Twitter Bootstrap 3 Button Tutorial
Objective
Buttons are used widely in any website or app. In this tutorial, we will discuss how to use Boostrap 3 button along examples, explanation and how to customize default buttons.
Using default buttons
We will start with examples showing how to create buttons quickly with the host of buttons available out of the box in Bootstrap 3.
<!DOCTYPE html>
<html>
<head>
<title>Default buttons from Bootstrap 3</title>
<meta name="viewport" content="width=device-width, initial-scale=1.0 ">
<!-- Bootstrap -->
<link href="dist/css/bootstrap.min.css" rel="stylesheet" media="screen">
<style>
body {
padding: 50px
}
</style>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/libs/html5shiv/3.7.0/html5shiv.js"></script>
<script src="https://oss.maxcdn.com/libs/respond.js/1.3.0/respond.min.js"></script>
<![endif]-->
</head>
<body>
<!-- Standard button -->
<button type="button" class="btn btn-default">Default</button>
<!-- Provides extra visual weight and identifies the primary action in a set of buttons -->
<button type="button" class="btn btn-primary">Primary</button>
<!-- Indicates a successful or positive action -->
<button type="button" class="btn btn-success">Success</button>
<!-- Contextual button for informational alert messages -->
<button type="button" class="btn btn-info">Info</button>
<!-- Indicates caution should be taken with this action -->
<button type="button" class="btn btn-warning">Warning</button>
<!-- Indicates a dangerous or potentially negative action -->
<button type="button" class="btn btn-danger">Danger</button>
<!-- Deemphasize a button by making it look like a link while maintaining button behavior -->
<button type="button" class="btn btn-link">Link</button>
<!-- jQuery (necessary for Bootstrap's JavaScript plugins) -->
<script src="https://code.jquery.com/jquery.js"></script>
<!-- Include all compiled plugins (below), or include individual files as needed -->
<script src="dist/js/bootstrap.min.js"></script>
</body>
</html>
Help us to serve you with Better content.
The output of the above code looks like following. You may view a live demo here.

CSS Behind
We will dive into the CSS rules used to create these buttons. The primary class used to create buttons is btn which is shown below.
.btn {
display: inline-block;
padding: 6px 12px;
margin-bottom: 0;
font-size: 14px;
font-weight: normal;
line-height: 1.428571429;
text-align: center;
white-space: nowrap;
vertical-align: middle;
cursor: pointer;
background-image: none;
border: 1px solid transparent;
border-radius: 4px;
-webkit-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
-o-user-select: none;
user-select: none;
}
Because of the CSS code above, buttons (with btn class) is displayed as inline-block, which allows buttons to be rendered inline along with elements next to it w.r.t to their common baseline, but you may add width and height properties to it. It has a top and bottom padding of 6 and 12 pixels. Bottom margin is set to zero.
Font size is set to 14 pixels and font's thickness is normal. A button with btn class will be having a line height of 1.428571429, which is slightly more than the default height 1. The text is aligned center.
whitespace property is set to nowrap, so new-lines, spaces, and tabs will be collapsed. Vertically the element associated will be positioned to the middle of the height. When you move your mouse over the associated element, the cursor will be looking like a pointer. There will be no background image rendered.
A transparent, 1-pixel thick solid border will be rendered. Because of the border-radius: 4px;, the corners of the associated button will be rounded. By increasing or decreasing the value 4px, you may reach bigger or smaller roundness. Because of user-select: none, the text of the associated button and sub-elements will appear as if they cannot be selected. For cross-browser consistency, the same property is applied with several vendor prefixes.
Each of the buttons shown is the previous example has another CSS class along with btn, e.g. btn-default, btn-primary etc. These classes are used to set the color, background-color and border properties of the button. They are CSS rules for regular buttons and also for buttons with link states (i.e. hover, active, focus etc.).
btn-primary class's code is follows
.btn-primary {
color: #ffffff;
background-color: #428bca;
border-color: #357ebd;
}
Different sizes of button
There are three sizes can be set out of the box. To set each of the sizes, an additional class needs to be added.
btn-lg for setting large button.
<button type="button" class="btn btn-primary btn-lg">Large button</button>
CSS code for the btn-lg class is as follows
.btn-lg {
padding: 10px 16px;
font-size: 18px;
line-height: 1.33;
border-radius: 6px;
}
btn-sm and btn-xs for setting buttons with other different sizes.
<button type="button" class="btn btn-primary btn-sm">Large button</button>
<button type="button" class="btn btn-primary btn-xs">Large button</button>
CSS code for the btn-sm and btn-xs classes are as follows
.btn-sm,
.btn-xs {
padding: 5px 10px;
font-size: 12px;
line-height: 1.5;
border-radius: 3px;
}
.btn-xs {
padding: 1px 5px;
}
Following code shows different types of buttons with different sizes. You may have a live demo here. We have also presented a screenshot before the code.
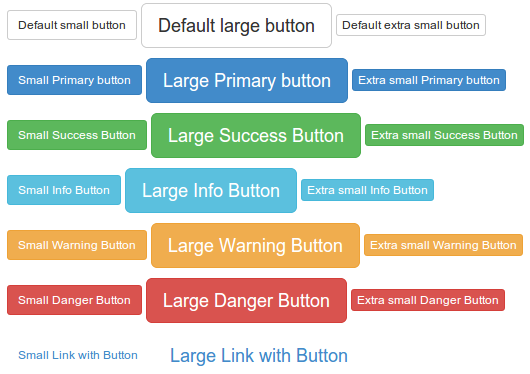
<!DOCTYPE html>
<html>
<head>
<title>Buttons of different sizes from Bootstrap 3</title>
<meta name="viewport" content="width=device-width, initial-scale=1.0 ">
<!-- Bootstrap -->
<link href="dist/css/bootstrap.min.css" rel="stylesheet" media="screen">
<style>
body {
padding: 50px
}
</style>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/libs/html5shiv/3.7.0/html5shiv.js"></script>
<script src="https://oss.maxcdn.com/libs/respond.js/1.3.0/respond.min.js"></script>
<![endif]-->
</head>
<body>
<!-- Standard button -->
<p>
<button type="button" class="btn btn-default btn-sm">Default small button</button>
<button type="button" class="btn btn-default btn-lg">Default large button</button>
<button type="button" class="btn btn-default btn-xs">Default extra small button</button>
</p>
<p>
<!-- Provides extra visual weight and identifies the primary action in a set of buttons -->
<button type="button" class="btn btn-primary btn-sm">Small Primary button</button>
<button type="button" class="btn btn-primary btn-lg">Large Primary button</button>
<button type="button" class="btn btn-primary btn-xs">Extra small Primary button</button>
</p>
<p>
<!-- Indicates a successful or positive action -->
<button type="button" class="btn btn-success btn-sm">Small Success Button</button>
<button type="button" class="btn btn-success btn-lg">Large Success Button</button>
<button type="button" class="btn btn-success btn-xs">Extra small Success Button</button>
</p>
<p>
<!-- Contextual button for informational alert messages -->
<button type="button" class="btn btn-info btn-sm">Small Info Button</button>
<button type="button" class="btn btn-info btn-lg">Large Info Button</button>
<button type="button" class="btn btn-info btn-xs">Extra small Info Button</button>
</p>
<p>
<!-- Indicates caution should be taken with this action -->
<button type="button" class="btn btn-warning btn-sm">Small Warning Button</button>
<button type="button" class="btn btn-warning btn-lg">Large Warning Button</button>
<button type="button" class="btn btn-warning btn-xs">Extra small Warning Button</button>
</p>
<p>
<!-- Indicates a dangerous or potentially negative action -->
<button type="button" class="btn btn-danger btn-sm">Small Danger Button</button>
<button type="button" class="btn btn-danger btn-lg">Large Danger Button</button>
<button type="button" class="btn btn-danger btn-xs">Extra small Danger Button</button>
</p>
<p>
<!-- Deemphasize a button by making it look like a link while maintaining button behavior -->
<button type="button" class="btn btn-link btn-sm">Small Link with Button</button>
<button type="button" class="btn btn-link btn-lg">Large Link with Button</button>
</p>
<!-- jQuery (necessary for Bootstrap's JavaScript plugins) -->
<script src="https://code.jquery.com/jquery.js"></script>
<!-- Include all compiled plugins (below), or include individual files as needed -->
<script src="dist/js/bootstrap.min.js"></script>
</body>
</html>
>block level buttons
To use the full width of the container within which the button is residing, Bootstrap 3 offers block button option. You need to add btn-block class in addition to the classes discussed before to create a block level button.
