StringBuffer / StringBuilder Class
Introduction
In Java String is immutable objects means once created it cannot be changed, reference will point to a new object. If our application has string manipulation activity, then there will be many discarded string object in heap memory which might result in performance impact. To circumvent these limitations, you can use either the StringBuilder or StringBuffer class. You use one of these classes, which are alternatives to the String class, when you know a String will be modified; usually, you can use a StringBuilder or StringBuffer object anywhere you would use a String. StringBuffer may have characters and substrings inserted in the middle or appended to the end. StringBuffer will automatically grow to make room for such additions and often has more characters pre-allocated than are actually needed, to allow room for growth. Similar to String class, these two classes are part of the java.lang package and are automatically imported into every program. The classes are identical except for the following:
- StringBuilder is more efficient.
- StringBuffer is thread safe because of all synchronized methods.
Let us see below program which is comparing the performance of String and String buffer objects. In this program, we are creating new string object interactively inside for loop. In next program, we are replacing String object with StringBuffer. Application time is calculated and displayed in a millisecond.
Java Code: Go to the editor
import java.util.Date;
import java.sql.Timestamp;
public class StringPerformanceCompare {
public static void main(String[] args) {
Date sDate = new Date();
long sTime = sDate.getTime();
System.out.println("Start Time for StringBuffer: " + new Timestamp(sTime));
StringBuffer s = new StringBuffer("AA");
for (int i=0; i< 10000; i++){
s.append(i);
}
Date eDate = new Date();
long eTime = eDate.getTime();
System.out.println("End Time for StringBuffer: " + new Timestamp(eTime));
System.out.println("Time taken to Execute StringBuffer process " + (eTime-sTime) + "ms");
System.out.println("=====================================================================");
Date strDate = new Date();
long strTime = strDate.getTime();
System.out.println("Start Time for String: " + new Timestamp(strTime));
String str = new String("AA");
for (int i=0; i< 10000; i++){
str+=i;
}
Date eStrDate = new Date();
long eStrTime = eStrDate.getTime();
System.out.println("End Time for String: " + new Timestamp(eStrTime));
System.out.println("Time taken to Execute String process " + (eStrTime-strTime) + "ms");
}
}
Output:
As seen in output screen, String objects are slightly slower in performance due to new object creation.
Important Methods of StringBuffer & StringBuilder classes
StringBuffer and StringBuilder class has one difference that StringBuffer is having all the methods synchronized while StringBuilder is not thread safe so better performance. Here we will discuss methods of StringBuilder which is applicable to StringBuffer as well.
StringBuilder delete (int startIndex, int endIndex)
This method deletes a portion of StringBuilder’s character sequence. We need to provide two int argument startIndex and endIndex. For example
StringBuilder sb = new StringBuilder("JavaWorld"); sb.delete(4, 8);//sb value would be Javad
StringBuilder insert(int offset, String s )
This method used when we want to insert particular char sequence, string or any primitive types at particular index inside StringBuilder character sequence. For example
StringBuilder sb = new StringBuilder("ABC"); sb.insert(1, "xyz"); // Here sb value is ABxyzC
StringBuilder replace (int start, int end, String s)
This method will replace particular portion of character sequence with third argument (String) mentioned in method call. Syntax example:
StringBuilder sb = new StringBuilder("ABCDEF"); sb.replace(1, 3, "XYZ"); System.out.println(sb); //here sb value is AXYZDEF
StringBuilder reverse()
As the name suggest, this method will reverse the sequence of characters in target StringBuilder object. Syntax example
StringBuilder sb = new StringBuilder("ABCDEF"); sb.reverse();// here sb value is FEDCBA
void setCharAt(int index, char ch)
This method should be used when we want to replace one character at particular index with the second argument of the method. Syntax example,
StringBuilder sb = new StringBuilder("ABCDEF"); sb.setCharAt(3, 'x'); //This point sb value is ABCxEF
Below Java program shows how to use these important methods in java program along with output.
Java Code: Go to the editor
public class StringBuilderDemo {
public static void main(String[] args) {
StringBuilder sb1 = new StringBuilder("Hello Java World");
sb1.delete(4, 8);
System.out.println("Delete method demo: " + sb1);
StringBuilder sb2 = new StringBuilder("Hello Java World");
sb2.insert(4, "abc");
System.out.println("Inser Operation: "+sb2);
StringBuilder sb3 = new StringBuilder("W3resource.com");
sb3.replace(1, 4, "Amit");
System.out.println("Replace Operation: "+sb3);
StringBuilder sb4 = new StringBuilder("ABCDE");
System.out.println("Reverse of ABCDE: "+ sb4.reverse());
StringBuilder sb5 = new StringBuilder("ABCDEF");
sb5.setCharAt(3, 'x');
System.out.println("Replacing char at index 3: "+ sb5);
}
}
Output:
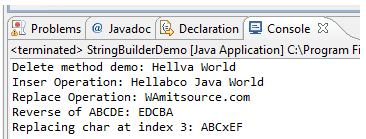
Summary
- String objects are immutable which makes performance of application bit slower
- Java provides StringBuffer and StringBuilder classes for improved string manipulation operation.
- StringBuffer and StringBuilder are similar classes except StringBuffer has all methods synchronized while StringBuilder has non-synchronized methods.
Java Code Editor:
Previous: Important methods of String class with example
Next:File Input and Output