HTML5 Canvas path tutorial
Introduction
The canvas paths allow you to draw custom shapes. In HTML5 canvas, a path consists of a list of zero or more subpaths. There are one or more points in each subpath that are connected by straight lines or curves. To create a custom shape perform the following steps :
- Use beginPath() method to start drawing the path.
- Draw the path that makes a shape using lines, curves and other primitives.
- After creating the path, call fill() method to fills subpaths by using the current fill style or stroke() method to render the strokes of the current subpath by using the current stroke styles. Your shape will not be visible until you call fill() or stroke() methods.
- Now call closePath() method to close the current subpath and starts a new subpath that has a start point that is equal to the end of the closed subpath.
Note : If a subpath has fewer than two points, it is ignored when the path is painted.
HTML5 Canvas Path : Example - 1
The following web document uses the beginPath() method to draw two paths by using different colors. At the end it uses closePath() method to close the current subpath.
Output:
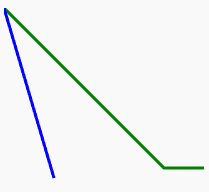
Code:
<!DOCTYPE html>
<html>
<head>
<title>Sample arcs example</title>
</head>
<body>
<canvas id="myCanvas" width="300" height="600"></canvas>
<script>
var canvas = document.getElementById("myCanvas");
if (canvas.getContext)
{
var ctx = canvas.getContext('2d');
ctx.beginPath(); // Start a new path.
ctx.lineWidth = "3";
ctx.strokeStyle = "green"; // This path is green.
ctx.moveTo(0, 0);
ctx.lineTo(160, 160);
ctx.lineTo(200, 160);
ctx.stroke();
ctx.beginPath();
ctx.strokeStyle = "blue"; // This path is blue.
ctx.moveTo(0, 0);
ctx.lineTo(50, 170);
ctx.stroke();
ctx.closePath(); // Close the current path.
}
</script>
</body>
</html>
HTML5 Canvas Path Example - 2 : Draw a Triangle
The following web document draws a triangle.
Output :
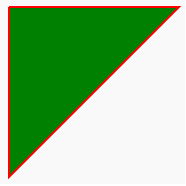
Code:
<!DOCTYPE html>
<html>
<head>
<title>Sample arcs example</title>
</head>
<body>
<canvas id="DemoCanvas" width="300" height="600"></canvas>
<script>
var canvas = document.getElementById("DemoCanvas");
var context = canvas.getContext("2d");
// Set the style properties.
context.fillStyle = 'green';
context.strokeStyle = 'red';
context.lineWidth = 2;
context.beginPath();
// Start from the top-left point.
context.moveTo(20, 20); // give the (x,y) coordinates
context.lineTo(190, 20);
context.lineTo(20, 190);
context.lineTo(20, 20);
// Now fill the shape, and draw the stroke.
context.fill();
context.stroke();
context.closePath();
</script>
</body>
</html>
HTML5 Canvas Path : Example - 3
The following path diagram has created by a quadratic curve and a straight line.
Output :
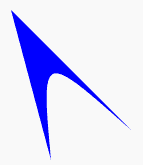
Code :
<!DOCTYPE html>
<html>
<head>
<title>Sample arcs example</title>
</head>
<body>
<canvas id="DemoCanvas" width="300" height="600"></canvas>
<script>
var ctx = document.getElementById('DemoCanvas').getContext('2d');
ctx.fillStyle = "blue";
ctx.beginPath();
ctx.moveTo(30, 30);
ctx.lineTo(150, 150);
ctx.bezierCurveTo(60, 70, 60, 70, 70, 180);
ctx.lineTo(30, 30);
ctx.fill();
</script>
</body>
</html>
HTML5 Canvas Path : Example - 4
The following path diagram has created by a straight line (red color), a quadratic curve (blue color) and a bezier curve (green color).
Output:
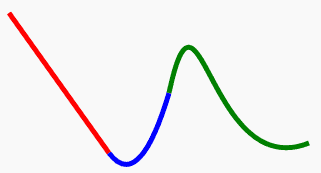
Code:
<!DOCTYPE html>
<html>
<head>
<title>Sample arcs example</title>
</head>
<body>
<canvas id="DemoCanvas" width="500" height="600"></canvas>
<script>
var canvas = document.getElementById('DemoCanvas');
if (canvas.getContext)
{
var ctx = canvas.getContext('2d');
ctx.lineWidth = 5;
ctx.beginPath();
ctx.moveTo(100, 20);
// line 1
ctx.lineTo(200, 160);
ctx.strokeStyle = 'red';
ctx.stroke();
// quadratic curve
ctx.beginPath();
ctx.moveTo(200,160);
ctx.quadraticCurveTo(230, 200, 260, 100);
ctx.strokeStyle = 'blue';
ctx.stroke();
// bezier curve
ctx.beginPath();
ctx.moveTo(260,100);
ctx.bezierCurveTo(290, -40, 300, 190, 400, 150);
ctx.strokeStyle = 'green';
ctx.stroke();
ctx.closePath();
}
</script>
</body>
</html>
See the Pen html css common editor by w3resource (@w3resource) on CodePen.
Previous: HTML5 Canvas arcs tutorial
Next:
HTML5 Canvas Rectangle