<button type="button" class="btn btn-primary btn-lg btn-block">Large button</button>
<button type="button" class="btn btn-success btn-lg btn-block">Large button</button>
For block level button, following CSS is defined
.btn-block {
display: block;
width: 100%;
padding-right: 0;
padding-left: 0;
}
.btn-block + .btn-block {
margin-top: 5px;
}
Following shows screenshot, and code for the usage of block level buttons. You may see a live demo here.
<!DOCTYPE html>
<html>
<head>
<title>Block level buttons from Bootstrap 3</title>
<meta name="viewport" content="width=device-width, initial-scale=1.0 ">
<!-- Bootstrap -->
<link href="dist/css/bootstrap.min.css" rel="stylesheet" media="screen">
<style>
body {
padding: 50px
}
</style>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/libs/html5shiv/3.7.0/html5shiv.js"></script>
<script src="https://oss.maxcdn.com/libs/respond.js/1.3.0/respond.min.js"></script>
<![endif]-->
</head>
<body>
<div class="container">
<div class="row">
<div class="col-lg-12">
<!-- Standard button -->
<p>
<button type="button" class="btn btn-primary btn-sm btn-block">Default small block level button</button>
<button type="button" class="btn btn-default btn-lg btn-block">Default large block level button</button>
<button type="button" class="btn btn-primary btn-xs btn-block">Default extra block level button</button>
</p>
<p>
<!-- Provides extra visual weight and identifies the primary action in a set of buttons -->
<button type="button" class="btn btn-primary btn-sm btn-block">Small Primary block level button</button>
<button type="button" class="btn btn-primary btn-lg btn-block">Large Primary block level button</button>
<button type="button" class="btn btn-primary btn-xs btn-block">Extra Large Primary block level button</button>
</p>
<p>
<!-- Indicates a successful or positive action -->
<button type="button" class="btn btn-success btn-sm btn-block">Small Success block level button</button>
<button type="button" class="btn btn-success btn-lg btn-block">Large Success block level button</button>
<button type="button" class="btn btn-success btn-xs btn-block">Extra large Success block level button</button>
</p>
<p>
<!-- Contextual button for informational alert messages -->
<button type="button" class="btn btn-info btn-sm btn-block">Small Info block level button</button>
<button type="button" class="btn btn-info btn-lg btn-block">Large Info block level button</button>
<button type="button" class="btn btn-info btn-xs btn-block">Extra Large Info block level button</button>
</p>
<p>
<!-- Indicates caution should be taken with this action -->
<button type="button" class="btn btn-warning btn-sm btn-block">Small Warning block level button</button>
<button type="button" class="btn btn-warning btn-lg btn-block">Large Warning block level button</button>
<button type="button" class="btn btn-warning btn-xs btn-block">Extra Large Warning block level button</button>
</p>
<p>
<!-- Indicates a dangerous or potentially negative action -->
<button type="button" class="btn btn-danger btn-sm btn-block">Small Danger block level button</button>
<button type="button" class="btn btn-danger btn-lg btn-block">Large Danger block level button</button>
<button type="button" class="btn btn-danger btn-xs btn-block">Extra Large Danger block level button</button>
</p>
<p>
<!-- Deemphasize a button by making it look like a link while maintaining button behavior -->
<button type="button" class="btn btn-link btn-sm btn-block">Small Link with block level button</button>
<button type="button" class="btn btn-link btn-lg btn-block">Large Link with block level button</button>
</p>
</div>
</div>
</div>
<!-- jQuery (necessary for Bootstrap's JavaScript plugins) -->
<script src="https://code.jquery.com/jquery.js"></script>
<!-- Include all compiled plugins (below), or include individual files as needed -->
<script src="dist/js/bootstrap.min.js"></script>
</body>
</html>
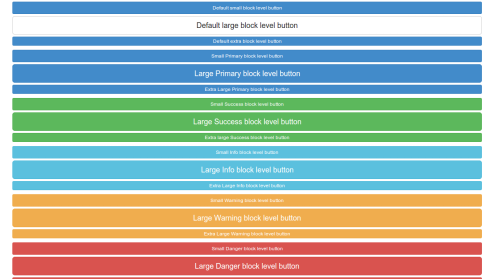
Active Buttons
You may use the active CSS class with buttons as well as links to add different look, to show that it is activated. Here is an example, screenshot and code.

<!DOCTYPE html>
<html>
<head>
<title>Active buttons from Bootstrap 3</title>
<meta name="viewport" content="width=device-width, initial-scale=1.0 ">
<!-- Bootstrap -->
<link href="dist/css/bootstrap.min.css" rel="stylesheet" media="screen">
<style>
body {
padding: 50px
}
</style>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/libs/html5shiv/3.7.0/html5shiv.js"></script>
<script src="https://oss.maxcdn.com/libs/respond.js/1.3.0/respond.min.js"></script>
<![endif]-->
</head>
<body>
<!-- Standard button -->
<button type="button" class="btn btn-default active">Default</button>
<!-- Provides extra visual weight and identifies the primary action in a set of buttons -->
<button type="button" class="btn btn-primary active">Primary</button>
<!-- Indicates a successful or positive action -->
<button type="button" class="btn btn-success active">Success</button>
<!-- Contextual button for informational alert messages -->
<button type="button" class="btn btn-info active">Info</button>
<!-- Indicates caution should be taken with this action -->
<button type="button" class="btn btn-warning active">Warning</button>
<!-- Indicates a dangerous or potentially negative action -->
<button type="button" class="btn btn-danger active">Danger</button>
<!-- Deemphasize a button by making it look like a link while maintaining button behavior -->
<button type="button" class="btn btn-link active">Link</button>
<!-- jQuery (necessary for Bootstrap's JavaScript plugins) -->
<script src="https://code.jquery.com/jquery.js"></script>
<!-- Include all compiled plugins (below), or include individual files as needed -->
<script src="dist/js/bootstrap.min.js"></script>
</body>
</html>
Using Chrome developer Tools, we found what CSS rules are working when Buttons are added with active class
For Default Active Button
.btn-default:hover, .btn-default:focus, .btn-default:active, .btn-default.active, .open .dropdown-toggle.btn-default {
color: #333;
background-color: #ebebeb;
border-color: #adadad;
}
For Primary Active Button
.btn-primary:hover, .btn-primary:focus, .btn-primary:active, .btn-primary.active, .open .dropdown-toggle.btn-primary {
color: #fff;
background-color: #3276b1;
border-color: #285e8e;
}
For Success Active Button
.btn-success:hover, .btn-success:focus, .btn-success:active, .btn-success.active, .open .dropdown-toggle.btn-success { color: #fff; background-color: #47a447; border-color: #398439; }
For Info Active Button
.btn-info:hover, .btn-info:focus, .btn-info:active, .btn-info.active, .open .dropdown-toggle.btn-info {
color: #fff;
background-color: #39b3d7;
border-color: #269abc;
}
For Warning Active Button
.btn-warning:hover, .btn-warning:focus, .btn-warning:active, .btn-warning.active, .open .dropdown-toggle.btn-warning {
color: #fff;
background-color: #ed9c28;
border-color: #d58512;
}
For Danger Active Button
.btn-danger:hover, .btn-danger:focus, .btn-danger:active, .btn-danger.active, .open .dropdown-toggle.btn-danger {
color: #fff;
background-color: #d2322d;
border-color: #ac2925;
}
For Link Active Button
.btn-link, .btn-link:hover, .btn-link:focus, .btn-link:active {
border-color: transparent;
}
So, you can see this is acheived by changing color, backgound-color and border-color properties.
Button disabled
You may use the disabled CSS class with buttons as well as links to add a different look, to show that it is disabled. Here is an example, screenshot, and code.
