Java LinkedHashSet
Introduction
A LinkedHashSet is an ordered version of HashSet that maintains a doubly-linked List across all elements. Use this class instead of HashSet when you care about the iteration order. When you iterate through a HashSet the order is unpredictable, while a LinkedHashSet lets you iterate through the elements in the order in which they were inserted.when cycling through LinkedHashSet using an iterator, the elements will be returned in the order in which they were inserted.
LinkedHashSet constructors
LinkedHashSet() // Default constructor LinkedHashSet(Collection c) // It creates LinkedHashSet from collection c LinkedHashSet( int capacity) // it creates LinkedHashSet with initial capacity mentioned LinkedHashSet(int capacity, float loadFactor) // This creates LinkedHashSet with capacity and load factor
LinkedHashSet Methods
Method | Purpose |
---|---|
public boolean add(Object o) | Adds an object to a LinkedHashSet if already not present in HashSet. |
public boolean remove(Object o) | Removes an object from LinkedHashSet if found in HashSet. |
public boolean contains(Object o) | Returns true if object found else return false |
public boolean isEmpty() | Returns true if LinkedHashSet is empty else return false |
public int size() | Returns number of elements in the LinkedHashSet |
Java Code:Go to the editor
import java.util.LinkedHashSet;
public class LinkedHashSetDemo {
public static void main(String[] args) {
LinkedHashSet<String> linkedset = new LinkedHashSet<String>();
// Adding element to LinkedHashSet
linkedset.add("Maruti");
linkedset.add("BMW");
linkedset.add("Honda");
linkedset.add("Audi");
linkedset.add("Maruti"); //This will not add new element as Maruti already exists
linkedset.add("WalksWagon");
System.out.println("Size of LinkedHashSet=" + linkedset.size());
System.out.println("Original LinkedHashSet:" + linkedset);
System.out.println("Removing Audi from LinkedHashSet: " + linkedset.remove("Audi"));
System.out.println("Trying to Remove Z which is not present: "
+ linkedset.remove("Z"));
System.out.println("Checking if Maruti is present=" + linkedset.contains("Maruti"));
System.out.println("Updated LinkedHashSet: " + linkedset);
}
}
Output:
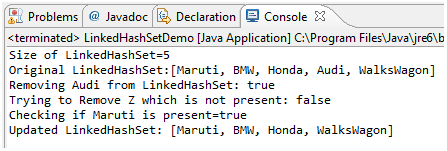
We can user Iterator object to iterate through our collection. While iterating we can add or remove objects from the collection. Below program demonstrates the use of iterator in LinkedHashSet collection.
Java Code:
package linkedHashSet;
import java.util.Iterator;
import java.util.LinkedHashSet;
import java.util.Set;
public class LinkedHashSetIterator {
public static void main(String[] args) {
Set<String> myCricketerSet = new LinkedHashSet<String>();
myCricketerSet.add("Ashwin");
myCricketerSet.add("Dhoni");
myCricketerSet.add("Jadeja");
myCricketerSet.add("Bravo");
myCricketerSet.add("Hussy");
myCricketerSet.add("Morkal");
myCricketerSet.add("Vijay");
myCricketerSet.add("Raina");
Iterator<String> setIterator = myCricketerSet.iterator();
while(setIterator.hasNext()){
System.out.println(setIterator.next());
}
}
Output:
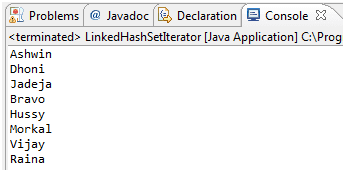
Summary:
- LinkedHashSet provides collection of unique objects
- LinkedHashSet is unsorted and non-indexed based collection class
- Iteration in LinkedHashSet is as per insertion order
Java Code Editor:
Previous: Java TreeSet
Next: Java Maps