Java Branching Statements
Description
Java provides three branching statements break, continue and return. The break and continue in Java are two essential keyword beginners needs to familiar while using loops ( for loop, while loop and do while loop). break statement in java is used to break the loop and transfers control to the line immediate outside of loop while continue is used to escape current execution (iteration) and transfers control back to the start of the loop. Both break and continue allow the programmer to create sophisticated algorithm and looping constructs.
In this java tutorial, we will see the example of break and continue statement in Java and some important points related to breaking the loop using label and break statement. break keyword can also be used inside switch statement to break current choice and if not used it can cause fall-through on switch. Both the break statement and the continue statement can be unlabeled or labeled. Although it's far more common to use a break and continue unlabeled.
Let’s understand unlabeled continue and break statement using java program. Below program will do the addition of all even numbers of array till it encounters 0 or negative number from an array.
Java Code: Go to the editor
public class BreakContinueDemo {
public static void main(String[] args) {
int [] numbers = {10,23,19,34,54,23,76,39,65,24,8,0,12,55};
int sum =0;
for(int i=0; i< numbers.length; i++){
if(numbers[i]<=0){
System.out.println("Break statement coming because number is = "+ numbers[i]);
break;
}else if (numbers[i]%2 != 0){
System.out.println("Odd number found in array, ignoring number " + numbers[i]);
continue;
}else
sum = sum + numbers[i];
}
System.out.println("Sum of all even numbers is = " + sum);
}
}
Output:
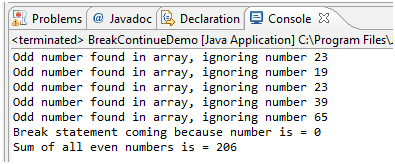
Labeled Statements
Although many statements in a Java program can be labeled, it's most common to use labels with loop statements like for or while, in conjunction with break and continue statements. A label statement must be placed just before the statement being labeled, and it consists of a valid identifier that ends with a colon (:).
You need to understand the difference between labeled and unlabeled break and continue. The labeled varieties are needed only in situations where you have nested loop and need to indicate which of the nested loops you want to break from,or from which of the nested loops you want to continue with the next iteration. A break statement will exit out of the labeled loop, as opposed to the innermost loop,if the break keyword is combined with a label.Here is an example program that uses continue to print a triangular multiplication table for 0 through 9.
Java Code: Go to the editor
public class LabledBreakContinueDemo {
public static void main(String[] args) {
int breaklimit = 9;
outer: for (int i = 0; ; i++) {
for (int j = 0; j < 10; j++) {
if (j > i) {
System.out.println();
continue outer;
}
System.out.print(" " + (i * j));
}
if(i==breaklimit){
break outer;
}
}
System.out.println();
}
}
Output:
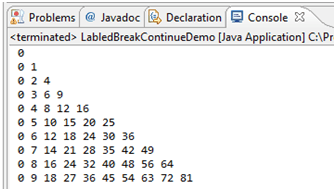
The return statement
The last control statement is return. The return statement is used to explicitly return from a method. That is, it causes program control to transfer back to the caller of the method. As such, it is categorized as a jump statement. At any time in a method, the return statement can be used to cause execution to branch back to the caller of the method. Thus, the return statement immediately terminates the method in which it is executed. The following example illustrates this point. In below program main () is calling method and checkEven() is called the method. Execution of checkEven() method ends when return statement encountered.
Java Code: Go to the editor
public class ReturnDemo {
public static void main(String[] args) {
for (int k =25; k< 31; k++){
new ReturnDemo().checkEven(k);
}
}
public boolean checkEven(int a){
if (a%2 == 0){
System.out.println(a + " is even number");
return true;
}
System.out.println(a + " is odd number");
return false;
}
}
Output:
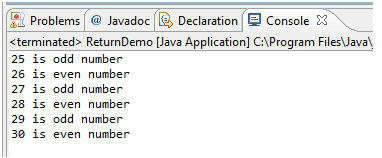
Summary
- Java provides 3 branching statement named break, continue and return.
- Branching statements are used to change the normal flow of execution based on some condition.
- The return statement is used to explicitly return from a method.
Java Code Editor:
Previous: For loop
Next: Handling Exceptions