HTML5 Canvas : Adding Shadows
Shadows
In HTML5 canvas, you can add shadows on a shape, a line, text, or an image which can create a sense of third dimension. To add shadows with the HTML5 Canvas, you can use the following properties of the canvas context.
- shadowOffsetX
- shadowOffsetY
- shadowColor
- shadowBlur
shadowOffsetX() Property
The property is used to get or set the horizontal distance of a shadow from a shape. You can use positive or negative values to control the position of a shadow. The default value is zero.
Syntax:
ctx.shadowOffsetX = h_distance;
Where h_distance (type: number) is the horizontal distance of a shadow from a shape.
shadowOffsetY() Property
The property is used to get or set the vertical distance of a shadow from a shape. You can use positive or negative values to control the position of a shadow. The default value is zero.
Syntax:
ctx.shadowOffsetX = v_distance;
Where v_distance (type: number) is the vertical distance of a shadow from a shape.
shadowColor() Property
The property is used to get or set the color to use for shadows.
Syntax:
ctx.shadowColor
Where shadowColor (type: string) is the CSS color.
shadowBlur() Property
The property is used to get or set the current level of blur that is applied to shadows.
Syntax:
ctx.shadowBlur = blur_value
Where blur_value is the amount of blur (type: number) that is applied to shadows.
Example: HTML5 Canvas adding shadow
The following web document creates a series of squares that have different degrees of shadow blur.
Output:
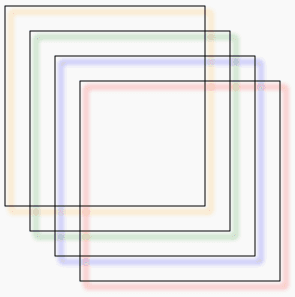
Code:
<!DOCTYPE html>
<html>
<head><title>HTML5 Canvas - shadow</title>
</head>
<body>
<canvas id="DemoCanvas" width="500" height="600"></canvas>
<script>
var canvas = document.getElementById("DemoCanvas");
if (canvas.getContext)
{
var ctx = canvas.getContext('2d');
ctx.shadowColor = "black";
ctx.shadowBlur = 6;
ctx.shadowOffsetX = 6;
ctx.shadowOffsetY = 6;
ctx.shadowColor = "orange";
ctx.strokeRect(25, 25, 200, 200);
ctx.shadowColor = "green";
ctx.strokeRect(50, 50, 200, 200);
ctx.shadowColor = "blue";
ctx.strokeRect(75, 75, 200, 200);
ctx.shadowColor = "red";
ctx.strokeRect(100, 100, 200, 200);
}
</script>
</body>
</html>
Here is an another example :
Output:
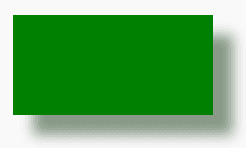
Code:
<!DOCTYPE html>
<html>
<head><title>HTML5 Canvas - shadow</title>
</head>
<body>
<canvas id="DemoCanvas" width="500" height="600"></canvas>
<script>
var canvas = document.getElementById("DemoCanvas");
if (canvas.getContext)
{
var ctx = canvas.getContext('2d');
ctx.rect(100, 40, 200, 100);
ctx.fillStyle = 'green';
ctx.shadowColor = '#898';
ctx.shadowBlur = 20;
ctx.shadowOffsetX = 20;
ctx.shadowOffsetY = 20;
ctx.fill();
}
</script>
</body>
</html>
Example : HTML5 Canvas adding shadow on Text
The following web document creates shadow on text.
Output:
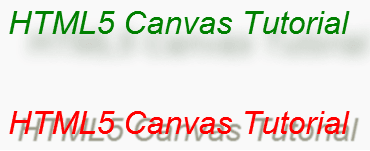
Code :
<!DOCTYPE html>
<html>
<head><title>HTML5 Canvas - shadow</title>
</head>
<body>
<canvas id="DemoCanvas" width="500" height="600"></canvas>
<script>
var canvas = document.getElementById("DemoCanvas");
if (canvas.getContext)
{
var ctx = canvas.getContext('2d');
ctx.fillStyle = 'green';
ctx.shadowColor = '#898';
ctx.shadowBlur = 20;
ctx.shadowOffsetX = 20;
ctx.shadowOffsetY = 20;
//ctx.fill();
ctx.font = 'italic 32px sans-serif';
ctx.fillText('HTML5 Canvas Tutorial', 10, 50);
ctx.fillStyle = 'red';
ctx.shadowColor = '#998';
ctx.shadowBlur = 1;
ctx.shadowOffsetX = 9;
ctx.shadowOffsetY = 7;
//ctx.fill();
ctx.font = 'italic 32px sans-serif';
ctx.fillText('HTML5 Canvas Tutorial', 10, 150);
}
</script>
</body>
</html>
Code Editor:
See the Pen html css common editor by w3resource (@w3resource) on CodePen.
Previous: HTML5 Canvas Text
Next:
HTML5 Canvas translation, scaling and rotation tutorial