Java Defining, Instantiating, and Starting Threads
Introduction
One of the most appealing features in Java is the support for easy thread programming. Java provides built-in support for multithreaded programming. A multithreaded program contains two or more parts that can run concurrently. Each part of such a program is called a thread, and each thread defines a separate path of execution. Thus we can say that multithreading is a specialized form of multitasking.
The formal definition of a thread is, A thread is a basic processing unit to which an operating system allocates processor time, and more than one thread can be executing code inside a process. A thread is sometimes called a lightweight process or an execution context
Imagine an online ticket reservation application with a lot of complex capabilities. One of its functions is "search for train/flight tickets from source and destination" another is "check for prices and availability," and a third time-consuming operation is "ticket booking for multiple clients at a time".
In a single-threaded runtime environment, these actions execute one after another. The next action can happen only when the previous one is finished. If a ticket booking takes 10 mins, then other users have to wait for their search operation or book operation. This kind of application will result into waste of time and clients. To avoid this kind of problems java provides multithreading features where multiple operations can take place simultaneously and faster response can be achieved for better user experience. Multithreading enables you to write very efficient programs that make maximum use of the CPU, because idle time can be kept to a minimum.
Defining a Thread
In the most general sense, you create a thread by instantiating an object of type Thread.
Java defines two ways in which this can be accomplished:
- You can implement the Runnable interface.
- You can extend the Thread class
Implementing Runnable
The easiest way to create a thread is to create a class that implements the Runnable interface. Runnable abstracts a unit of executable code. You can construct a thread on any object that implements Runnable. To implement Runnable, a class need only implements a single method called run( ), which is declared like this:
public void run( )
Inside run( ), you will define the code that constitutes the new thread. It is important to understand that run( ) can call other methods, use other classes, and declare variables, just like the main thread can. The only difference is that run( ) establishes the entry point for another, concurrent thread of execution within your program. This thread will end when run( ) returns.
class MyRunnableThread implements Runnable { public void run() { System.out.println("Important job running in MyRunnableThread"); } }
Extending java.lang.Thread
The simplest way to define code to run in a separate thread is to
- Extend the java.lang.Thread class.
- Override the run() method.
It looks like this:
classMyThread extends Thread { public void run() { System.out.println("Important job running in MyThread"); } }
The limitation with this approach (besides being a poor design choice in most cases) is that if you extend Thread, you can't extend anything else. And it's not as if you really need that inherited Thread class behavior because in order to use a thread you'll need to instantiate one anyway.
Instantiating a Thread
Remember, every thread of execution begins as an instance of class Thread. Regardless of whether your run() method is in a Thread subclass or a Runnable implementation class, you still need a Thread object to do the work.
If you have approach two (extending Thread class): Instantiation would be simple
MyThread thread = new MyThread();
If you implement Runnable, instantiation is only slightly less simple.
To instantiate your Runnable class:
MyRunnableThreadmyRunnable = new MyRunnableThread (); Thread thread = new Thread(myRunnable); // Pass your Runnable to the Thread
Giving the same target to multiple threads means that several threads of execution will be running the very same job (and that the same job will be done multiple times).
Thread Class Constructors
- Thread() :
Default constructor – To create thread with default name and priority
- Thread(Runnable target)
This constructor will createa thread from the runnable object.
- Thread(Runnable target, String name)
This constructor will create thread from runnable object with name as passed in the second argument
- Thread(String name)
This constructor will create a thread with the name as per argument passed.
So now we've made a Thread instance, and it knows which run() method to call. But nothing is happening yet. At this point, all we've got is a plain old Java object of type Thread. It is not yet a thread of execution. To get an actual thread—a new call stack—we still have to start the thread.
Starting a Thread
You've created a Thread object and it knows its target (either the passed-inRunnable or itself if you extended class Thread). Now it's time to get the whole thread thing happening—to launch a new call stack. It's so simple it hardly deserves its own subheading:
t.start();
Prior to calling start() on a Thread instance, the thread is said to be in the new state. There are various thread states which we will cover in next tutorial.
When we call t.start() method following things happens:
- A new thread of execution starts (with a new call stack).
- The thread moves from the new state to the runnable state.
- When the thread gets a chance to execute, its target run() method will run.
The following example demonstrates what we've covered so far—defining, instantiating, and starting a thread: In below Java program we are not implementing thread communication or synchronization, because of that output may depend on operating system’s scheduling mechanism and JDK version.
We are creating two threads t1 and t2 of MyRunnable class object. Starting both threads, each thread is printing thread name in the loop.
Java Code ( MyRunnable.java )
package mythreading;
public class MyRunnable implements Runnable{
@Override
public void run() {
for(int x =1; x < 10; x++) {
System.out.println("MyRunnable running for Thread Name: " + Thread.currentThread().getName());
}
}
}
Java Code ( TestMyRunnable.java )
package mythreading;
public class TestMyRunnable {
public static void main (String [] args) {
MyRunnable myrunnable = new MyRunnable();
//Passing myrunnable object to Thread class constructor
Thread t1 = new Thread(myrunnable);
t1.setName("Amit-1 Thread");
//Starting Thread t1
t1.start();
Thread t2 = new Thread(myrunnable);
t2.setName("Amit-2 Thread");
t2.start();
}
}
Output:
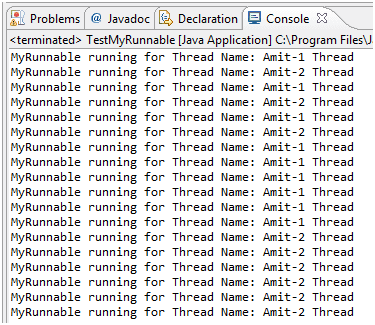
Summary:
- Threads can be created by extending Thread and overriding the public void run() method
- Thread objects can also be created by calling the Thread constructor that takes a Runnable argument. The Runnable object is said to be the target of the thread.
- You can call start() on a Thread object only once. If start() is called more than once on a Thread object, it will throw a Runtime Exception.
- Each thread has its own call stack which is storing state of thread execution
- When a Thread object is created, it does not become a thread of execution until its start() method is invoked. When a Thread object exists but hasn't been started, it is in the new state and is not considered alive.
Java Code Editor:
Previous: Java Utility Class
Next: Java Thread States and Transitions
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics