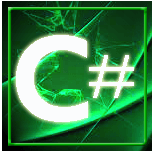
C# Sharp Searching and Sorting Algorithm Exercises: Composite structures
C# Sharp Searching and Sorting Algorithm: Exercise-1 with Solution
Write a C# Sharp program of composite structures.
C# Sharp Code:
using System; using System.Collections.Generic; using System.Linq; class Program { struct Entry { public Entry(string name, int value) { Name = name; Value = value; } public string Name; public int Value; } static void Main(string[] args) { var Elements = new List<Entry> { new Entry("Afghanistan", 93), new Entry("Austria", 43), new Entry("Swaziland", 268), new Entry("United States", 1), new Entry("Singapore", 65), new Entry("Portugal", 351) }; var sortedElements = Elements.OrderBy(c => c.Name); Console.Write("Country Name Country Code\n"); foreach (Entry c in sortedElements) Console.WriteLine("{0,-15}{1}", c.Name, c.Value); } }
C# Sharp Practice online: