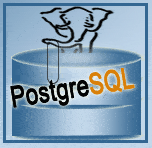
1. Write a sql statement to insert a record with your own value into the table countries against each columns.nd region_id.
Here in the following is the structure of the table countries.
Column | Type | Modifiers --------------+-----------------------+----------- country_id | character varying(2) | country_name | character varying(40) | region_id | numeric(10,0) |
INSERT INTO countries VALUES('C1','India',1002);
postgres=# SELECT * FROM countries; country_id | country_name | region_id ------------+--------------+----------- C1 | India | 1002 (1 row)
2. Write a sql statement to insert one row into the table countries against the column country_id and country_name.
Here in the following is the structure of the table countries.
Column | Type | Modifiers --------------+-----------------------+----------- country_id | character varying(2) | country_name | character varying(40) | region_id | numeric(10,0) |
INSERT INTO countries (country_id,country_name) VALUES('C2','USA');
postgres=# SELECT * FROM countries; country_id | country_name | region_id ------------+--------------+----------- C1 | India | 1002 C2 | USA |
3. Write a sql statement to create duplicate of countries table named country_new with all structure and data.
Here in the following is the structure of the table countries.
Column | Type | Modifiers --------------+-----------------------+----------- country_id | character varying(2) | country_name | character varying(40) | region_id | numeric(10,0) |
CREATE TABLE country_new AS SELECT * FROM countries;
postgres=# \d country_new; Column | Type | Modifiers --------------+-----------------------+----------- country_id | character varying(2) | country_name | character varying(40) | region_id | numeric(10,0) | postgres=# SELECT * FROM country_new; country_id | country_name | region_id ------------+--------------+----------- C1 | India | 1002 C2 | USA | (2 rows)
4. Write a sql statement to insert NULL values against region_id column for a row of countries table.
INSERT INTO countries (country_id,country_name,region_id) VALUES('C3','UK',NULL);
postgres=# SELECT * FROM countries; country_id | country_name | region_id ------------+--------------+----------- C1 | India | 1002 C2 | USA | C3 | UK | (3 rows)
5. Write a sql statement to insert 3 rows by a single insert statement.
INSERT INTO countries VALUES('C4','India',1001), ('C5','USA',1007),('C6','UK',1003);
postgres=# SELECT * FROM countries; country_id | country_name | region_id ------------+--------------+----------- C1 | India | 1002 C2 | USA | C3 | UK | C4 | India | 1001 C5 | USA | 1007 C6 | UK | 1003 (6 rows)
6. Write a sql statement insert rows from country_new table to countries table.
Here is the rows for country_new table. Assume that, the countries table is empty.
country_id | country_name | region_id ------------+--------------+----------- C1 | India | 1002 C2 | USA | C3 | UK | C4 | India | 1001 C5 | USA | 1007 C6 | UK | 1003 (6 rows)
INSERT INTO countries SELECT * FROM country_new;
postgres=# SELECT * FROM countries; country_id | country_name | region_id ------------+--------------+----------- C1 | India | 1002 C2 | USA | C3 | UK | C4 | India | 1001 C5 | USA | 1007 C6 | UK | 1003 (6 rows)
7. Write a sql statement to insert one row in jobs table to ensure that no duplicate value will be entered in the job_id column.
Create the table jobs. CREATE TABLE jobs ( JOB_ID integer NOT NULL UNIQUE , JOB_TITLE varchar(35) NOT NULL, MIN_SALARY decimal(6,0) ); INSERT INTO jobs VALUES(1001,'OFFICER',8000); postgres=# SELECT * FROM jobs; job_id | job_title | min_salary --------+-----------+------------ 1001 | OFFICER | 8000 (1 row)
INSERT INTO jobs VALUES(1001,'OFFICER',8000);
postgres=# INSERT INTO jobsn VALUES(1001,'OFFICER',8000); ERROR: duplicate key value violates unique constraint "jobs_job_id_key" DETAIL: Key (job_id)=(1001) already exists.
8. Write a sql statement to insert one row in jobs table to ensure that no duplicate value will be entered in the job_id column.
Create the table jobs. CREATE TABLE jobs ( JOB_ID integer NOT NULL UNIQUE PRIMARY KEY, JOB_TITLE varchar(35) NOT NULL, MIN_SALARY decimal(6,0) ); INSERT INTO jobs VALUES(1001,'OFFICER',8000); postgres=# SELECT * FROM jobs; job_id | job_title | min_salary | max_salary --------+-----------+------------+------------ 1001 | OFFICER | 8000 | (1 row)
INSERT INTO jobs VALUES(1001,'OFFICER',8000);
postgres=# INSERT INTO jobsn VALUES(1001,'OFFICER',8000); ERROR: duplicate key value violates unique constraint "jobs_pkey" DETAIL: Key (job_id)=(1001) already exists.
9. Write a sql statement to insert a record into the table countries to ensure that, a country_id and region_id combination will be entered once in the table.
Create the table countries. CREATE TABLE countries ( COUNTRY_ID integer NOT NULL, COUNTRY_NAME varchar(40) NOT NULL, REGION_ID integer NOT NULL, PRIMARY KEY (COUNTRY_ID,REGION_ID) ); INSERT INTO countries VALUES(501,'India',185); postgres=# SELECT * FROM countries; country_id | country_name | region_id ------------+--------------+----------- 501 | India | 185 (1 row)
INSERT INTO countries VALUES(501,'Italy',185);
postgres=# INSERT INTO kan VALUES(501,'Italy',185); ERROR: duplicate key value violates unique constraint "countries_pkey" DETAIL: Key (country_id, region_id)=(501, 185) already exists.
10. Write a sql statement to insert rows into the table countries in which the value of country_id column will be unique and auto incremented.
Create the table countries. CREATE TABLE countries ( COUNTRY_ID SERIAL PRIMARY KEY, COUNTRY_NAME varchar(40) NOT NULL, REGION_ID integer NOT NULL );
INSERT INTO countries(COUNTRY_NAME,REGION_ID) VALUES('India',185);
postgres=# SELECT * FROM countries; country_id | country_name | region_id ------------+--------------+----------- 1 | India | 185 (1 row)
INSERT INTO countries(COUNTRY_NAME,REGION_ID) VALUES('Japan',102);
postgres=# SELECT * FROM countries; country_id | country_name | region_id ------------+--------------+----------- 1 | India | 185 2 | Japan | 102 (2 rows)
11. Write a sql statement to insert records into the table countries to ensure that the country_id column will not contain any duplicate data and this will be automatically incremented and the column country_name will be filled up by 'N/A' if no value assigned for that column.
Create the table countries. CREATE TABLE countries ( COUNTRY_ID SERIAL PRIMARY KEY, COUNTRY_NAME varchar(40) NOT NULL DEFAULT 'N/A', REGION_ID integer NOT NULL );
INSERT INTO countries VALUES(501,'India',102);
postgres=# SELECT * FROM countries; country_id | country_name | region_id ------------+--------------+----------- 501 | India | 102 (1 row)
INSERT INTO countries(region_id) VALUES(109);
postgres=# SELECT * FROM countries; country_id | country_name | region_id ------------+--------------+----------- 501 | India | 102 1 | N/A | 109 (2 rows)
INSERT INTO countries(country_name,region_id) VALUES('Australia',121);
postgres=# SELECT * FROM countries; country_id | country_name | region_id ------------+--------------+----------- 501 | India | 102 1 | N/A | 109 2 | Australia | 121 (3 rows)
12. Write a sql statement to insert rows in the job_history table in which one column job_id is containing those values which are exists in job_id column of jobs table.
Sample table jobs. CREATE TABLE jobs ( JOB_ID integer NOT NULL UNIQUE PRIMARY KEY, JOB_TITLE varchar(35) NOT NULL DEFAULT ' ', MIN_SALARY decimal(6,0) DEFAULT 8000, MAX_SALARY decimal(6,0) DEFAULT 20000 ); INSERT INTO jobs(JOB_ID,JOB_TITLE) VALUES(1001,'OFFICER'); INSERT INTO jobs(JOB_ID,JOB_TITLE) VALUES(1002,'CLERK'); job_id | job_title | min_salary | max_salary --------+-----------+------------+------------ 1001 | OFFICER | 8000 | 20000 1002 | CLERK | 8000 | 20000 (2 rows) Sample table job_history; CREATE TABLE job_history ( EMPLOYEE_ID integer NOT NULL PRIMARY KEY, JOB_ID integer NOT NULL, DEPARTMENT_ID integer DEFAULT NULL, FOREIGN KEY (job_id) REFERENCES jobs(job_id) );
Now insert the rows in the job_history.
INSERT INTO job_history VALUES(501,1001,60);
postgres=# SELECT * FROM job_history; employee_id | job_id | department_id -------------+--------+--------------- 501 | 1001 | 60 (1 row)
The value against job_id is 1001 which is exists in the job_id column of the jobs table, so no problem arise.
Now insert another row in the job_history table.
INSERT INTO job_history VALUES(502,1003,80);
postgres=# INSERT INTO job_history VALUES(502,1003,80); ERROR: insert or update on table "job_history" violates foreign key constraint "job_history_job_id_fkey" DETAIL: Key (job_id)=(1003) is not present in table "jobs".
Here in the above, the value against job_id is 1003 which is not exists in the job_id column of the jobs(parent table) table and that is why the child table job_history can not contain the value of job_id as specified. Here the primary key - foreign key relationship is violating and shows the above message.
13. Write a sql statement to insert rows into the table employees in which a set of columns department_id and manager_id contains a unique value and that combined values must have exists into the table departments.
Sample table departments. CREATE TABLE departments ( DEPARTMENT_ID integer NOT NULL UNIQUE, DEPARTMENT_NAME varchar(30) NOT NULL, MANAGER_ID integer DEFAULT NULL, LOCATION_ID integer DEFAULT NULL, PRIMARY KEY (DEPARTMENT_ID,MANAGER_ID) ); INSERT INTO departments VALUES(60,'SALES',201,89); INSERT INTO departments VALUES(61,'ACCOUNTS',201,89); INSERT INTO departments VALUES(80,'FINANCE',211,90); postgres=# SELECT * FROM departments; department_id | department_name | manager_id | location_id ---------------+-----------------+------------+------------- 60 | SALES | 201 | 89 61 | ACCOUNTS | 201 | 89 80 | FINANCE | 211 | 90 (3 rows) Sample table employees. CREATE TABLE employees ( EMPLOYEE_ID integer NOT NULL PRIMARY KEY, FIRST_NAME varchar(20) DEFAULT NULL, LAST_NAME varchar(25) NOT NULL, JOB_ID varchar(10) NOT NULL, SALARY decimal(8,2) DEFAULT NULL, MANAGER_ID integer DEFAULT NULL, DEPARTMENT_ID integer DEFAULT NULL, FOREIGN KEY(DEPARTMENT_ID,MANAGER_ID) REFERENCES departments(DEPARTMENT_ID,MANAGER_ID) );
Now insert the rows in the employees.
INSERT INTO employees VALUES(510,'Alex','Hanes','CLERK',18000,201,60); INSERT INTO employees VALUES(511,'Kim','Leon','CLERK',18000,211,80);
postgres=# SELECT * FROM employees; employee_id | first_name | last_name | job_id | salary | manager_id | department_id -------------+------------+-----------+--------+----------+------------+--------------- 510 | Alex | Hanes | CLERK | 18000.00 | 201 | 60 511 | Kim | Leon | CLERK | 18000.00 | 211 | 80 (2 rows)
The value against department_id and manager_id combination (60,201) and (80,211) are unique in the departmentis(parent) table so, there is no problem arise to insert the rows in the child table employees.
Now insert another row in the employees table.
INSERT INTO employees VALUES(512,'Kim','Leon','CLERK',18000,80,211);
postgres=# INSERT INTO employees VALUES(512,'Kim','Leon','CLERK',18000,80,211); ERROR: insert or update on table "employees" violates foreign key constraint "employees_department_id_fkey" DETAIL: Key (department_id, manager_id)=(211, 80) is not present in table "departments".
Here in the above, the value against department_id and manager_id combination (211,80) does not matching with the same combination in departments(parent table) table and that is why the child table employees can not contain the combination of values including department_id and manager_id as specified. Here the primary key - foreign key relationship is being violated and shows the above message.
14. Write a sql statement to insert rows into the table employees in which a set of columns department_id and job_id contains the values which must have exists into the table departments and jobs.
Sample table departments. CREATE TABLE departments ( DEPARTMENT_ID integer NOT NULL UNIQUE, DEPARTMENT_NAME varchar(30) NOT NULL, MANAGER_ID integer DEFAULT NULL, LOCATION_ID integer DEFAULT NULL, PRIMARY KEY (DEPARTMENT_ID) ); INSERT INTO departments VALUES(60,'SALES',201,89); INSERT INTO departments VALUES(61,'ACCOUNTS',201,89); postgres=# select * from departments; department_id | department_name | manager_id | location_id ---------------+-----------------+------------+------------- 60 | SALES | 201 | 89 61 | ACCOUNTS | 201 | 89 (2 rows) Sample table jobs. CREATE TABLE jobs ( JOB_ID integer NOT NULL UNIQUE PRIMARY KEY, JOB_TITLE varchar(35) NOT NULL DEFAULT ' ', MIN_SALARY decimal(6,0) DEFAULT 8000, MAX_SALARY decimal(6,0) DEFAULT 20000 ); INSERT INTO jobs(JOB_ID,JOB_TITLE) VALUES(1001,'OFFICER'); INSERT INTO jobs(JOB_ID,JOB_TITLE) VALUES(1002,'CLERK'); postgres=# SELECT * FROM jobs; job_id | job_title | min_salary | max_salary --------+-----------+------------+------------ 1001 | OFFICER | 8000 | 20000 1002 | CLERK | 8000 | 20000 (2 rows) Sample table employees. CREATE TABLE employees ( EMPLOYEE_ID integer NOT NULL PRIMARY KEY, FIRST_NAME varchar(20) DEFAULT NULL, LAST_NAME varchar(25) NOT NULL, DEPARTMENT_ID integer DEFAULT NULL, FOREIGN KEY(DEPARTMENT_ID) REFERENCES departments(DEPARTMENT_ID), JOB_ID integer NOT NULL, FOREIGN KEY(JOB_ID) REFERENCES jobs(JOB_ID), SALARY decimal(8,2) DEFAULT NULL );
Now insert the rows into the table employees.
INSERT INTO employees VALUES(510,'Alex','Hanes',60,1001,18000);
postgres=# SELECT * FROM employees; employee_id | first_name | last_name | department_id | job_id | salary -------------+------------+-----------+---------------+--------+---------- 510 | Alex | Hanes | 60 | 1001 | 18000.00 (1 row)
Here in the above insert statement the child column department_id and job_id of child table employees are successfully referencing with the department_id and job_id column of parent tables departments and jobs respectively, so no problem have been arisen to the insertion.
Now insert another row in the employees table.
INSERT INTO employees VALUES(511,'Tom','Elan',60,1003,22000);
postgres=# INSERT INTO employees VALUES(511,'Tom','Elan',60,1003,22000); ERROR: insert or update on table "employees" violates foreign key constraint "employees_job_id_fkey" DETAIL: Key (job_id)=(1003) is not present in table "jobs".
Here in the above insert statement show that, within child columns department_id and job_id of child table employees, the department_id are successfully referencing with the department_id of parent table departments but job_id column are not successfully referencing with the job_id of parent table jobs, so the problem have been arisen to the insertion displayed an error message.
Now insert another row in the employees table.
INSERT INTO employees VALUES(511,'Tom','Elan',80,1001,22000);
postgres=# INSERT INTO employees VALUES(511,'Tom','Elan',80,1001,22000); ERROR: insert or update on table "employees" violates foreign key constraint "employees_department_id_fkey" DETAIL: Key (department_id)=(80) is not present in table "departments".
Here in the above insert statement show that, within child columns department_id and job_id of child table employees, the job_id are successfully referencing with the job_id of parent table jobs but department_id column are not successfully referencing with the department_id of parent table departments, so the problem have been arisen to the insertion and displayed the error message.
Structure of 'hr' database :