Java Property File Processing
Introduction
Properties is a file extension for files mainly used in Java related technologies to store the configurable parameters of an application. Java Properties files are amazing resources to add information in Java. Generally, these files are used to store static information in key and value pair. Things that you do not want to hard code in your Java code goes into properties files. The advantage of using properties file is we can configure things which are prone to change over a period of time without the need of changing anything in code. Properties file provide flexibility in terms of configuration. Sample properties file is shown below, which has information in key-value pair.
Each parameter is stored as a pair of strings, left side of equal (=) sign is for storing the name of the parameter (called the key), and the other storing the value.
Configuration:
This is property file having my configuration
FileName=Data.txt
Author_Name=Amit Himani
Website=w3resource.com
Topic=Properties file Processing
First Line which starts with # is called comment line. We can add comments in properties which will be ignored by java compiler.
Below is the java program for reading above property file.
Java Code:
package propertiesfile;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.Properties;
public class PropertyFileReading {
public static void main(String[] args) {
Properties prop = new Properties();
try {
// load a properties file for reading
prop.load(new FileInputStream("myConfig.properties"));
// get the properties and print
prop.list(System.out);
//Reading each property value
System.out.println(prop.getProperty("FileName"));
System.out.println(prop.getProperty("Author_Name"));
System.out.println(prop.getProperty("Website"));
System.out.println(prop.getProperty("TOPIC"));
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
The program uses load( ) method to retrieve the list. When the program executes, it first tries to load the list from a file called myConfig.properties. If this file exists, the list is loaded else IO exception is thrown.
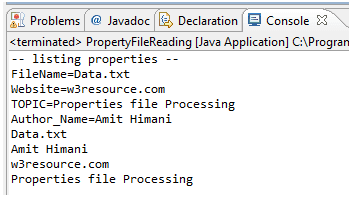
If File is not present in default project root location, we will get an exception like below, We can provide a fully qualified path of the file as well.
Use of getProperties() Method
One useful capability of the Properties class is that you can specify a default property that will be returned if no value is associated with a certain key. For example, a default value can be specified along with the key in the getProperty( ) method—such as getProperty(“name”,“default value”). If the “name” value is not found, then “default value” is returned. When you construct a Properties object, you can pass another instance of Properties to be used as the default properties for the new instance.
Writing Properties file
At any time, you can write a Properties object to a stream or read it back. This makes property lists especially convenient for implementing simple databases. For Example below program writes states can capital cities. “capitals.properties” file having state name as keys and state capital as values.
Java Code:
package propertiesfile;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.Properties;
public class PropertyFileWriting {
public static void main(String args[]) {
Properties prop = new Properties();
try {
// set the properties value
prop.setProperty("Gujarat", "Gandhinagar");
prop.setProperty("Maharashtra", "Mumbai");
prop.setProperty("Madhya_Pradesh", "Indore");
prop.setProperty("Rajasthan", "Jaipur");
prop.setProperty("Punjab", "mkyong");
prop.setProperty("Uttar_Pradesh", "Lucknow");
// save properties to project root folder
prop.store(new FileOutputStream("capitals.properties"), null);
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
After running the program we can see a new file created named “capitals.properties” under project root folder as shown below.
Web Apr 24 19:50:18 IST 2013 Uttar_Pradesh=Lucknow Madhyar_Pradesh=Indore Maharashtra=Mumbai Gujarat=Gandhinagar Panjab=mkyong Rajasthan=jaipur
Summary:
- Properties file provide flexibility in terms of configuration in java based application.
- We can store information in a properties file which can be changed over a period of time like database connection properties, password, input file name or location etc.
- We can Read/Write property file using java.util.Properties library of Java.
Java Code Editor:
Previous: Writing file
Next: Java Serialization
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics