JavaScript: HTML Form - IP address validation
IP address validation
Every computer connected to the Internet is identified by a unique four-part string, known as its Internet Protocol (IP) address. An IP address consists of four numbers (each between 0 and 255) separated by periods. The format of an IP address is a 32-bit numeric address written as four decimal numbers (called octets) separated by periods; each number can be written as 0 to 255 (e.g., 0.0.0.0 to 255.255.255.255).
Example of valid IP address
- 115.42.150.37
- 192.168.0.1
- 110.234.52.124
Example of invalid IP address
- 210.110 – must have 4 octets
- 255 – must have 4 octets
- y.y.y.y – the only digit has allowed
- 255.0.0.y – the only digit has allowed
- 666.10.10.20 – digit must between [0-255]
- 4444.11.11.11 – digit must between [0-255]
- 33.3333.33.3 – digit must between [0-255]
JavaScript code to validate an IP address
function ValidateIPaddress(ipaddress)
{
if (/^(25[0-5]|2[0-4][0-9]|[01]?[0-9][0-9]?)\.(25[0-5]|2[0-4][0-9]|[01]?[0-9][0-9]?)\.(25[0-5]|2[0-4][0-9]|[01]?[0-9][0-9]?)\.(25[0-5]|2[0-4][0-9]|[01]?[0-9][0-9]?)$/.test(myForm.emailAddr.value))
{
return (true)
}
alert("You have entered an invalid IP address!")
return (false)
}
Explanation of the said Regular expression (IP address)
Regular Expression Pattern :
/^(25[0-5]|2[0-4][0-9]|[01]?[0-9][0-9]?)\.(25[0-5]|2[0-4][0-9]|[01]?[0-9][0-9]?)\.(25[0-5]|2[0-4][0-9]|[01]?[0-9][0-9]?)\.(25[0-5]|2[0-4][0-9]|[01]?[0-9][0-9]?)$/
Character | Description |
---|---|
/ .. / | All regular expressions start and end with forward slashes. |
^ | Matches the beginning of the string or line. |
25[0-5] | Matches 250 or 251 or 252 or 253 or 254 or 255. |
| | or |
2[0-4][0-9] | Start with 2, follow a single character between 0-4 and again a single character between 0-9. |
| | or |
[01] | |
? | Matches the previous character 0 or 1 time. |
[0-9][0-9] | Matches a single character between 0-9 and again a single character between 0-9. |
? | Matches the previous character 0 or 1 time. |
\. | Matches the character "." literally. |
Note: Last two parts of the regular expression is similar to above.
Syntax diagram - IP-address validation:
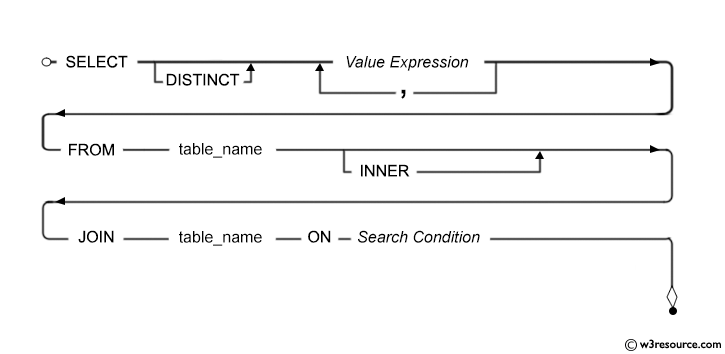
Let apply the above JavaScript function in an HTML form.
HTML Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>JavaScript form validation - checking IP address/title>
<link rel='stylesheet' href='form-style.css' type='text/css' />
</head>
<body onload='document.form1.text1.focus()'>
<div class="mail">
<h2>Input an IP address and Submit</h2>
<form name="form1" action="#">
<ul>
<li><input type='text' name='text1'/></li>
<li> </li>
<li class="submit"><input type="submit" name="submit" value="Submit" onclick="ValidateIPaddress(document.form1.text1)"/></li>
<li> </li>
</ul>
</form>
</div>
<script src="ipaddress-validation.js"></script>
</body>
</html>
JavaScript Code
function ValidateIPaddress(inputText)
{
var ipformat = /^(25[0-5]|2[0-4][0-9]|[01]?[0-9][0-9]?)\.(25[0-5]|2[0-4][0-9]|[01]?[0-9][0-9]?)\.(25[0-5]|2[0-4][0-9]|[01]?[0-9][0-9]?)\.(25[0-5]|2[0-4][0-9]|[01]?[0-9][0-9]?)$/;
if(inputText.value.match(ipformat))
{
document.form1.text1.focus();
return true;
}
else
{
alert("You have entered an invalid IP address!");
document.form1.text1.focus();
return false;
}
}
Flowchart:
CSS Code
li {list-style-type: none;
font-size: 16pt;
}
.mail {
margin: auto;
padding-top: 10px;
padding-bottom: 10px;
width: 400px;
background : #D8F1F8;
border: 1px soild silver;
}
.mail h2 {
margin-left: 38px;
}
input {
font-size: 20pt;
}
input:focus, textarea:focus{
background-color: lightyellow;
}
input submit {
font-size: 12pt;
}
.rq {
color: #FF0000;
font-size: 10pt;
}
View the Javascript IP address validation in the browser
file_download Download the validation code from here.
Other JavaScript Validation:
- Checking for non-empty
- Checking for all letters
- Checking for all numbers
- Checking for floating numbers
- Checking for letters and numbers
- Checking string length
- Email Validation
- Date Validation
- A sample Registration Form
- Phone No. Validation
- Credit Card No. Validation
- Password Validation
- IP address Validation
Previous: JavaScript : HTML Form validation - checking for password
Next: JavaScript Cookies
Test your Programming skills with w3resource's quiz.