HTML5 Canvas Text
Draw Text
In HTML5, canvas element supports basic text rendering on a line-by-line basis. There are two methods fillText() and strokeText() to draw text on canvas. You can use font property (type : string) to specify a number of text setting such as style, weight, size, and font. The style can be normal, italic, or bold. Default style is normal.
Code example of font property :
ctx.font = 'italic 400 12px, sans-serif';
The "400" font-weight doesn't appear because that is the default value.
fillText() Method
The fillText() method is used to render filled text to the canvas by using the current fill style and font.
Syntax:
ctx.fillText(text, x, y, maxWidth)
Parameters | Type | Description |
---|---|---|
text |
string | The text characters to paint on the canvas. |
x | number | The horizontal coordinate to start painting the text, relative to the canvas. |
y | number | The vertical coordinate to start painting the text, relative to the canvas. |
maxWidth | number | The maximum possible text width. |
Example : HTML5 Canvas draw text using fillText() Method
The following web document draws text using fillText() method and font property.
Output:

Code :
<!DOCTYPE html>
<html>
<head>
<title>HTML5 Canvas - Text</title>
</head>
<body>
<canvas id="DemoCanvas" width="500" height="600"></canvas>
<script>
var canvas = document.getElementById("DemoCanvas");
if (canvas.getContext)
{
var ctx = canvas.getContext('2d');
ctx.font = 'italic 32px sans-serif';
ctx.fillText('HTML5 Canvas Tutorial', 10, 50);
}
</script>
</body>
</html>
strokeText() Method
The strokeText() method is used to render the specified text at the specified position by using the current font, lineWidth, and strokeStyle property.
Syntax:
ctx.strokeText(text, x, y, maxWidth);
Parameters | Type | Description |
---|---|---|
text | string | The text characters to paint on the canvas. |
x | number | The horizontal coordinate to start painting the text, relative to the canvas. |
y | number | The vertical coordinate to start painting the text, relative to the canvas. |
maxWidth | number | The maximum possible text width. |
Example: HTML5 Canvas draw text using strokeText() Method
The following web document draws text using strokeText() method and font, lineWidth properties.
Output:
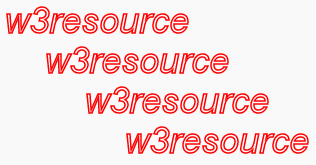
Code :
<!DOCTYPE html>
<html>
<head>
<title>HTML5 Canvas - Text</title>
</head>
<body>
<canvas id="DemoCanvas" width="500" height="600"></canvas>
<script>
var canvas = document.getElementById("DemoCanvas");
if (canvas.getContext)
{
var ctx = canvas.getContext('2d');
ctx.font = "italic 36px Unknown Font, sans-serif";
ctx.strokeStyle = "red"; // set stroke color to red
ctx.lineWidth = "1.5"; // set stroke width to 1.5
for (var i = 40; i < 200; i += 40)
{
ctx.strokeText("w3resource", i, i);
}
}
</script>
</body>
</html>
Example : Draw text using fillText() method and linear gradient
The following web document draws text that is filled with a linear gradient.
Output:
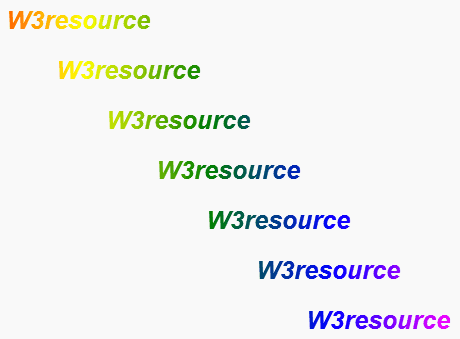
Code:
<!DOCTYPE html>
<html>
<head>
<title>HTML5 Canvas - Text</title>
</head>
<body>
<canvas id="DemoCanvas" width="500" height="600"></canvas>
<script>
var canvas = document.getElementById("DemoCanvas");
if (canvas.getContext)
{
var ctx = canvas.getContext('2d');
gradient = ctx.createLinearGradient(0, 0, canvas.width, 0);
// Add the colors with fixed stops at 25% of the width.
gradient.addColorStop("0", "red");
gradient.addColorStop(".25", "yellow");
gradient.addColorStop(".50", "green");
gradient.addColorStop(".75", "blue");
gradient.addColorStop("1.0", "magenta");
// Use the gradient.
ctx.font = "italic 700 25px Unknown Font, sans-serif";
ctx.fillStyle = gradient;
for (var i = 0; i < 400; i += 50)
{
ctx.fillText("W3resource", i, i);
}
}
</script>
</body>
</html>
Text Alignment
In HTML5 canvas textAlign property is used to set the text alignment on the canvas. Possible values are start, end, left, right, and center. Default value : start.
- start : The text is aligned at the normal start of the line (left-aligned for left-to-right locales, right-aligned for right-to-left locales).
- end : The text is aligned at the normal end of the line (right-aligned for left-to-right locales, left-aligned for right-to-left locales).
- left : The text is left-aligned.
- right : The text is right-aligned.
- center : The text is centered.
Syntax :
ctx.textAlign = value
Example: HTML5 Canvas, draw text using text alignment
The following code example shows all the property values of textAlign property.
Output :
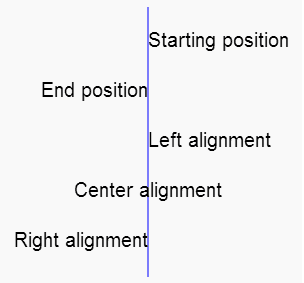
Code:
<!DOCTYPE html>
<html>
<head>
<title>HTML5 Canvas - Text</title>
</head>
<body>
<canvas id="DemoCanvas" width="500" height="600"></canvas>
<script>
var canvas = document.getElementById("DemoCanvas");
if (canvas.getContext)
{
var ctx = canvas.getContext('2d');
// Create a line at the anchor point.
ctx.strokeStyle = "blue";
ctx.textAlign = "center";
ctx.moveTo(150, 60);
ctx.lineTo(150, 330);
ctx.stroke();
ctx.strokeStyle = "green";
ctx.font = "20px sans-serif";
ctx.textAlign = "start";
ctx.fillText("Starting position", 150, 100);
ctx.textAlign = "end";
ctx.fillText("End position", 150, 150);
ctx.textAlign = "left";
ctx.fillText("Left alignment", 150, 200);
ctx.textAlign = "center";
ctx.fillText("Center alignment", 150, 250);
ctx.textAlign = "right";
ctx.fillText("Right alignment", 150, 300);
}
</script>
</body>
</html>
Text Baseline
In HTML5 canvas textBaseline property is used to get or set the current settings for the font baseline alignment. Legal values are top, hanging, middle, alphabetic, ideographic, bottom.
- top : The top of the em square.
- hanging : The hanging baseline
- middle : The middle of the em square.
- alphabetic : Default. The alphabetic baseline.
- ideographic : The ideographic baseline.
- bottom : The bottom of the em square.
Syntax:
ctx.textBaseline = value
The position of each baseline value, relative to the bounding box, is shown in the following picture :
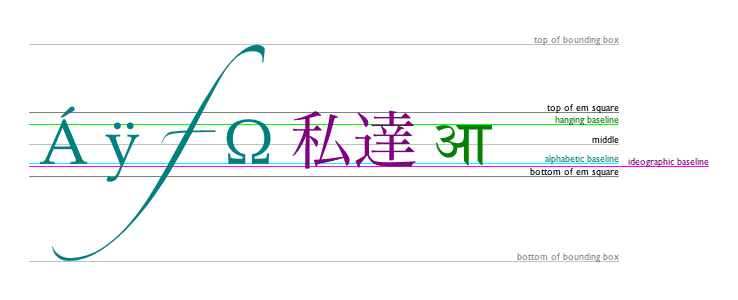
Example : HTML5 Canvas, text baseline example
In the following example the text is vertically centered (textBaseline = 'middle) in the bounding box.
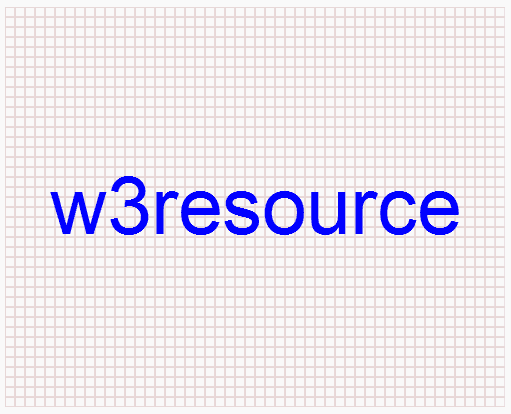
Code:
<!DOCTYPE html>
<html>
<head>
<title>HTML5 Canvas - Text</title>
</head>
<body>
<canvas id="DemoCanvas" width="500" height="400"></canvas>
<script>
var canvas = document.getElementById("DemoCanvas");
if (canvas.getContext)
{
var ctx = canvas.getContext('2d');
for (i = 0; i < 500; i += 10)
{
ctx.moveTo(0, i);
ctx.strokeStyle = "#E8D8D8";
ctx.lineTo(canvas.width, i);
ctx.stroke();
}
for (i = 0; i <511; i += 10)
{
ctx.moveTo(i, 0);
ctx.strokeStyle = "#E8D8D8";
ctx.lineTo(i,canvas.height);
ctx.stroke();
}
ctx.beginPath();
ctx.moveTo(0, 300);
ctx.font = "80px Unknown Font, sans-serif";
var x = canvas.width / 2;
var y = canvas.height / 2;
ctx.textAlign = 'center';
ctx.textBaseline = 'middle';
ctx.fillStyle = 'blue';
ctx.fillText('w3resource', x, y);
}
</script>
</body>
</html>
Text Metrics
In HTML5 canvas measureText() method is used to get the text metrics of HTML5 Canvas text. The method returns an object that contains the width (in pixels) of the specified text.
Syntax:
var textWidth = ctx.measureText(text)
Example : HTML5 Canvas, text metrics example
The following example returns the width (in pixels) of a given text.
<!DOCTYPE html>
<html>
<head>
<title>HTML5 Canvas - Text</title>
</head>
<body>
<canvas id="DemoCanvas" width="500" height="600"></canvas>
<script>
var canvas = document.getElementById("DemoCanvas");
if (canvas.getContext)
{
var ctx = canvas.getContext('2d');
var text='w3resource';
ctx.font = "24px Unknown Font, sans-serif";
ctx.fillText(text, 120, 40);
var metrics=ctx.measureText(text);
alert(metrics.width);
}
</script>
</body>
</html>
Code Editor:
See the Pen html css common editor by w3resource (@w3resource) on CodePen.
Previous: HTML5 Canvas: Gradients and Patterns
Next:
HTML5 Canvas : Adding Shadows
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/html5-canvas/html5-canvas-text.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics