JavaScript: HTML Form validation - checking for all letters
Checking for all letters
Sometimes situations arise when a user should fill a single or more than one fields with alphabet characters (A-Z or a-z) in an HTML form. You can write a JavaScript form validation script to check whether the required field(s) in the HTML form contains only letters.
Javascript function to check for all letters in a field
function allLetter(inputtxt)
{
var letters = /^[A-Za-z]+$/;
if(inputtxt.value.match(letters))
{
return true;
}
else
{
alert("message");
return false;
}
}
To get a string contains only letters (both uppercase or lowercase) we use a regular expression (/^[A-Za-z]+$/) which allows only letters. Next the match() method of string object is used to match the said regular expression against the input value. Here is the complete web document.
Flowchart:
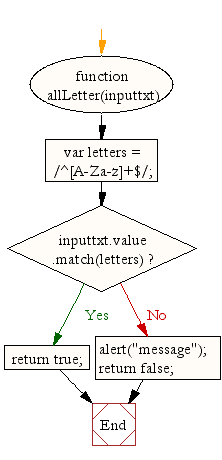
HTML Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta http-equiv="content-type" content="text/html; charset=utf-8" />
<title>JavaScript form validation - checking all letters</title>
<link rel='stylesheet' href='form-style.css' type='text/css' />
</head>
<body onload='document.form1.text1.focus()'>
<div class="mail">
<h2>Enter your Name and Submit</h2>
<form name="form1" action="#">
<ul>
<li>Code:</li>
<li><input type='text' name='text1'/></li>
<li class="rq">*Enter alphabets only.</li>
<li> </li>
<li><input type="submit" name="submit" value="Submit" onclick="allLetter(document.form1.text1)" /></li>
<li> </li>
</ul>
</form>
</div>
<script src="all-letter.js"> </script>
</body>
</html>
JavaScript Code
function allLetter(inputtxt)
{
var letters = /^[A-Za-z]+$/;
if(inputtxt.value.match(letters))
{
alert('Your name have accepted : you can try another');
return true;
}
else
{
alert('Please input alphabet characters only');
return false;
}
}
CSS Code
li {list-style-type: none;
font-size: 16pt;
}
.mail {
margin: auto;
padding-top: 10px;
padding-bottom: 10px;
width: 400px;
background : #D8F1F8;
border: 1px solid silver;
}
.mail h2 {
margin-left: 38px;
}
input {
font-size: 20pt;
}
input:focus, textarea:focus{
background-color: lightyellow;
}
input submit {
font-size: 12pt;
}
.rq {
color: #FF0000;
font-size: 10pt;
}
View the example in the browser
Practice the example online
See the Pen all-letters-field-1 by w3resource (@w3resource) on CodePen.
file_download Download the validation code from here.
Other JavaScript Validation :
- Checking for non-empty
- Checking for all letters
- Checking for all numbers
- Checking for floating numbers
- Checking for letters and numbers
- Checking string length
- Email Validation
- Date Validation
- A sample Registration Form
- Phone No. Validation
- Credit Card No. Validation
- Password Validation
- IP address Validation
Previous: JavaScript: HTML Form - checking for non empty field
Next: JavaScript: HTML Form validation - checking for all numbers
Test your Programming skills with w3resource's quiz.