To make the buttons a bit blurred, the following CSS rule works (found with Chrome Developer Tools)
.btn[disabled], fieldset[disabled] .btn {
pointer-events: none;
cursor: not-allowed;
opacity: .65;
filter: alpha(opacity=65);
-webkit-box-shadow: none;
box-shadow: none;
}

<!DOCTYPE html>
<html>
<head>
<title>Disabled buttons from Bootstrap 3</title>
<meta name="viewport" content="width=device-width, initial-scale=1.0 ">
<!-- Bootstrap -->
<link href="dist/css/bootstrap.min.css" rel="stylesheet" media="screen">
<style>
body {
padding: 50px
}
</style>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/libs/html5shiv/3.7.0/html5shiv.js"></script>
<script src="https://oss.maxcdn.com/libs/respond.js/1.3.0/respond.min.js"></script>
<![endif]-->
</head>
<body>
<!-- Standard button -->
<button type="button" class="btn btn-default" disabled="disabled">Default</button>
<!-- Provides extra visual weight and identifies the primary action in a set of buttons -->
<button type="button" class="btn btn-primary" disabled="disabled">Primary</button>
<!-- Indicates a successful or positive action -->
<button type="button" class="btn btn-success" disabled="disabled">Success</button>
<!-- Contextual button for informational alert messages -->
<button type="button" class="btn btn-info" disabled="disabled">Info</button>
<!-- Indicates caution should be taken with this action -->
<button type="button" class="btn btn-warning" disabled="disabled">Warning</button>
<!-- Indicates a dangerous or potentially negative action -->
<button type="button" class="btn btn-danger" disabled="disabled">Danger</button>
<!-- jQuery (necessary for Bootstrap's JavaScript plugins) -->
<script src="https://code.jquery.com/jquery.js"></script>
<!-- Include all compiled plugins (below), or include individual files as needed -->
<script src="dist/js/bootstrap.min.js"></script>
</body>
</html>
Anchor elements disabled
When anchor elements with btn and other related classes are represented as disabled, it works a bit differently. Firstly, the link of the anchor elements are still exists, only the look and feel is changed. Moreover, you can not use them in navbar.
A live demo and code follow.
<!DOCTYPE html>
<html>
<head>
<title>Disabled anchor buttons from Bootstrap 3</title>
<meta name="viewport" content="width=device-width, initial-scale=1.0 ">
<!-- Bootstrap -->
<link href="dist/css/bootstrap.min.css" rel="stylesheet" media="screen">
<style>
body {
padding: 50px
}
</style>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/libs/html5shiv/3.7.0/html5shiv.js"></script>
<script src="https://oss.maxcdn.com/libs/respond.js/1.3.0/respond.min.js"></script>
<![endif]-->
</head>
<body>
<!-- Standard button -->
<a href="http://www.example.com" class="btn btn-default disabled" role="button">Default</a>
<!-- Provides extra visual weight and identifies the primary action in a set of buttons -->
<a href="http://www.example.com" class="btn btn-primary disabled" role="button">Primary</a>
<!-- Indicates a successful or positive action -->
<a href="http://www.example.com" class="btn btn-success disabled" role="button">Success</a>
<!-- Contextual button for informational alert messages -->
<a href="http://www.example.com" class="btn btn-info disabled" role="button">Info</a>
<!-- Indicates caution should be taken with this action -->
<a href="http://www.example.com" class="btn btn-warning disabled" role="button">Warning</a>
<!-- Indicates a dangerous or potentially negative action -->
<a href="http://www.example.com" class="btn btn-danger disabled" role="button">Danger</a>
<!-- jQuery (necessary for Bootstrap's JavaScript plugins) -->
<script src="https://code.jquery.com/jquery.js"></script>
<!-- Include all compiled plugins (below), or include individual files as needed -->
<script src="dist/js/bootstrap.min.js"></script>
</body>
</html>
Note that role="button" is used for accessibility purpose.
Button tags
You may use button classes with button, a and input element to use buttons as tags. But it is recommended that you use it with button elements mostly. Otherwise, it may cause cross-browser inconsistency issues.
