Python Multiple Requests with Connection Pooling Example
Python urllib3 : Exercise-6 with Solution
Write a Python program that makes multiple requests to the same host and observe the benefits of connection pooling.
Sample Solution:
Python Code :
# Import the urllib3 library
import urllib3
def make_multiple_requests():
# Create a PoolManager instance to manage HTTP connections with connection pooling
http = urllib3.PoolManager()
# Define the API endpoint URL
api_url = 'https://jsonplaceholder.typicode.com/posts'
# Number of requests to make
num_requests = 5
try:
# Make multiple GET requests to the same host
for _ in range(num_requests):
response = http.request('GET', api_url)
# Check if the request was successful (status code 200)
if response.status == 200:
print("GET Request Successful:")
print(response.data.decode('utf-8'))
else:
print(f"Error: Unable to fetch data. Status Code: {response.status}")
except urllib3.exceptions.RequestError as e:
print(f"Error: {e}")
if __name__ == "__main__":
make_multiple_requests()
Sample Output:
GET Request Successful: [ { "userId": 1, "id": 1, "title": "sunt aut facere repellat provident occaecati excepturi optio reprehenderit", "body": "quia et suscipit\nsuscipit recusandae consequuntur expedita et cum\nreprehenderit molestiae ut ut quas totam\nnostrum rerum est autem sunt rem eveniet architecto" }, { "userId": 1, "id": 2, "title": "qui est esse", "body": "est rerum tempore vitae\nsequi sint nihil reprehenderit dolor beatae ea dolores neque\nfugiat blanditiis voluptate porro vel nihil molestiae ut reiciendis\nqui aperiam non debitis possimus qui neque nisi nulla" }, { "userId": 1, "id": 3, "title": "ea molestias quasi exercitationem repellat qui ipsa sit aut", "body": "et iusto sed quo iure\nvoluptatem occaecati omnis eligendi aut ad\nvoluptatem doloribus vel accusantium quis pariatur\nmolestiae porro eius odio et labore et velit aut" }, { "userId": 1, "id": 4, "title": "eum et est occaecati", "body": "ullam et saepe reiciendis voluptatem adipisci\nsit amet autem assumenda provident rerum culpa\nquis hic commodi nesciunt rem tenetur doloremque ipsam iure\nquis sunt voluptatem rerum illo velit" }, { "userId": 1, "id": 5, "title": "nesciunt quas odio", "body": "repudiandae veniam quaerat sunt sed\nalias aut fugiat sit autem sed est\nvoluptatem omnis possimus esse voluptatibus quis\nest aut tenetur dolor neque" }, { "userId": 1, "id": 6, "title": "dolorem eum magni eos aperiam quia", "body": "ut aspernatur corporis harum nihil quis provident sequi\nmollitia nobis aliquid molestiae\nperspiciatis et ea nemo ab reprehenderit accusantium quas\nvoluptate dolores velit et doloremque molestiae" }, -------------------------------------------------------- -------------------------------------------------------- -------------------------------------------------------- { "userId": 10, "id": 100, "title": "at nam consequatur ea labore ea harum", "body": "cupiditate quo est a modi nesciunt soluta\nipsa voluptas error itaque dicta in\nautem qui minus magnam et distinctio eum\naccusamus ratione error aut" } ]
Explanation:
Here's a brief explanation of the above Python urllib3 library code:
- Import urllib3:
- Imports the urllib3 library for handling HTTP requests and connection pooling.
- Create a PoolManager instance:
- Initializes a PoolManager instance ('http') to manage HTTP connections with connection pooling.
- Define API endpoint:
- Specifies the API endpoint URL ('api_url') for multiple GET requests.
- Number of requests to make:
- Sets the variable 'num_requests' to 5, indicating the number of GET requests to make.
- Make multiple GET requests:
- Uses a loop to make multiple GET requests to the same host using the 'http.request' method.
- Check if the requests were successful:
- Checks the HTTP status code of each response. If it's 200 (OK), print the response data; otherwise, print an error message.
- Handle exceptions:
- Uses a try-except block to handle potential exceptions during the requests, printing an error message if any occur.
- Run the program:?
- Calls the make_multiple_requests function when the script is executed.
Flowchart:
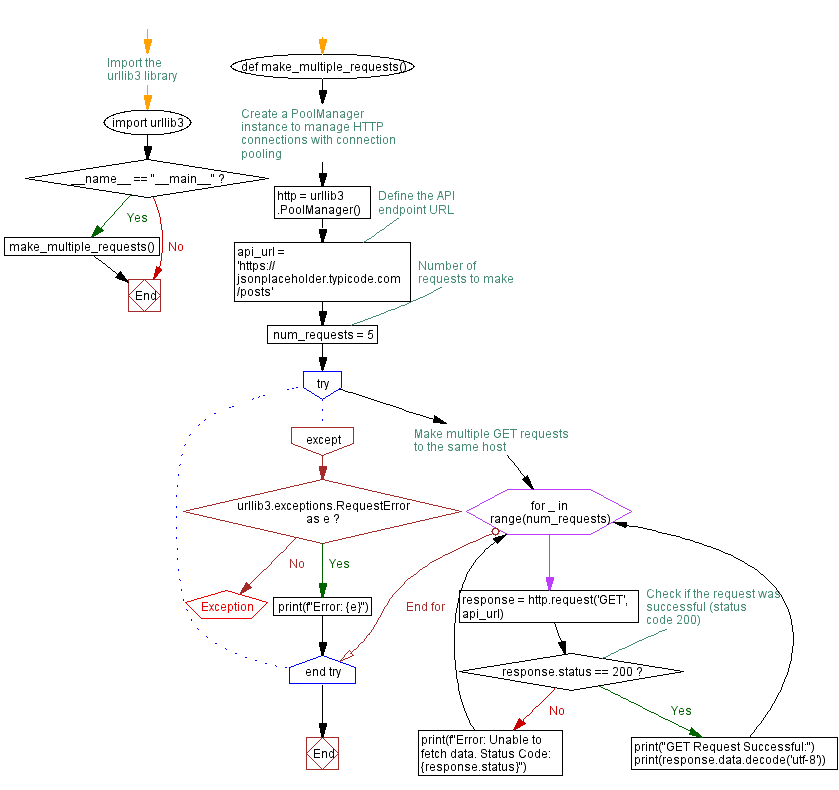
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Python GET Request with Timeout example.
Next: Python Redirect Handling and final URL example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics