Node.js : Global Objects
Introduction
Node.js has a number of built-in global identifiers. These objects are available in all modules. Some of these objects are true globals and can be accessed from anywhere, other exist at module level in every module.
Contents:
global
The global namespace. Setting a property to this namespace makes it globally visible within the running process.
Type : object
In the browser, the top-level property is the global scope. Therefore within the browser, the var var_name will define a global variable. In Node var var_name inside a module is the local to that module i.e. top-level scope is not the global scope.
console
This built-in object is used to print stdout and stderr. The object has several methods, see details in console section.
Type : object
Here is a simple example that prints a string.
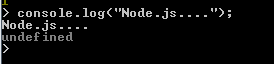
process
A process object is a global object, which provides interaction with the current Node process and can be accessed from anywhere.
Type : object
In the following example, the exit event fires whenever the process is about to exit.
var fs = require('fs');
process.on('exit', function () {
var content = fs.readFileSync("readme.txt", "utf8");
});
Class: Buffer
The Buffer class is a global. It deals with binary data directly and can be constructed in a variety of ways. See details in buffer section.
Type : function
In the following example, roll_no contains the contents of an array. See the output.
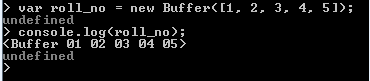
require()
The require() function is a built-in function, and used to include other modules that exist in separate files, a string specifying the module to load. It accepts a single argument. It is not global but rather local to each module.
Type : function
In the following example 'fs' (file system) module is included.
var fs = require('fs');
var content = fs.readFileSync("readme.txt", "utf8");
console.log(content);
console.log('Reading file...');
require.resolve()
require.resolve() is used to search the location of a module and returns the resolved filename. Here is an example
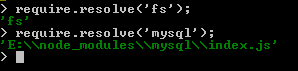
The first command require.resolve('fs') displays just 'fs' without any path as it is a default module comes with Node. The second one displays the entire path of 'mysql' module which is a third party module.
require.cache
Modules are cached (contains all the current loaded modules) in this object. You can clear a module by deleting a key value from this object. You can load it again using require().
Type : object
filename
__filename contains the absolute path of the currently executing file. This is not present in the Node REPL.
Type : string
Here is an example.
Code (test.js) :
console.log(__filename);
Output :

dirname
__dirname contains the path to the root directory of the currently executing script. This is not present in the Node REPL.
Type : string
Here is an example.
Code (test.js) :
console.log(__dirname);
Output :

module
A reference to the current module. In a particular program, a module becomes available through require(). It is not global but rather local to each module.
Type : Object
In the following example 'fs' module is used to read a file :
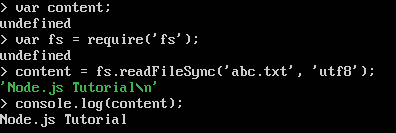
exports
A reference to the module.exports that is shorter to type. exports isn't actually a global but rather local to each module.
setTimeout(cb, ms)
The setTimeout() calls a function (cb) after a specified number of milliseconds (ms). The timeout must be in the range of 1-2,147,483,647 inclusive. If the value is outside that range, it's changed to 1 millisecond. Here is an example :
setTimeout(function(){
console.log('I have come after 500 miliseconds')
},500);
Output :

clearTimeout(t)
The clearTimeout() is used to cancel a timeout that was set with setTimeout(). The callback will not execute.
setInterval(cb, ms)
setinterval() calls a function (cb) repeatedly at specified intervals (in milliseconds (ms)). The interval must be in the range of 1-2,147,483,647 inclusive. If the value is outside that range, it's changed to 1 millisecond.
Note : The actual interval may vary, depending on external factors like OS timer granularity and system load. It's never less than ms but it may be longer.
In the following example, 'Node.js' string will print repeatedly in every 300 milliseconds.
setInterval(function(){
console.log('Node.js')
}, 300);
Output :
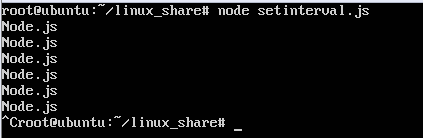
clearInterval(t)
The clearInterval() is used to stop a timer that was set with setInterval(). The callback will not execute.
Note : All of the timer functions are global variables.
Previous:
Node Package Manager
Next:
Http Module