Java Serialization
Introduction
Creating object is a fundamental feature of Java and all other object-oriented languages. Java allows you to write entire objects to files, instead of separately writing individual fields.
Imagine you want to save the state of one or more objects. If Java didn't have serialization (as the earliest version did not), you'd have to use one of the I/O classes to write out the state of the instance variables of all the objects you want to save. Serialization lets you simply say "save this object and all of its instance variables."
Serialization is Java’s built-in mechanism for manipulating objects as streams of bytes; the Serializable interface endows your class with the ability to be serialized.
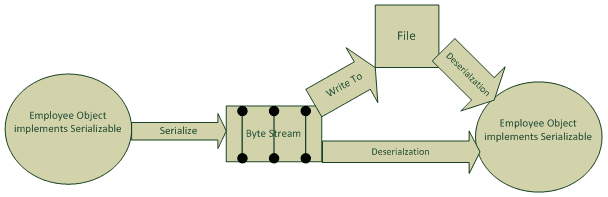
Working with ObjectOutputStream and ObjectInputStream
The magic of basic serialization happens with just two methods: one to serialize objects and write them to a stream and a second to read the stream and deserialize objects.
ObjectOutputStream.writeObject() // serialize and write
ObjectInputStream.readObject() // read and deserialize
The java.io.ObjectOutputStream and java.io.ObjectInputStream classes are considered to be higher-level classes in the java.io package, and as we learned earlier, that means that you'll wrap them around lower-level classes, such as java.io.FileOutputStream and java.io.FileInputStream.
Here's a small program that creates an (Employee) object, serializes it, and then deserializes it:
Java Code:
package serializationdemo;
import java.io.*;
public class EmployeeSerialDemo {
public static void main(String[] args) {
Employee c = new Employee("Suresh", "E123"); // 2
File outFile = new File("empSerial.ser");
try {
FileOutputStream fs = new FileOutputStream(outFile);
ObjectOutputStream os = new ObjectOutputStream(fs);
os.writeObject(c); // 3
os.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Below program will read empSerial.ser file created by above program which holds the state of an object. File will created under project root directory as shown below (default path, as we have not mentioned fully qualified path)
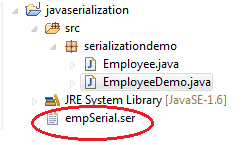
Deserialization Demo Java class
Java Code:
package serializationdemo;
import java.io.*;
public class EmployeeDeserialDemo {
public static void main(String[] args) {
File ReadFile = new File("empSerial.ser");
try {
FileInputStream fis = new FileInputStream(ReadFile);
ObjectInputStream ois = new ObjectInputStream(fis);
Employee e = (Employee) ois.readObject();
System.out.println("Deserialized Employee name= "+ e.geteName());
System.out.println("Deserialized Employee ID= "+ e.geteId());
ois.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output:
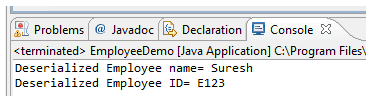
Let's take a look at the key points in this example
1. We declare that the Employee class implements the Serializable interface. Serializable is a marker interface; it has no methods to implement.
2. We make a new Employee object, which as we know is Serializable.
3. We serialize the Employee object c by invoking the writeObject() method. The invocation ofwriteObject() performs two tasks: it serializes the object, and then it writes the serialized object to a file.
4. We de-serialize the Employee object by invoking the readObject() method. ThereadObject() method returns an Object, so we have to cast the deserialized object back to an Employee object.
Serialization with Composition(Has-A) relationship
Composition (HAS-A) simply mean the use of instance variables that are references to other objects. Let’s understand if Employee class has department object reference and if department class does not implement A Serializable interface then we have to declare department reference as transient or we will get an exception while calling serialize method.
Java Code:
package serializationdemo;
import java.io.*;
public class EmployeeWithDepartmentSerialDemo {
public static void main(String[] args) {
// Creating object of NewEmployee class
NewEmployee c = new NewEmployee("Suresh", "E123", new Department());
File outFile = new File("NewEmpSerial.ser");
try {
FileOutputStream fs = new FileOutputStream(outFile);
ObjectOutputStream os = new ObjectOutputStream(fs);
os.writeObject(c); // 3
os.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Java Code:
package serializationdemo;
import java.io.Serializable;
class NewEmployee implements Serializable {
private static final long serialVersionUID = 1L;
private String eName;
private String eId;
private Department dName;
public String geteName() {
return eName;
}
public String geteId() {
return eId;
}
public Department getdName() {
return dName;
}
NewEmployee(String a, String b, Department d){
this.eName = a;
this.eId = b;
this.dName = d;
}
}
Output:
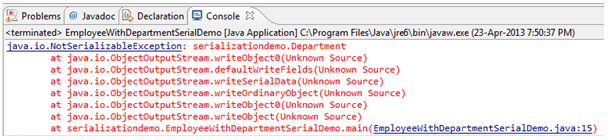
Serialization with Inheritance relationship
If a superclass is Serializable, then according to normal Java interface rules, all subclasses of that class automatically implement Serializable implicitly. In other words, a subclass of a class marked Serializable passes the IS-A test for Serializable, and thus can be saved without having to explicitly mark the subclass as Serializable.
If you serialize a collection or an array, every element must be Serializable. A single non-serializable element will cause serialization to fail if not declared transient.
Serialization Is Not for Static variables
Finally, you might notice that we've talked ONLY about instance variables, not static variables. That is because static variables are not part of instance state, so not part of Serialization process.
Summary:
- A class must implement the Serializable interface before its objects can be serialized.
- The ObjectOutputStream.writeObject() method serializes objects, and the ObjectInputStream.readObject() method deserializes objects.
- If you mark an instance variable transient, it will not be serialized even though the rest of the object's state will be.
- If a superclass implements Serializable, then its subclasses do automatically.
Java Code Editor:
Previous: Java Property File Processing
Next: Java Collection Framework
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics