JavaScript: HTML Form validation - checking for Floating point numbers
Checking for Floating point numbers
A floating number has the following parts.
- A decimal integer.
- A decimal point ('.').
- A fraction.
- An exponent (optional).
The exponent part is an "e" or "E" followed by an integer, which can be signed (preceded by "+" or "-").
Example of some floating numbers:
- 7.2935
- -12.72
- 1/2
- 12.4e3 [ Equivalent to 12.4 x 103 ]
- 4E-3 [ Equivalent to 4 x 10-3 ]
Sometimes it is required to input a number with decimal part particularly for quantity, length, height, amounts etc. We can use a floating point number in various ways, here are some examples.
- No exponent, mandatory integer and fraction and optional sign.
- No exponent, mandatory sign (+-), integer, and a fraction.
- Mandatory sign (+-), exponent, integer, fraction.
To check the said format we have used the various regular expression. Following code blocks contain actual codes and we have kept the CSS code part common for all the validations.
CSS Code
li {list-style-type: none;
font-size: 16pt;
}
.mail {
margin: auto;
padding-top: 10px;
padding-bottom: 10px;
width: 400px;
background : #D8F1F8;
border: 1px soild silver;
}
.mail h2 {
margin-left: 38px;
}
input {
font-size: 20pt;
}
input:focus, textarea:focus{
background-color: lightyellow;
}
input submit {
font-size: 12pt;
}
.rq {
color: #FF0000;
font-size: 10pt;
}
Javascript function to check whether a field input contains a number with no exponent, mandatory integer and fraction and optional sign
To get a string contains a number with no exponent, mandatory integer and fraction and optional sign we use a regular expression /^[-+]?[0-9]+\.[0-9]+$/ which allows the said format. Next, the match() method of a string object is used to match the said regular expression against the input value. Here is the complete web document.
HTML Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>JavaScript form validation - check a Floating Number</title>
<link rel='stylesheet' href='form-style.css' type='text/css' />
</head>
<body onload='document.form1.text1.focus()'>
<div class="mail">
<h2>Check whether the field input contains a number with no exponent, mandatory integer and fraction and optional sign</h2>
<form name="form1" action="#">
<ul>
<li><input type='text' name='text1'/></li>
<li> </li>
<li><input type="submit" name="submit" value="Submit" onclick="CheckDecimal(document.form1.text1)"/></li>
<li> </li>
</ul>
</form>
</div>
<script src="check-decimal.js"></script>
</body>
</html>
JavaScript Code
function CheckDecimal(inputtxt)
{
var decimal= /^[-+]?[0-9]+\.[0-9]+$/;
if(inputtxt.value.match(decimal))
{
alert('Correct, try another...')
return true;
}
else
{
alert('Wrong...!')
return false;
}
}
Flowchart :
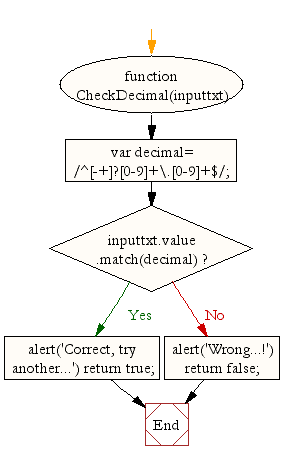
View the example in the browser
Javascript function to check whether a field input contains a number with no exponent, mandatory sign (+-), integer, and fraction
To get a string contains a number with no exponent, mandatory integer, fraction, sign (+ -) we use a regular expression /[-+][0-9]+\.[0-9]+$/ which allows the said format. Next, the match() method of a string object is used to match the said regular expression against the input value. Here is the complete web document.
HTML Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>JavaScript form validation - check a Floating Number starting with sign</title>
<link rel='stylesheet' href='form-style.css' type='text/css' />
</head>
<body onload='document.form1.text1.focus()'>
<div class="mail">
<h2>Check whether a field input contains a number with no exponent, mandatory sign (+-), integer, and fraction</h2>
<form name="form1" action="#">
<ul>
<li><input type='text' name='text1'/></li>
<li> </li>
<li><input type="submit" name="submit" value="Submit" onclick="CheckDecimal(document.form1.text1)"/></li>
<li> </li>
</ul>
</form>
</div>
<script src="check-decimal-with-sign.js"></script>
</body>
</html>
JavaScript Code
function CheckDecimal(inputtxt)
{
var decimal= /[-+][0-9]+\.[0-9]+$/;
if(inputtxt.value.match(decimal))
{
alert('Correct, try another...')
return true;
}
else
{
alert('Wrong...!')
return false;
}
}
Flowchart:
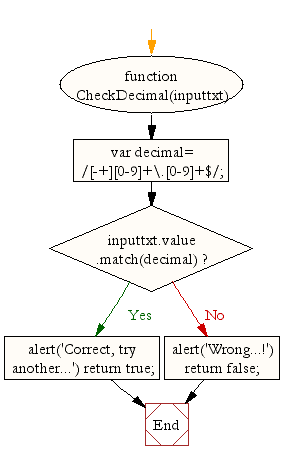
View the example in the browser
Javascript function to check whether a field input contains a number starting with mandatory sign (+-), exponent, integer, fraction
To get a string contains a number with a mandatory sign (+ -), exponent integer, fraction, a sign we use a regular expression /^[-+][0-9]+\.[0-9]+[eE][-+]?[0-9]+$/ which allows the said format. Next, the match() method of a string object is used to match the said regular expression against the input value. Here is the complete web document.
HTML Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>JavaScript form validation - check a Floating Number with exponent and starting with sign </title>
<link rel='stylesheet' href='form-style.css' type='text/css' />
</head>
<body onload='document.form1.text1.focus()'>
<div class="mail"><h2>Check whether a field input contains a number starting with mandatory sign (+-), exponent, integer, fraction</h2>
<form name="form1" action="#">
<ul>
<li><input type='text' name='text1'/></li>
<li> </li>
<li><input type="submit" name="submit" value="Submit" onclick="CheckDecimal(document.form1.text1)"/></li>
<li> </li>
</ul>
</form>
</div>
<script src="check-decimal-with-exponent-sign.js"></script>
</body>
</html>
JavaScript Code
function CheckDecimal(inputtxt)
var decimal= /^[-+][0-9]+\.[0-9]+[eE][-+]?[0-9]+$/;
if(inputtxt.value.match(decimal))
{
alert('Correct, try another...')
return true;
}
else
{
alert('Wrong...!')
return false;
}
}
Flowchart:
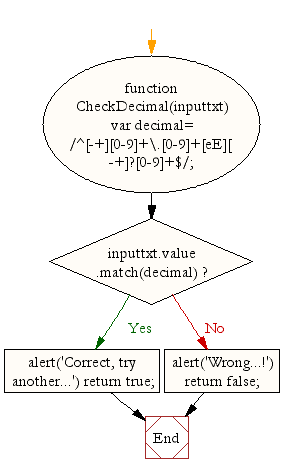
View the example in the browser
Other JavaScript Validation :
- Checking for non-empty
- Checking for all letters
- Checking for all numbers
- Checking for floating numbers
- Checking for letters and numbers
- Checking string length
- Email Validation
- Date Validation
- A sample Registration Form
- Phone No. Validation
- Credit Card No. Validation
- Password Validation
- IP address Validation
Previous: JavaScript: HTML Form validation - checking for all numbers
Next: JavaScript: HTML Form - checking for numbers and letters
Test your Programming skills with w3resource's quiz.