Java TreeSet
Introduction
The TreeSet is one of two sorted collections (the other being TreeMap).TreeSet extends AbstractSet and implements the NavigableSet interface. It creates a collection that uses a tree for storage. Objects are stored in sorted, ascending order according to the natural order. Optionally, you can construct a TreeSet with a constructor that lets you give the collection your own rules for what the order should be (rather than relying on the ordering defined by the elements' class) by using a Comparable or Comparator.
Access and retrieval times are quite fast, which makes TreeSet an excellent choice when storing large amounts of sorted information that must be found quickly. TreeSet might not be used when our application has requirement of modification of set in terms of frequent addition of elements.
Constructors
TreeSet( ); // Default Constructor TreeSet(Collection<? extends E> c); //TreeSet from Collection C TreeSet(Comparator<? super E> comp); // TreeSet with custom ordering as per Comparator TreeSet(SortedSet<E>ss); //TreeSet that contains the elements of ss.
Important Methods of TreeSet Class
Method | Description |
---|---|
void add(Object o) | Adds the specified element to this set if it is not already present. |
void clear() | Removes all of the elements from this set. |
Object first() | Returns the first (lowest) element currently in this sorted set. |
Object last() | Returns the last (highest) element currently in this sorted set. |
boolean isEmpty() | Returns true if this set contains no elements. |
boolean remove(Object o) | Removes the specified element from this set if it is present. |
SortedSetsubSet(Object fromElement, Object toElement) | Returns a view of the portion of this set whose elements range from fromElement, inclusive, to toElement, exclusive. |
Java Code: Go to the editor
import java.util.TreeSet;
public class TreeSetDemo {
public static void main(String[] args) {
TreeSet<String> playerSet = new TreeSet<String>();
playerSet.add("Sachin");
playerSet.add("Zahir");
playerSet.add("Mahi");
playerSet.add("Bhajji");
playerSet.add("Viru");
playerSet.add("Gautam");
playerSet.add("Ishant");
playerSet.add("Umesh");
playerSet.add("Pathan");
playerSet.add("Virat");
playerSet.add("Sachin"); // This is duplicate element so will not be added again
//below will print list in alphabetic order
System.out.println("Original Set:" + playerSet);
System.out.println("First Name: "+ playerSet.first());
System.out.println("Last Name: "+ playerSet.last());
TreeSet<String> newPlySet = (TreeSet<String>) playerSet.subSet("Mahi", "Virat");
System.out.println("Sub Set: "+ newPlySet);
}
}
Output:
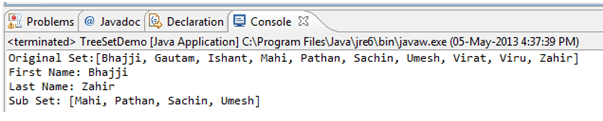
In above example, we are creating TreeSet of String object. String class is having comparable interface implemented by Java library. Let’s think of a case when we need to have our own objects to be stored in Set and ordering of objects as per our rule. Below example shows Cricketers as an object having two properties name and battingPosition. We want to store all Cricketer object as per batting position defined means when we iterate through collection we get names as per batting positions.
Java Code ( Cricketer.java)
package treeset;
public class Cricketer {
private String name;
private int battingPosition;
Cricketer(String cricketerName, int cBattingPosition){
this.name = cricketerName;
this.battingPosition = cBattingPosition;
}
public String getName() {
return name;
}
public int getBattingPosition() {
return battingPosition;
}
}
CompareCricketer.java: Here we are defining rules how to organize Cricketer objects in TreeMap.
Java Code (CompareCricketer.java)
package treeset;
import java.util.Comparator;
public class CompareCricketer implements Comparator <Cricketer> {
@Override
public int compare(Cricketer arg0, Cricketer arg1) {
if(arg0.getBattingPosition() > arg1.getBattingPosition())
return 1;
else if (arg0.getBattingPosition() < arg1.getBattingPosition())
return -1;
else return 0;
}
}
Java Code:
package treeset;
import java.util.Iterator;
import java.util.TreeSet;
public class CustomTreeSetDemo {
public static void main(String[] args) {
TreeSet<Cricketer> playerSet = new TreeSet<Cricketer>(
new CompareCricketer());
playerSet.add(new Cricketer("Sachin", 1));
playerSet.add(new Cricketer("Zahir", 9));
playerSet.add(new Cricketer("Mahi", 7));
playerSet.add(new Cricketer("Bhajji", 8));
playerSet.add(new Cricketer("Viru", 2));
playerSet.add(new Cricketer("Gautam", 4));
playerSet.add(new Cricketer("Ishant", 10));
playerSet.add(new Cricketer("Umesh", 11));
playerSet.add(new Cricketer("Pathan", 5));
playerSet.add(new Cricketer("Virat", 3));
playerSet.add(new Cricketer("Raina", 6));
Iterator<Cricketer> it = playerSet.iterator();
while (it.hasNext()) {
System.out.println(it.next().getName());
}
}
}
Output:
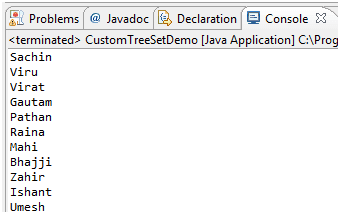
Summary:
- TreeSet is sorted collection class
- Sorting of objects in TreeSet in natural order by default or we can provide comparator class reference for customized sorting.
- While working with large amount of data access and retrieval is faster with TreeSet
Java Code Editor:
Previous: Java HashSet
Next: Java Linked HashSet