Java Thread Methods and Thread States
Introduction
We have various methods which can be called on Thread class object. These methods are very useful when writing a multithreaded application. Thread class has following important methods. We will understand various thread states as well later in this tutorial.
Method Signature | Description |
String getName() | Retrieves the name of running thread in the current context in String format |
void start() | This method will start a new thread of execution by calling run() method of Thread/runnable object. |
void run() | This method is the entry point of the thread. Execution of thread starts from this method. |
void sleep(int sleeptime) | This method suspend the thread for mentioned time duration in argument (sleeptime in ms) |
void yield() | By invoking this method the current thread pause its execution temporarily and allow other threads to execute. |
void join() | This method used to queue up a thread in execution. Once called on thread, current thread will wait till calling thread completes its execution |
boolean isAlive() | This method will check if thread is alive or dead |
Thread States
The thread scheduler's job is to move threads in and out of the therunning state. While the thread scheduler can move a thread from the running state back to runnable, other factors can cause a thread to move out of running, but not back to runnable. One of these is when the thread's run()method completes, in which case the thread moves from the running state directly to the dead state.
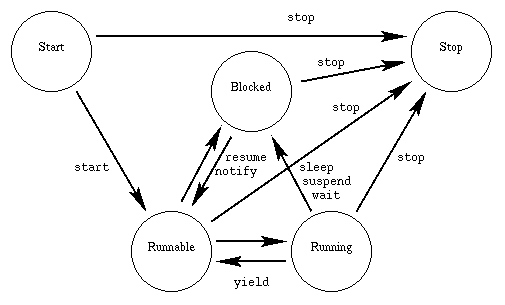
New/Start:
This is the state the thread is in after the Thread instance has been created, but the start() method has not been invoked on the thread. It is a live Thread object, but not yet a thread of execution. At this point, the thread is considered not alive.
Runnable:
This means that a thread can be run when the time-slicing mechanism has CPU cycles available for the thread. Thus, the thread might or might not be running at any moment, but there’s nothing to prevent it from being run if the scheduler can arrange it. That is, it’s not dead or blocked.
Running:
This state is important state where the action is. This is the state a thread is in when the thread scheduler selects it (from the runnable pool) to be the currently executing process. A thread can transition out of a running state for several reasons, including because "the thread scheduler felt like it". There are several ways to get to the runnable state, but only one way to get to the running state: the scheduler chooses a thread from the runnable pool of thread.
Blocked:
The thread can be run, but something prevents it. While a thread is in the blocked state, the scheduler will simply skip it and not give it any CPU time. Until a thread reenters the runnable state, it won’t perform any operations. Blocked state has some sub-states as below,
- Blocked on I/O: The thread waits for completion of blocking operation. A thread can enter this state because of waiting I/O resource. In that case, the thread sends back to runnable state after the availability of resources.
- Blocked for join completion: The thread can come in this state because of waiting for the completion of another thread.
- Blocked for lock acquisition: The thread can come in this state because of waiting for acquire the lock of an object.
Dead:
A thread in the dead or terminated state is no longer schedulable and will not receive any CPU time. Its task is completed, and it is no longer runnable. One way for a task to die is by returning from its run( ) method, but a task’s thread can also be interrupted, as you’ll see shortly.
Let's take an example of Java program to demonstrate various thread state and methods of thread class.
Java Code ( AnimalRunnable.java )
package threadstates;
public class AnimalRunnable implements Runnable {
@Override
public void run() {
for (int x = 1; x < 4; x++) {
System.out.println("Run by " + Thread.currentThread().getName());
try {
Thread.sleep(1000);
} catch (InterruptedException ex) {
ex.printStackTrace();
}
}
System.out.println("Thread State of: "+ Thread.currentThread().getName()+ " - "+Thread.currentThread().getState());
System.out.println("Exit of Thread: "
+ Thread.currentThread().getName());
}
}
Java Code ( AnimalMultiThreadDemo.java ): Go to the editor
public class AnimalMultiThreadDemo {
public static void main(String[] args) throws Exception{
// Make object of Runnable
AnimalRunnable anr = new AnimalRunnable();
Thread cat = new Thread(anr);
cat.setName("Cat");
Thread dog = new Thread(anr);
dog.setName("Dog");
Thread cow = new Thread(anr);
cow.setName("Cow");
System.out.println("Thread State of Cat before calling start: "+cat.getState());
cat.start();
dog.start();
cow.start();
System.out.println("Thread State of Cat in Main method before Sleep: " + cat.getState());
System.out.println("Thread State of Dog in Main method before Sleep: " + dog.getState());
System.out.println("Thread State of Cow in Main method before Sleep: " + cow.getState());
Thread.sleep(10000);
System.out.println("Thread State of Cat in Main method after sleep: " + cat.getState());
System.out.println("Thread State of Dog in Main method after sleep: " + dog.getState());
System.out.println("Thread State of Cow in Main method after sleep: " + cow.getState());
}
}
class AnimalRunnable implements Runnable {
@Override
public void run() {
for (int x = 1; x < 4; x++) {
System.out.println("Run by " + Thread.currentThread().getName());
try {
Thread.sleep(1000);
} catch (InterruptedException ex) {
ex.printStackTrace();
}
}
System.out.println("Thread State of: "+ Thread.currentThread().getName()+ " - "+Thread.currentThread().getState());
System.out.println("Exit of Thread: "
+ Thread.currentThread().getName());
}
}
Output:
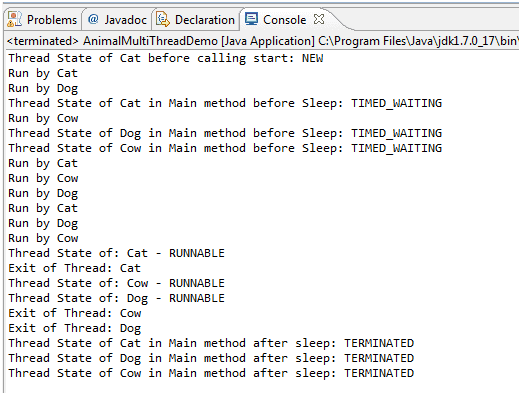
Summary:
- Once a new thread is started, it will always enter the runnable state.
- The thread scheduler can move a thread back and forth between the runnable state and the running state.
- For a typical single-processor machine, only one thread can be running at a time, although many threads may be in the runnable state.
- There is no guarantee that the order in which threads were starteddetermines the order in which they'll run.There's no guarantee that threads will take turns in any fair way. It's upto the thread scheduler, as determined by the particular virtual machine implementation.
- A running thread may enter a blocked/waiting state by a wait(), sleep(),or join() call.
Java Code Editor:
Previous:Java Defining, Instantiating and Starting Thread
Next: Java Thread Interaction
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics