JavaScript: Logical Operators - AND, OR, NOT
Logical Operators
The logical operators used in Javascript are listed below:
Operator | Usage | Description |
---|---|---|
Logical AND ( && ) | a && b | is true if both a and b are true. |
Logical OR ( || ) | a || b | is true if either a or b is true. |
Logical NOT ( ! ) | !a | is true if a is not true. |
JavaScript Logical AND operator (&&)
The following conditions are true :
- true && true
- (20 > 5) && (5 < 20)
The following conditions are false :
- true && false
- (20 > 5) && (20 < 5)
- false && true
- (20 < 5) && (20 > 5)
- false && false
- (20 < 5) && (5 > 20)
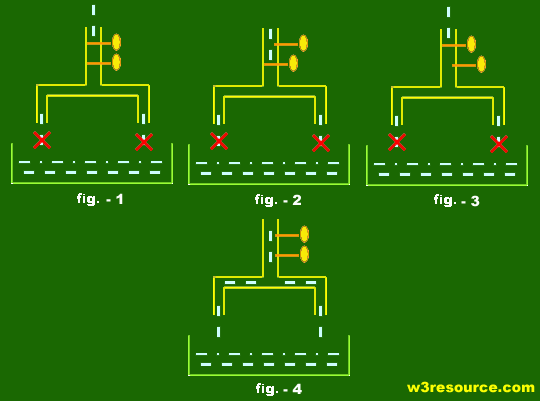
This above pictorial helps you to understand the concept of LOGICAL AND operation with an analogy of taps and water.
In fig.-1 of the picture, both of the taps are closed, so the water is not flowing down. Which explains that if both of conditions are FALSE or 0, the return is FALSE or 0.
In fig.-2 of the picture, one of the taps are closed, even then, the water is not flowing down. Which explains that even if any of conditions are FALSE or 0, the return is FALSE or 0.
fig.-3 of the picture resembles CASE -2.
In fig.-4 of the picture, both of the taps are open, so the water is flowing down. Which explains that if both of conditions are TRUE or 1, the return is TRUE or 1.
So we can conclude that if and only if, both of the conditions are TRUE or 1, LOGICAL AND operations returns TRUE or 1.
Example:
The following web document demonstrates the use of AND operator (&&).
HTML Code
<!doctype html>
<head>
<meta charset="utf-8">
<title>JavaScript logical AND operator example</title>
<meta name="description" content="This document contains an example of JavaScript logical AND operator"/>
<style>
h1 {
color:red;
border-bottom: 3px groove silver;
padding-bottom: 8px;
}
</style>
</head>
<body>
<h1>JavaScript equal operator (==) example</h1>
<form name="logical_or_test" action="javascript-logical-and-operator-example1-with-dom.html">
<input type="text" id="no" placeholder="Input a number between 5 and 10 and press tab" size="60" onchange="viewOutput();"/>
<input type="submit" value="Submit" />
</form>
<script src="javascript-logical-and-operator-example1.js"></script>
</body>
</html>
JS Code
function viewOutput()
{
'use strict';
var no = document.getElementById('no').value;
if( no>= 5 && no<10 )
{
var newParagraph = document.createElement("p"); //creates a new paragraph element
var newText = document.createTextNode('The number is between 5 and 10'); //creates text along with ouput to be displayed
newParagraph.appendChild(newText); //created text is appended to the paragraph element created
document.body.appendChild(newParagraph); // created paragraph and text along with output is appended to the document body
}
else
{
var newParagraph1 = document.createElement("p"); //creates a new paragraph element
var newText1 = document.createTextNode('Wrong input'); //creates text along with ouput to be displayed
newParagraph1.appendChild(newText1); //created text is appended to the paragraph element created
document.body.appendChild(newParagraph1); // created paragraph and text along with output is appended to the document body
}
}
View the example in the browser
JavaScript Logical OR operator (||)
The following conditions are true :
- true || true
- (20 > 5) || (5 < 20)
- true || false
- (20 > 5) || (20 < 5)
- false || true
- (20 < 5) || (20 > 5)
The following conditions are false :
- false || false
- (20 < 5) || (5 > 20)
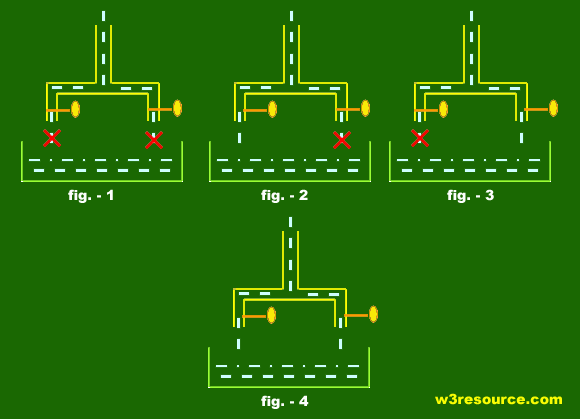
The above pictorial helps you to understand the concept of LOGICAL OR operation with an analogy of taps and water.
