Java Thread Interaction
Introduction
The Object class has three methods, wait(), notify(), and notifyAll()that help threads communicate about the status of an event that the threads care about. In last tutorial, we have seen about synchronization on resources where one thread can acquire a lock which forces other threads to wait for lock availability. Object class has these three methods for inter-thread communication.
For example, we have two threads named car_owner and car_meachanic, if thread car_mechanicis busy, so car_owner thread has to wait and keep checking for the car_mechanic thread to get free. Using the wait and notify mechanism, the car_owner for service thread could check for car_mechanic, and if it doesn't find any it can say, “Hey, I’m not going to waste my time checking every few minutes. I’m going to go hang out, and when a car_mechanic gets free, have him notify me so I can go back to runnable and do some work.” In other words, using wait() and notify() lets one thread put itself into a “waiting room” until some another thread notifies it that there’s a reason to come back out.
Method Signature | Description |
final void wait( ) throws InterruptedException | Calling this method will male calling thread to give up the monitor and go to sleep until some other thread enters the same monitor and calls notify( ) method. |
final void notify( ) | This method is similar to notify(), but it wakes up all the threads which are on waiting for state for object lock but only one thread will get access to object lock. |
One key point to remember about wait/notify is this:
wait(), notify(), and notifyAll() must be called from within a synchronized context. A thread can't invoke a wait or notify method on an object unless it owns that object's lock.
Below program explains the concept of car service queue where car_owner and car_mechanic thread interact with each other in the loop.
Java Code:
package threadcommunication;
public class CarOwner implements Runnable {
CarQueueClass q;
CarOwner(CarQueueClass queue){
this.q=queue;
new Thread(this, "CarOwner").start();
}
@Override
public void run() {
int count =0;
try {
while(count< 5){
Thread.sleep(2000);
q.put(count++);
}
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
package threadcommunication;
public class CarMechanic implements Runnable {
CarQueueClass q;
CarMechanic(CarQueueClass queue){
this.q=queue;
new Thread(this, "CarMechanic").start();
}
@Override
public void run() {
for(int i=0;i< 5;i++)
q.get();
}
}
package threadcommunication;
public class CarQueueClass {
int n;
boolean mechanic_available = false;
synchronized int get() {
if(!mechanic_available)
try {
wait(5000);
} catch(InterruptedException e) {
System.out.println("InterruptedException caught");
}
System.out.println("Got Request for Car Service: " + n);
mechanic_available = false;
notify();
return n;
}
synchronized void put(int n) {
if(mechanic_available)
try {
wait(5000);
} catch(InterruptedException e) {
System.out.println("InterruptedException caught");
}
this.n = n;
mechanic_available = true;
System.out.println("Put Request for Car Service: " + n);
notify();
}
}
package threadcommunication;
public class CarServiceDemo {
/**
* @param args
*/
public static void main(String[] args) {
CarQueueClass q = new CarQueueClass();
new CarOwner(q);
new CarMechanic(q);
System.out.println("Press Control-C to stop.");
}
}
Output:
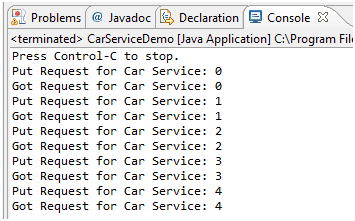
Summary:
- The wait() method puts a thread in waiting for pool from running state.
- The notify() method is used to send a signal to one and only one of the threads that are waiting in that same object's waiting pool.
- The method notifyAll() works in the same way as for notify(), only it sends the signal to all of the threads waiting on the object.
- All three methods—wait(), notify(), and notifyAll()—must be called from within a synchronized context. A thread invokes wait() or notify() on a particular object, and the thread must currently hold the lock on that object.
Java Code Editor:
Previous:Java Thread States and Transitions
Next: Java Code Synchronization