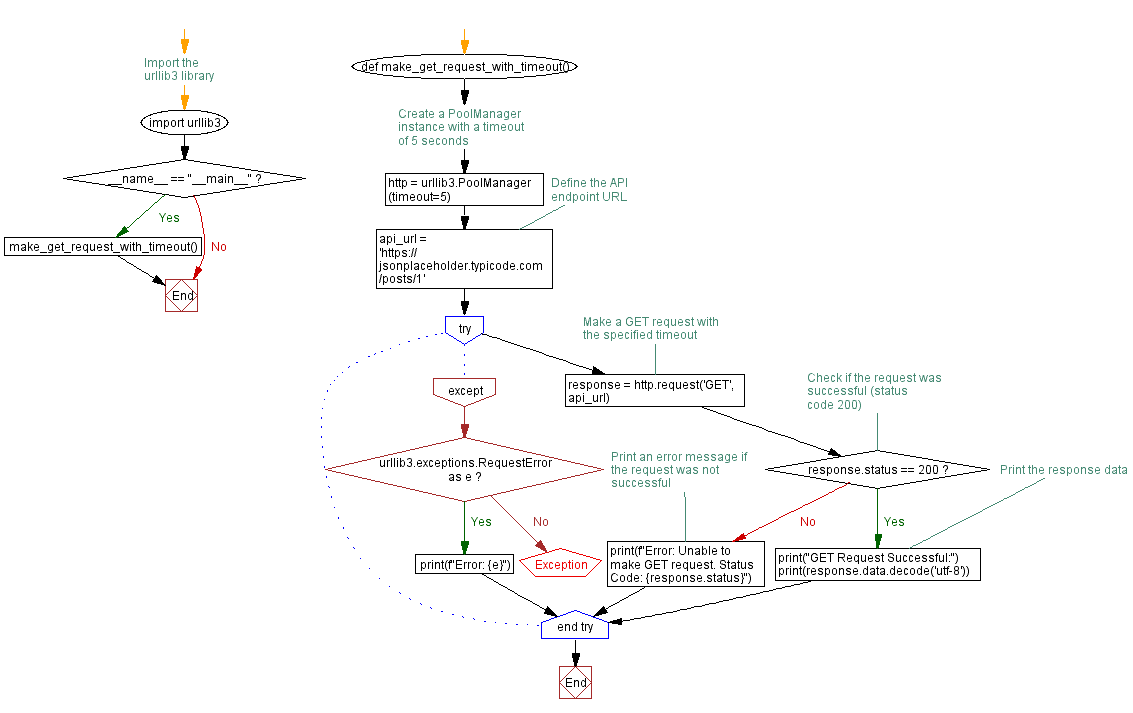
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Python POST Request to Sample API with Data example.
Next: Python Multiple Requests with Connection Pooling Example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.