In fig.-1 of the picture, both of the taps are closed, so the water is not flowing down. Which explains that if both of conditions are FALSE or 0, the return is FALSE or 0.
In fig.-2 of the picture, one of the taps are closed, and we can see that the water is flowing down. Which explains that if any of conditions are TRUE or 1, the return is TRUE or 1.
fig.-3 of the picture resembles CASE -2.
In fig.-4 of the picture, both of the taps are open, so the water is flowing down. Which explains that if both of conditions are TRUE or 1, the return is TRUE or 1.
So we can conclude that in LOGICAL OR operation if any of the conditions are true, the output is TRUE or 1.
Example:
The following web document demonstrates the use of OR operator (||).
<!doctype html>
<head><meta charset="utf-8">
<title>JavaScript logical OR operator example</title>
<meta name="description" content="This document contains an example of JavaScript logical OR operator"/>
<style>
h1 {
color:red;
border-bottom: 3px groove silver;
padding-bottom: 8px;
}</style>
</head>
<h1>JavaScript logical OR operator example</h1>
<form name="logical_or_test" action="javascript-logical-or-operator-example1-with-dom.html">
<input type="text" id="no" placeholder="Input a number less than 5 or greater than 20 and press tab" size="60" onchange="viewOutput();"/>
<input type="submit" value="Submit" />
</form>
<script src="javascript-logical-or-operator-example1.js">
</script>
</body>
</html>
JS Code
function viewOutput()
{
'use strict';
var no = document.getElementById('no').value;
if( no<5 || no>20 )
{
var newParagraph = document.createElement("p"); //creates a new paragraph element
var newText = document.createTextNode('Value of input is less than 5 or greater than 20'); //creates text along with ouput to be displayed
newParagraph.appendChild(newText); //created text is appended to the paragraph element created
document.body.appendChild(newParagraph); // created paragraph and text along with output is appended to the document body
}
else
{
var newParagraph1 = document.createElement("p"); //creates a new paragraph element
var newText1 = document.createTextNode('Wrong input'); //creates text along with ouput to be displayed
newParagraph1.appendChild(newText1); //created text is appended to the paragraph element created
document.body.appendChild(newParagraph1); // created paragraph and text along with output is appended to the document body
}
}
View the example in the browser
JavaScript Logical NOT operator (!)
HTML Code
<!doctype html>
<head>
<meta charset="utf-8">
<title>JavaScript logical NOT operator example with DOM</title>
<meta name="description" content="This document contains an example of JavaScript logical NOT operator"/>
<style>
h1 {
color:red;
border-bottom: 3px groove silver;
padding-bottom: 8px;
}
</style>
</head>
<h1>JavaScript logical NOT operator example</h1>
<script src="javascript-logical-not-operator-example1.js"></script>
</body>
</html>
JS Code
var a = 20;
var b = 5;
var newParagraph = document.createElement("p"); //creates a new paragraph element
var newText = document.createTextNode('Value of a = '+a+' b = '+b); //creates text along with ouput to be displayed
newParagraph.appendChild(newText); //created text is appended to the paragraph element created
document.body.appendChild(newParagraph); // created paragraph and text along with output is appended to the document body
if( a != b )
{
var newParagraph1 = document.createElement("p"); //creates a new paragraph element
var newText1 = document.createTextNode('a is not equal to b [ != operator ]'); //creates text along with ouput to be displayed
newParagraph1.appendChild(newText1); //created text is appended to the paragraph element created
document.body.appendChild(newParagraph1); // created paragraph and text along with output is appended to the document body
}
else
{
var newParagraph2 = document.createElement("p"); //creates a new paragraph element
var newText2 = document.createTextNode('a is equal to b.'); //creates text along with ouput to be displayed
newParagraph2.appendChild(newText2); //created text is appended to the paragraph element created
document.body.appendChild(newParagraph2); // created paragraph and text along with output is appended to the document body
}
View the example in the browser
Want to Test your JavaScript skill ?
Want to Practice JavaScript exercises ?
- JavaScript basic [ 13 Exercises with Solution ]
- JavaScript functions [ 21 Exercises with Solution ]
- JavaScript conditional statements and loops [ 10 Exercises with Solution ]
- JavaScript array [ 13 Exercise with Solution ]
- JavaScript regular expression [ 6 Exercises with Solution ]
- JavaScript HTML DOM [ 14 Exercises with Solution ]
- JavaScript Drawing [ 5 Exercises with Solution ]
- JavaScript Object [ 4 Exercises with Solution ]
Previous: JavaScript: Comparison Operators
Next: JavaScript: String Operators
Test your Programming skills with w3resource's quiz.