Node.js : Console Logging
Introduction
If you start the Node.js binary without any arguments, you will see the REPL command prompt, the > character and from this prompt ( interactive shell ) you can execute raw JavaScript code you wish. Those who are familiar with browser-side development has probably used console.log for writing information to the console and debugging purposes.
Similar to the Node.js, there is a built-in console object with several methods which works for printing to stdout and stderr.
List of Methods
Contents:
console.log([data], [...])
The console.log() method is used to print to stdout with newline. Like printf() the function can take multiple arguments. Here is a simple example :
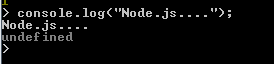
In the above example, we have executed a simple command console.log("Node.js....") and the REPL prints the provided string to stdout along with a string 'undefined' (as console.log doesn't return anything). You can also execute the code, providing it a JavaScript file. Let put the code within test.js and execute it.
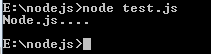
You can use the format characters to insert string, integer, or JSON data into your output. When you will use multiple format characters the order of the format characters must match the order of arguments. In the following example we have used three format character %s (represent string), %d (represent integer), and %j (represent JSON data). See the following example :
JavaScript Code (me.js) :
var my_name = 'Sunita',
my_class = 5,
my_fav_subject= {
subj1: 'English',
subj2: 'Math.'};
console.log('My name is %s,'
+'\n'+ 'I read in class %d,'
+'\n'+ 'Myfavourite subjects are %j',
my_name, my_class, my_fav_subject);

console.info([data], [...])
The method is same as console.log. Here are some examples :
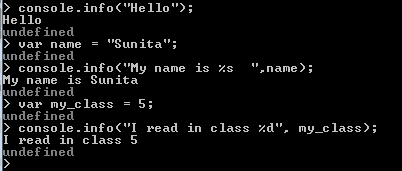
console.error([data], [...])
The console.error() method works the same as console.log, except that the output is sent to stderr instead of stdout. As stderr is always written to synchronously, therefore in node.js any use of console.error, or other functions that write to stderr, will block your process until the output has all been written. The method is useful for error messages, but excessive use could slow down your process. There is various error format for log output.
In the following example, the 'mysql' module is used to access the rows of a particular file. If the table connected successfully than the first row of the table will be printed to stdout using console.log. However, if an error occurs, it is logged to stderr using console.error(). Here is the code and output :
connection.js :
var my_name = 'Sunita',
var mysql = require('mysql');
var connection = mysql.createConnection({
host : 'localhost',
user : 'root',
password : 'datasoft123',
database : 'hr'
});
connection.connect();
connection.query('SELECT * FROM department', function(err, results)
{
if (err)
{
console.error(err);
}
else
{
console.log('First row of department table : ', results[0]);
}
});
connection.end();
Output :

You can show the error throguh stack trace. Here is the code and output :
var my_name = 'Sunita',
var mysql = require('mysql');
var connection = mysql.createConnection({
host : 'localhost',
user : 'root',
password : 'datasoft123',
database : 'hr'
});
connection.connect();
connection.query('SELECT * FROM department', function(err, results)
{
if (err)
{
console.error(err.stack);
}
else
{
console.log('First row of department table : ', results[0]);
}
});
connection.end();
Output :
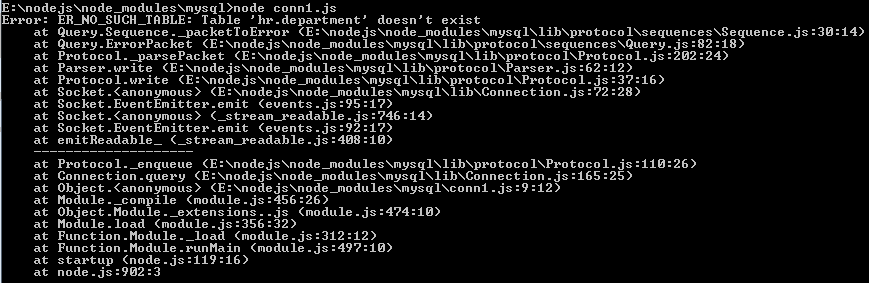
The stack property contains the message as well as the stack. You can show only the error message. Here is the code and output :
var my_name = 'Sunita',
var mysql = require('mysql');
var connection = mysql.createConnection({
host : 'localhost',
user : 'root',
password : 'datasoft123',
database : 'hr'
});
connection.connect();
connection.query('SELECT * FROM department', function(err, results)
{
if (err)
{
console.error(err.message);
}
else
{
console.log('First row of department table : ', results[0]);
}
});
connection.end();
Output :

You can show the error message in JSON way also. Here is the code and output :
var my_name = 'Sunita',
var mysql = require('mysql');
var connection = mysql.createConnection({
host : 'localhost',
user : 'root',
password : 'datasoft123',
database : 'hr'
});
connection.connect();
connection.query('SELECT * FROM department', function(err, results)
{
if (err)
{
console.error(JSON.stringify(err));
}
else
{
console.log('First row of department table : ', results[0]);
}
});
connection.end();
Output:

console.warn([data], [...])
The console.warn() method is same as console.error() method. In the following example, the 'fs' module is used to open a particular file. If the file opens successfully, than console.log() prints a message. However, if an error occurs, it is logged to stderr using console.warn(). Here is the code and output :
var fd = require("fs");
var path = "note.txt";
fd.open(path, "r", function(err, fd){
if (err){
console.warn(err);
}
else{
console.log("File open successfully" + path);
}
})
Output:

console.dir(obj)
Uses util.inspect on obj and prints resulting string to stdout.
console.time(label)
The console.time() method starts a timer and you can use it to track how long an operation takes. Each time must have and unique name (label). When you call console.timeEnd() with the same name, it prints the amount of time that has passed since a particular process started. See the next example.
console.timeEnd(label)
The console.timeEnd() method is used to stop a timer that was previously started by calling console.time() method.
Example :
console.time('Mytimer');
var sum = 0;
for (var x=0; x<100000; x++)
{
sum += x;
}
//console.log(/'sum=/'+sum);
console.log('Time taken Sum up numbers from 1 to 100000');
console.timeEnd('Mytimer');
Output:

console.trace(label)
The console.trace() method is used to prints a stack trace to stderr of the current position. See the following examples :
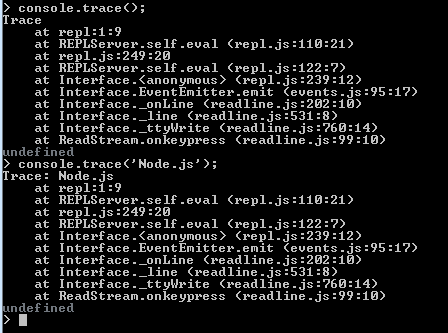
console.assert(expression, [message])
The console.assert() method tests whether an expression is true. If the expression evaluates as false it will throw an AssertionError with a message.
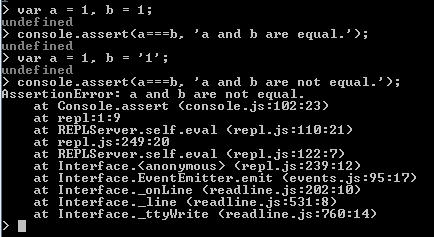
Previous:
Util Module
Next:
Buffer