Following example shows how to use button tags
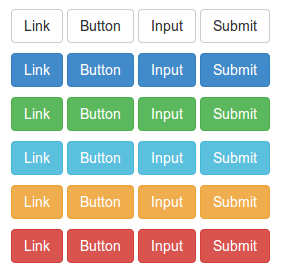
<!DOCTYPE html>
<html>
<head>
<title>Button tags example with Bootstrap 3</title>
<meta name="viewport" content="width=device-width, initial-scale=1.0 ">
<!-- Bootstrap -->
<link href="dist/css/bootstrap.min.css" rel="stylesheet" media="screen">
<style>
body {
padding: 50px
}
</style>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/libs/html5shiv/3.7.0/html5shiv.js"></script>
<script src="https://oss.maxcdn.com/libs/respond.js/1.3.0/respond.min.js"></script>
<![endif]-->
</head>
<body>
<p>
<a class="btn btn-default" href="#" role="button">Link</a>
<button class="btn btn-default" type="submit">Button</button>
<input class="btn btn-default" type="button" value="Input">
<input class="btn btn-default" type="submit" value="Submit">
</p>
<p>
<a class="btn btn-primary" href="#" role="button">Link</a>
<button class="btn btn-primary" type="submit">Button</button>
<input class="btn btn-primary" type="button" value="Input">
<input class="btn btn-primary" type="submit" value="Submit">
</p>
<p>
<a class="btn btn-success" href="#" role="button">Link</a>
<button class="btn btn-success" type="submit">Button</button>
<input class="btn btn-success" type="button" value="Input">
<input class="btn btn-success" type="submit" value="Submit">
</p>
<p>
<a class="btn btn-info" href="#" role="button">Link</a>
<button class="btn btn-info" type="submit">Button</button>
<input class="btn btn-info" type="button" value="Input">
<input class="btn btn-info" type="submit" value="Submit">
</p>
<p>
<a class="btn btn-warning" href="#" role="button">Link</a>
<button class="btn btn-warning" type="submit">Button</button>
<input class="btn btn-warning" type="button" value="Input">
<input class="btn btn-warning" type="submit" value="Submit">
</p>
<p>
<a class="btn btn-danger" href="#" role="button">Link</a>
<button class="btn btn-danger" type="submit">Button</button>
<input class="btn btn-danger" type="button" value="Input">
<input class="btn btn-danger" type="submit" value="Submit">
</p>
<!-- jQuery (necessary for Bootstrap's JavaScript plugins) -->
<script src="https://code.jquery.com/jquery.js"></script>
<!-- Include all compiled plugins (below), or include individual files as needed -->
<script src="dist/js/bootstrap.min.js"></script>
</body>
</html>
A live demo of the above example
itemscope itemtype="http://schema.org/WebPageElement/Heading">Customize Buttons
Now we will start with default button and customize it to give it a whole different look and feel.
Button code we will start with is as follows
<button type="button" class="btn btn-default">Default</button>
Now we will create a CSS class btn-w3r and replace it with btn-default. So, instead of the previous code, we will write
<button type="button" class="btn btn-w3r">Default</button>
We will change the background color first, by adding the following CSS
.btn-w3r {
background: #cb60b3; /* Old browsers */
background: -moz-linear-gradient(top, #cb60b3 0%, #ad1283 50%, #de47ac 100%); /* FF3.6+ */
background: -webkit-gradient(linear, left top, left bottom, color-stop(0%,#cb60b3), color-stop(50%,#ad1283), color-stop(100%,#de47ac)); / * Chrome,Safari4+ */
background: -webkit-linear-gradient(top, #cb60b3 0%,#ad1283 50%,#de47ac 100%); /* Chrome10+,Safari5.1+ */
background: -o-linear-gradient(top, #cb60b3 0%,#ad1283 50%,#de47ac 100%); /* Opera 11.10+ */
background: -ms-linear-gradient(top, #cb60b3 0%,#ad1283 50%,#de47ac 100%); /* IE10+ */
background: linear-gradient(to bottom, #cb60b3 0%,#ad1283 50%,#de47ac 100%); /* W3C */
filter: progid:DXImageTransform.Microsoft.gradient( startColorstr='#cb60b3', endColorstr='#de47ac',GradientType=0 ); /* IE6-9 */
}
The button looks like following now
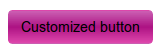
To change the font color, we will append the following CSS to the existing btn-w3r class
color:#fff;
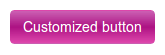
Now, to shape the button like circle, we will prepend the following CSS code to the existing CSS code of btn-w3r
width: 200px;
height: 200px;
-moz-border-radius: 50%;
-webkit-border-radius: 50%;
border-radius: 50%;
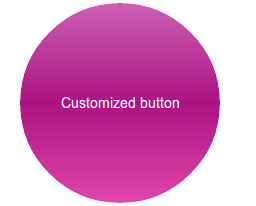
To add a hover effect, we will add the following CSS code
.btn-w3r:hover {
background: #333;
color:#e75616;
}
This is how it looks(on hover) after adding that CSS Code
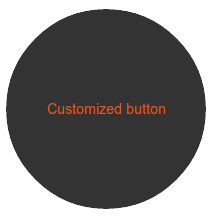
You can see a live demo of the customized button we have created here.
You may download the code of this button from here.
Questions? Suggestions? Join the discussion below. For the upcoming tutorials, please subscribe to our Feed.
Previous: Twitter Bootstrap Forms tutorial
Next:
Twitter Bootstrap 3 Images Tutorial
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics