Custom Exceptions
Introduction
We have talked about how to handle exceptions when they’re produced by calling methods in Java APIs. Java also lets you create and use custom exceptions—classes of your own exception as per application need which will be used to represent errors. Normally, you create a custom exception to represent some type of error within your application—to give a new, distinct meaning to one or more problems that can occur within your code. You may do this to show similarities between errors that exist in several places throughout your code, to differentiate one or more errors from similar problems that could occur as your code runs, or to give special meaning to a group of errors within your application.
It’s fairly easy to create and use a custom exception. There are three basic steps that you need to follow. We will explain this by an example of bank account balance. Here we want to have flexi-deposit functionality i.e. when account balance goes beyond 20k, a new deposit will be created.
Define the exception class
You typically represent a custom exception by defining a new class. In many cases, all you need to do is to create a subclass of an existing exception class:
Java Code:
public class AccountBalanceException extends Exception {
private float accountBalance ;
public AccountBalanceException(float f){
super();
this.accountBalance =f;
}
public AccountBalanceException(String message){
super(message);
}
public float getAccountBalance(){
return accountBalance;
}
}
At a minimum, you need to subclass Throwable or one of its subclasses. Often,you’ll also define one or more constructors to store information like an error message in the object, as shown in lines 6-12. Our exception class AccountBalanceException has two constructors. One with String argument and the second constructor is having float argument. When you subclass any exception, you automatically inherit some standard features from the Throwable class, such as:
- Error message
- Stack trace
- Exception wrapping
Declare that your exception-producing method throws your custom exception
Using throws keyword we can declare the method which might be exception producing. In order to use a custom exception, you must show classes that call your code that they need to plan for this new type of exception. You do this by declaring that one or more of your methods throws the exception. Below is code for account balance management. AccountManagement class has main() method and two utility methods addAmount() and createFixDeposit(). Here we have assumecurrentBalance as Rs. 15000. If account balance goes beyond Rs. 20000, the amount above 20,000 will be passed to make fix-deposit.
Java Code: Go to the editor
import java.util.Scanner;
public class AccountManagement {
private float currentBalance =15000f;
public static void main(String[] args) {
Scanner inputDevice = new Scanner(System.in);
System.out.print("Please enter amount to add in your balance: ");
float newAmount = inputDevice.nextFloat();
try{
float totalAmount = new AccountManagement().AddAmount(newAmount);
System.out.println("Total Account Balance = "+ totalAmount);
}catch (AccountBalanceException a){
float fdAmount = a.getAccountBalance() - 20000 ;
System.out.println("Your account balance is more than 20K now, So creating FD of Amount: "+ fdAmount);
new AccountManagement().createFixDeposit(fdAmount);
System.out.println("Account Balance = "+20000);
}
}
public float AddAmount(float amount) throws AccountBalanceException{
float total = currentBalance+amount;
if (total>20000){
throw new AccountBalanceException(total);
}
return total;
}
public void createFixDeposit(float fxAmount){
//Implimentation of FD creation
}
}
class AccountBalanceException extends Exception {
private float accountBalance ;
public AccountBalanceException(float f){
super();
this.accountBalance =f;
}
public AccountBalanceException(String message){
super(message);
}
public float getAccountBalance(){
return accountBalance;
}
}
Find the point(s) of failure in your error-producing method, create the exception and dispatch it using the keyword “throw”
The third and final step is to actually create the object and propagate it through the system. To do this, you need to know where your code needs special processing in the method. Here, Throwable Instance must be an object of type Throwable or a subclass of Throwable. There are two ways you can obtain a Throwable object: using a parameter in a catch clause or creating one with the new operator. The flow of execution stops immediately after the throw statement; any subsequent statements are not executed. The nearest enclosing try block is inspected to see if it has a catch statement that matches the type of exception. If it does find a match, control is transferred to that statement. If not, then the next enclosing try statement is inspected, and so on.
Output of above program based on various input parameters as below,
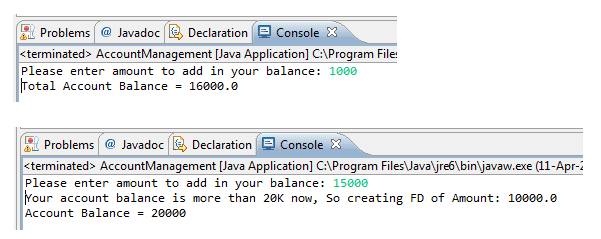
Summary:
- Java provides exception classes for most of the common type of errors as well as provides a mechanism to define custom exception classes.
- Three steps to create and use custom exception class (1) Define exception class (2) Declare exception prone method with throws keyword (3) Check condition to throw new exception object to be handled by calling the method.
Java Code Editor:
Previous: Checked and unchecked
Next:Try with resource feature of Java 7